Using String Functions Continued
Python has built-in functions for manipulating strings
Some examples are capitalizing the first characters and counting the occurrence of a specified value.
Care must be taken when manipulating strings to prevent errors such as editing the wrong value and deleting the content of a string by accident.
Python String Functions
String Methods
Name | Description | Example |
---|---|---|
find(sub[, start[, end]]) | Searches the string for a specified value and returns the position of where found. | s = str1.find(“i”, 2, 10) |
format(*args, **kwargs) | Formats specified values in a string. | s = “The sum of 1 + 2 is {0}”.format(1+2) |
format_map(mapping) | Formats specified values in a string. | s = ‘{name} is {age} years old’.format_map(person) |
index(sub[, start[, end]]) | Like find(), but raises ValueError when the substring is not found. | s = str1.index(“i”, 2, 10) |
isalnum() | Returns True if all characters in the string are alphanumeric. | b = str1.isalnum() |
isalpha() | Returns True if all characters in the string are in the alphabet. | b = str1.isalpha() |
isascii() | Returns True if all characters in the string are ascii characters. | b = str1.isascii() |
isdecimal() | Returns True if all characters in the string are decimals. | b = str1.isdecimal() |
isdigit() | Returns True if all characters in the string are digits. | b = str1.isdigit() |
isidentifier() | Returns True if the string is an identifier. | b = str1.isidentifier() |
islower() | Returns True if all characters in the string are lower case. | b = str1.islower() |
isnumeric() | Returns True if all characters in the string are numeric. | b = str1.isnumeric() |
isprintable() | Returns True if all characters in the string are printable. | b = str1.isprintable() |
isspace() | Returns True if all characters in the string are whitespaces. | b = str1.isspace() |
istitle() | Returns True if the string follows the rules of a title. | b = str1.istitle() |
isupper() | Returns True if all characters in the string are upper case. | b = str1.isupper() |
Name | Description | Example |
# Manipulating Strings Tutorial Part II Inspired By OjamboShop.com/learning-python Course # str1 = "this tutorial part II is about manipulating strings using Python" print(str1.find("i", 2, 10)) # String search for specified value and optional position print("The sum of 1 + 2 is {0}".format(1+2)) # String format specified values person = {'name': 'John', 'age': 24} print('{name} is {age} years old'.format_map(person)) # String format specified values print(str1.index("i", 2, 10)) # Search for substring print(str1.isalnum()) # Determine if alphanumeric print(str1.isalpha()) # Determine if alphabetic print(str1.isascii()) # Determine if ascii print(str1.isdecimal()) # Determine if decimals print(str1.isdigit()) # Determine if digits print(str1.isidentifier()) # Determine if identifier print(str1.islower()) # Determine if lowercase print(str1.isnumeric()) # Determine if numeric print(str1.isprintable()) # Determine if printable print(str1.isspace()) # Determine if whitespace print(str1.istitle()) # Determine if title print(str1.isupper()) # Determine if uppercase
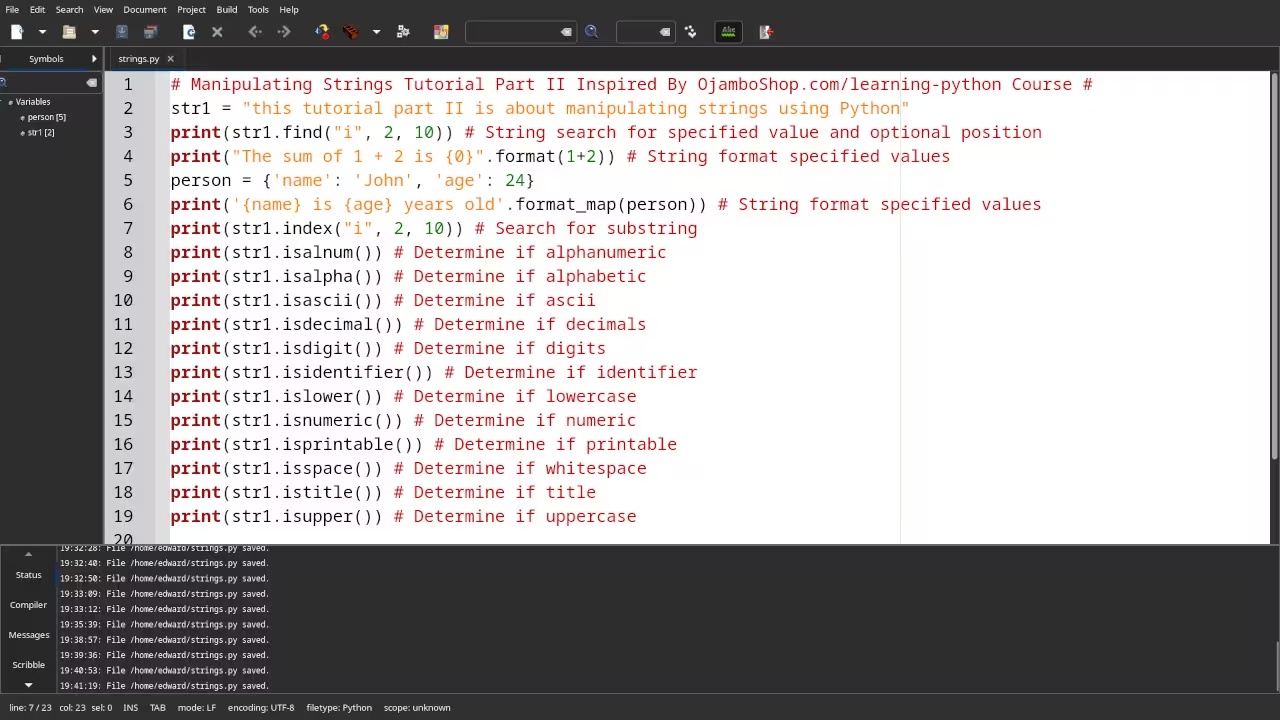
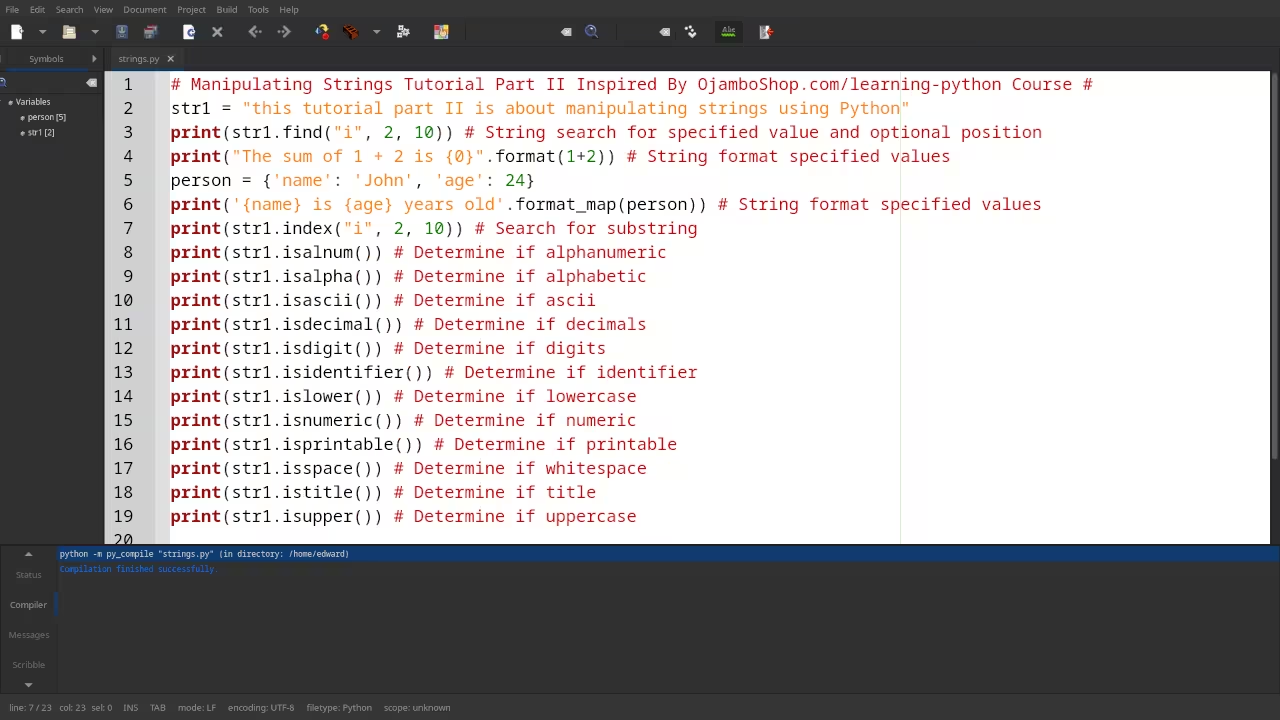
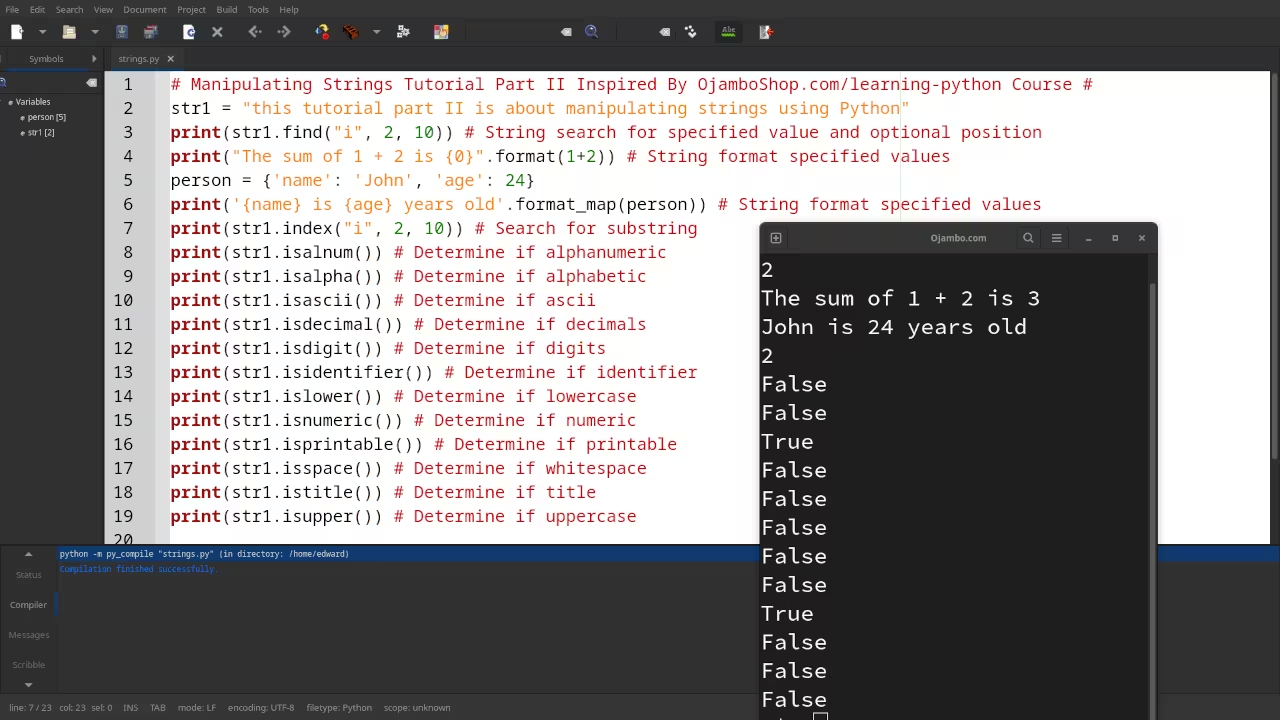
Usage
You can use any IDE, text editor or the Python interactive shell to manipulate strings. In this tutorial part II, strings are manipulated using the built-in Python methods.
Open Source
Python is licensed under the Python Software Foundation License. This allows commercial use, modification, distribution, and allows making private derivatives. The PSF License is compatible with other open source licenses.
Learning Python:
Course is optimized for your web browser on any device.
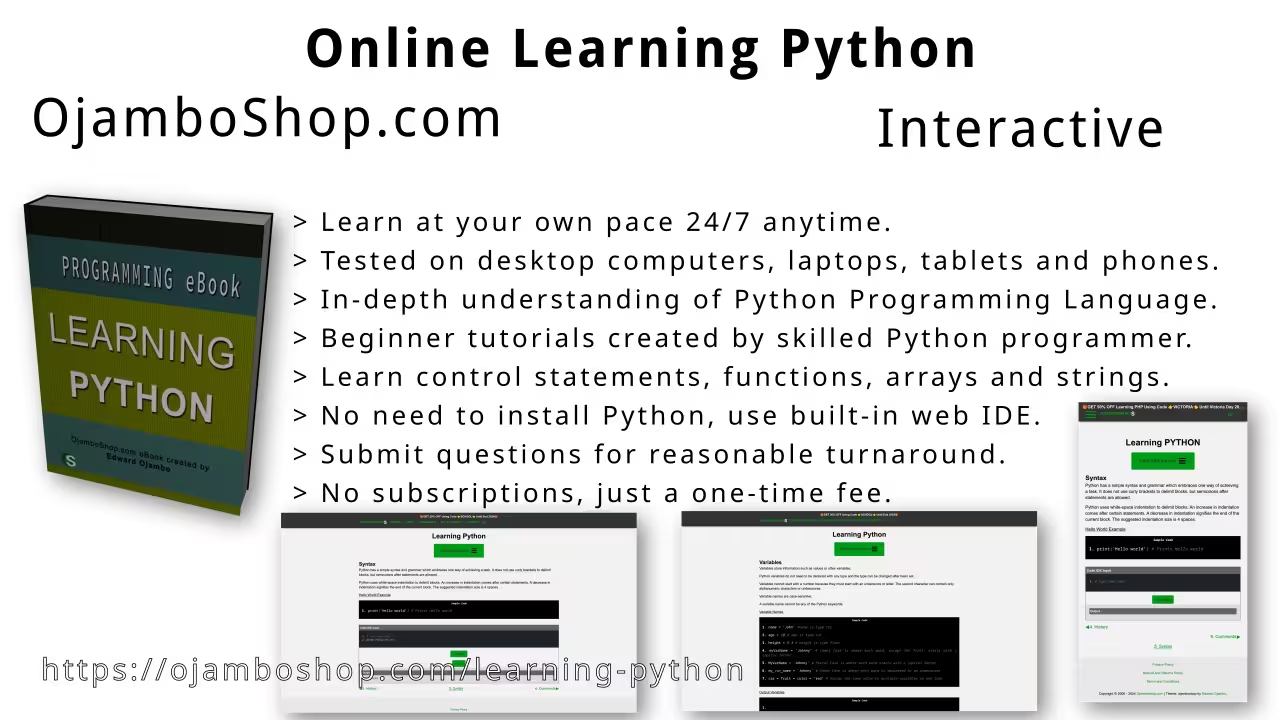
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python until End Day 2024.
Conclusion:
Python makes it easy to manipulate strings using the built-in string methods. Strings can be wrapped in single or double quotes.
Take this opportunity to learn Python programming language by making a one-time purchase at Learning Python. A web browser is the only thing needed to learn Python in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- Python String Methods
- Python Software Foundation License