Using String Functions Part 3 Of 3
Python has built-in functions for manipulating strings
Some examples are capitalizing the first characters and counting the occurrence of a specified value.
Care must be taken when manipulating strings to prevent errors such as editing the wrong value and deleting the content of a string by accident.
Python String Functions
String Methods
Name | Description | Example |
---|---|---|
join(iterable) | Concatenation of strings using separator | s = “,”.join(myTuple) |
ljust(width[, fillchar]) | Left justified string using width and optional padding fillchar | l = str1.ljust(20, ” “) |
lower() | Lowercase characters | s = str1.lower() |
lstrip([chars]) | Remove leading characters | s = str1.lstrip(“,.”) |
maketrans(x[, y[, z]]) | Returns a translation table | t = str1.maketrans(“t”, “T”) |
partition(sep) | Split string at first occurrence of sep | p = str1.partition(“about”) |
removeprefix(prefix, /) | Return string[len(prefix):] if string starts with prefix | s = str1.removeprefix(“this”) |
removesuffix(suffix, /) | Return string[:-len(suffix)] if string ends with prefix | s = str1.removesuffix(“Python”) |
replace(old, new[, count]) | Replace all occurrences of word or character | s = str1.replace(“this”, “that”) |
rfind(sub[, start[, end]]) | Search for last occurrence of word or character | s = str1.rfind(“this”) |
rindex(sub[, start[, end]]) | Similar to rfind() but raises ValueError if no match | s = str1.rindex(“this”) |
rjust(width[, fillchar]) | Right justified string using width and optional padding fillchar | r = str1.rjust(20, ” “) |
rpartition(sep) | Split string at last occurrence of sep | p = str1.rpartition(“about”) |
rsplit(sep=None, maxsplit=-1) | Split a string into a list, using specified separator | s = str1.rsplit(“,”) |
rstrip([chars]) | Remove any white spaces at the end of the string | s = str1.rstip() |
split(sep=None, maxsplit=-1) | Split a string into a list where each word is a list item | s = str1.split(“, “) |
splitlines(keepends=False) | Split a string into a list where each line is a list item | s = str1.splitlines(True) |
startswith(prefix[, start[, end]]) | Check if the string starts with prefix with optional start and end | s = str1.startswith(“this”, 7, 20) |
strip([chars]) | Remove trailing spaces with optional chars | s = str1.strip(“,.”) |
swapcase() | Make the lower case letters upper case and the upper case letters lower case | s = str1.swapcase() |
title() | Return title case version of string | s = str1.title() |
translate(table) | Each character mapped through the given translation table | s = str1.translate(dict1) |
upper() | Converts a string into upper case | s = str1.upper() |
zfill(width) | Fill the string with zeros until it specified characters long | s = str1.zfill(10) |
Name | Description | Example |
# Manipulating Strings Tutorial Part III Inspired By OjamboShop.com/learning-python Course str1 = " this tutorial, part III is about manipulating strings using Python ends with a space " tuple1 = ("John", "Jane") print(" and".join(tuple1)) # Join all items in tuple into a string using specified separator print(str1.ljust(20, " ")) # Left justified string 20 length and optional padding space print(str1.lower()) # Lowercase characters print(str1.lstrip(",.")) # Remove leading characters table1 = str1.maketrans("t", "T") # Generate mapping table print(str1.translate(table1)) # Translated string print(str1.partition("about")) # Search for first specified word and return tuple with 3 elements print(str1.removeprefix("this")) # Return string[len(prefix):] if string starts with prefix otherwise return original print(str1.removesuffix("Python")) # Return string[:-len(suffix)] if string ends with suffix otherwise return original print(str1.replace("this", "This")) # Replace all occurrences of word or character print(str1.rfind("this")) # Find last occurrence of the string "this" print(str1.rindex("this")) # Find last occurrence of the string "this" print(str1.rjust(20, " ")) # Right justified string 20 length and optional padding space print(str1.rpartition("about")) # Search for last specified word and return tuple with 3 elements print(str1.rsplit(", ")) # Return a list of the words in the string, using sep as the delimiter string with optional maxsplit print(str1.rstrip()) # Removes white space at end of string or optional characters print(str1.split(", ")) # Return a list of the words in the string, using sep as the delimiter string with optional maxsplit print(str1.splitlines(True)) # Split string keeping line breaks print(str1.startswith("this", 7, 20)) # Determine if string starts with prefix with optional start and end position. print(str1.strip(",.")) # Remove leading and trailing characters print(str1.swapcase()) # Make all upper case letters lower case and vice versa print(str1.title()) # First letter in each word upper case print(str1.upper()) # Upper case string print(str1.zfill(100)) # Fill the string with zeros until it is 100 characters long.
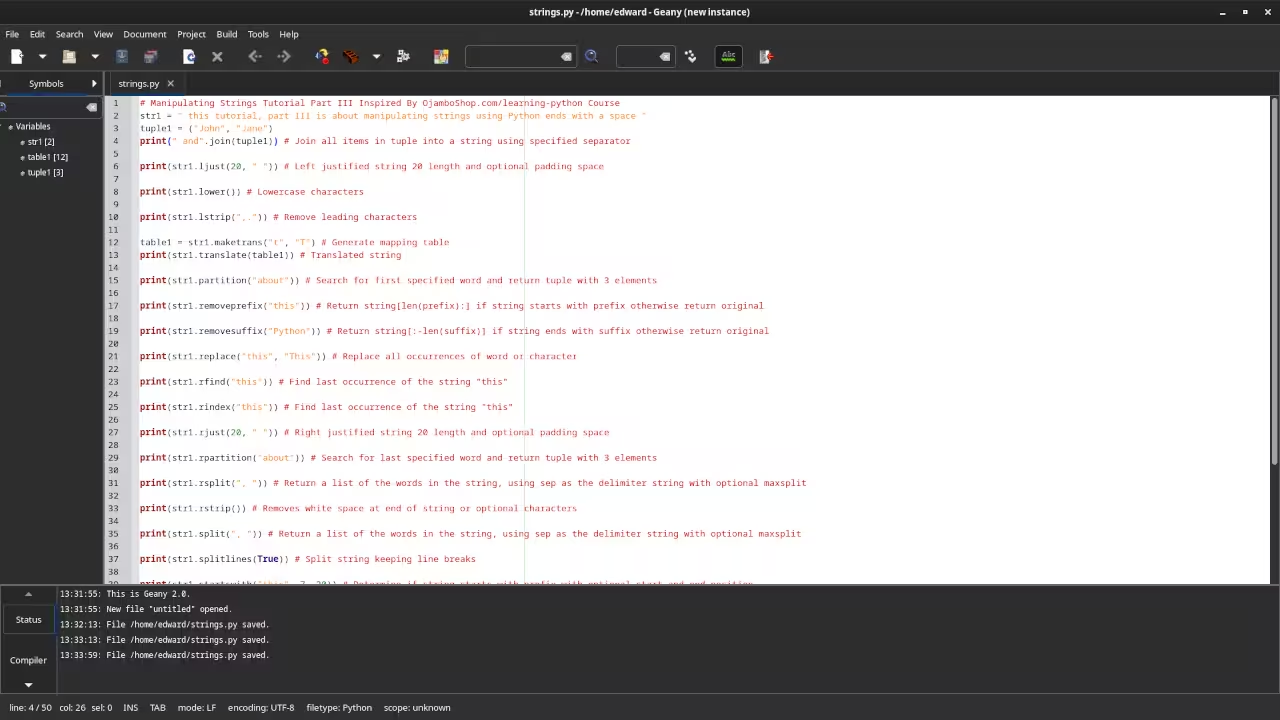
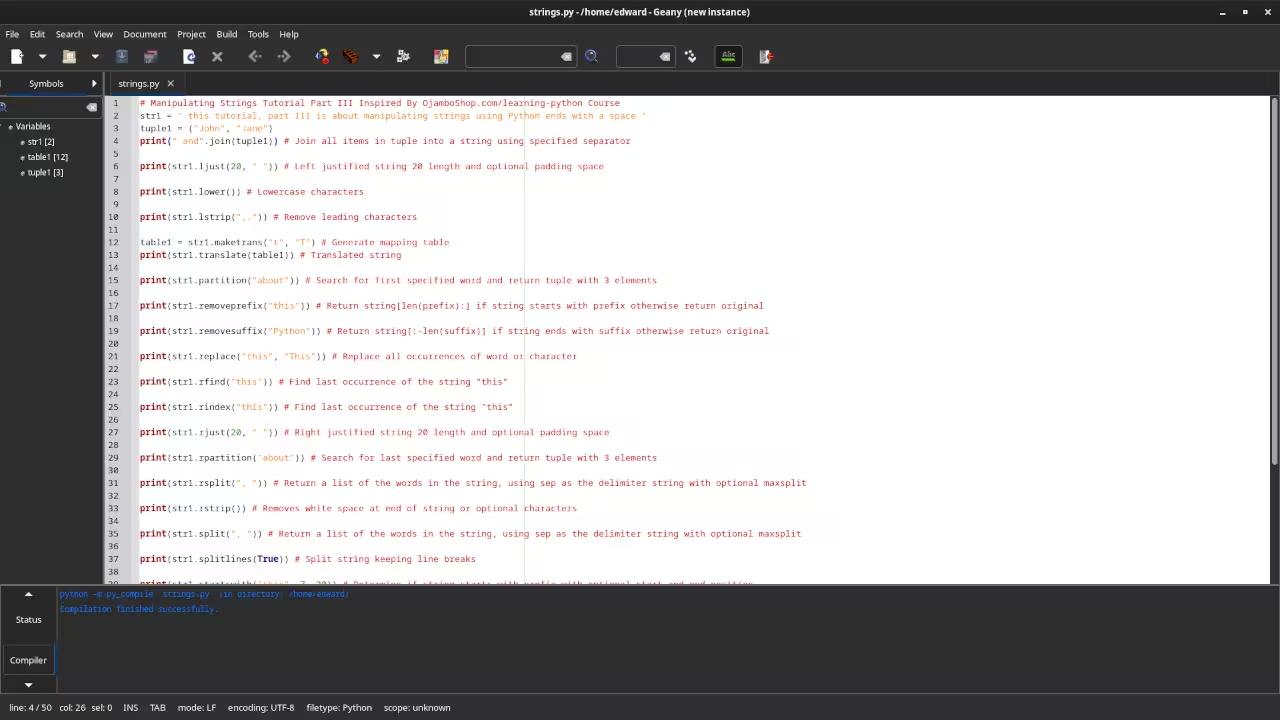
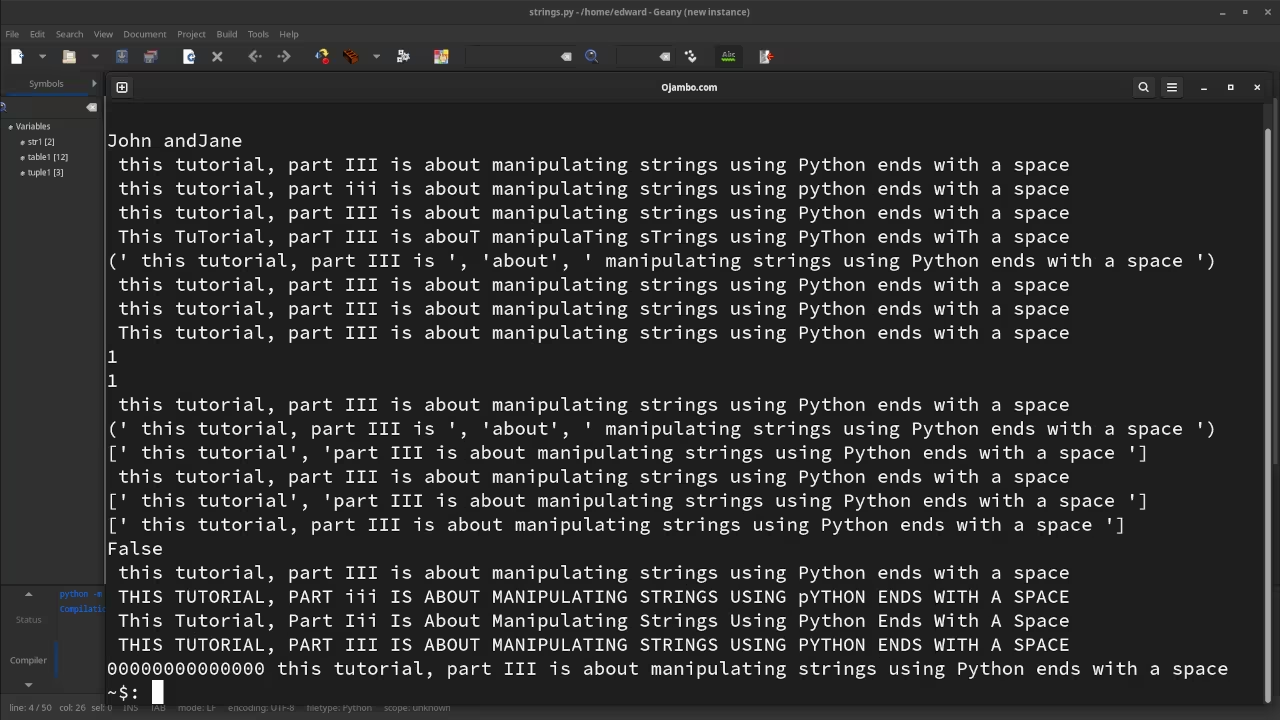
Usage
You can use any IDE, text editor or the Python interactive shell to manipulate strings. In this tutorial part III, strings are manipulated using the built-in Python methods.
Open Source
Python is licensed under the Python Software Foundation License. This allows commercial use, modification, distribution, and allows making private derivatives. The PSF License is compatible with other open source licenses.
Learning Python:
Course is optimized for your web browser on any device.
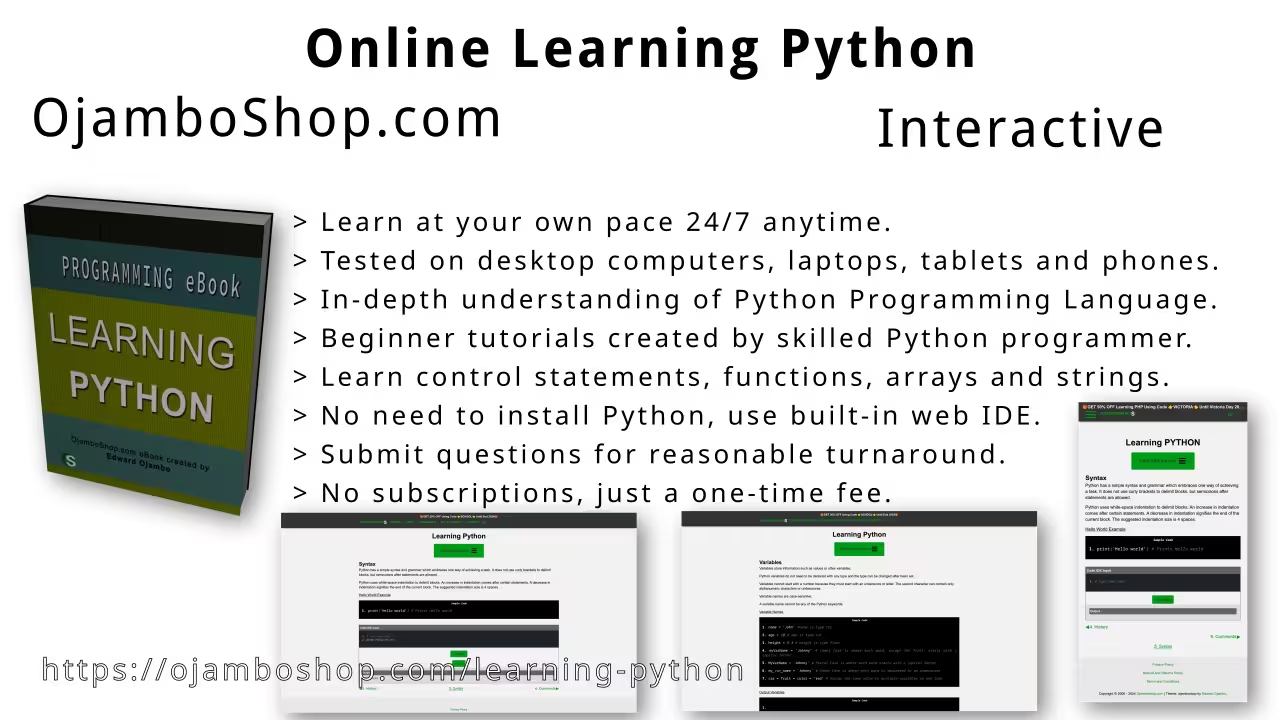
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python until End Day 2024.
Conclusion:
Python makes it easy to manipulate strings using the built-in string methods. Strings can be wrapped in single quotes for literal or double quotes for escape characters.
Take this opportunity to learn Python programming language by making a one-time purchase at Learning Python. A web browser is the only thing needed to learn Python in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- Manipulate Strings Using Python Part I Article
- Manipulate Strings Using Python Part II Article
- Python String Methods
- Python Software Foundation License