Using String Functions
Python has built-in functions for manipulating strings
Some examples are capitalizing the first characters and counting the occurrence of a specified value.
Care must be taken when manipulating strings to prevent errors such as editing the wrong value and deleting the content of a string by accident.
Python String Functions
String Methods
Name | Description | Example |
---|---|---|
capitalize() | String is lowercase except capitalized first character. | s = str1.capitalize() |
casefold() | String is converted to lowercase. | s = str1.casefold() |
lower() | String is converted to lowercase. | s = str1.lower() |
center(width[, fillchar]) | String is centered by width and optional padding value | s = str1.center(20, ” “) |
count(sub[, start[, end]]) | Number of times a specified value occurs | c = str1.count(” “) |
encode(encoding=’utf-8′, errors=’strict’) | Encode string specified format in bytes. | s = str1.encode(encoding=”utf-8″, errors=”strict”) |
endswith(suffix[, start[, end]]) | Determine if string ends with specified value. | s = str1.endswith(“.”) |
expandtabs(tabsize=8) | Tab characters replaced by one or more specified spaces. | s = str1.expandtabs(2) |
Name | Description | Example |
# Manipulating Strings Tutorial Inspired By OjamboShop.com/learning-python Course # str1 = "this tutorial is about manipulating strings using Python and includes \t one tab." print(str1.capitalize()) # String is lowercase except capitalized first character print(str1.casefold()) # String is lowercase including non-alpha characters print(str1.lower()) # String is lowercase print(str1.center(20, "-")) # String is centered by width and optional padding value print(str1.count(" ")) # Number of times a specified value occur print(str1.encode(encoding='utf-8', errors='strict')) # Encode string specified format in bytes print(str1.endswith(".")) # Determine if string ends with specified value print(str1.expandtabs(2)) # Tab characters replaced by one or more specified spaces
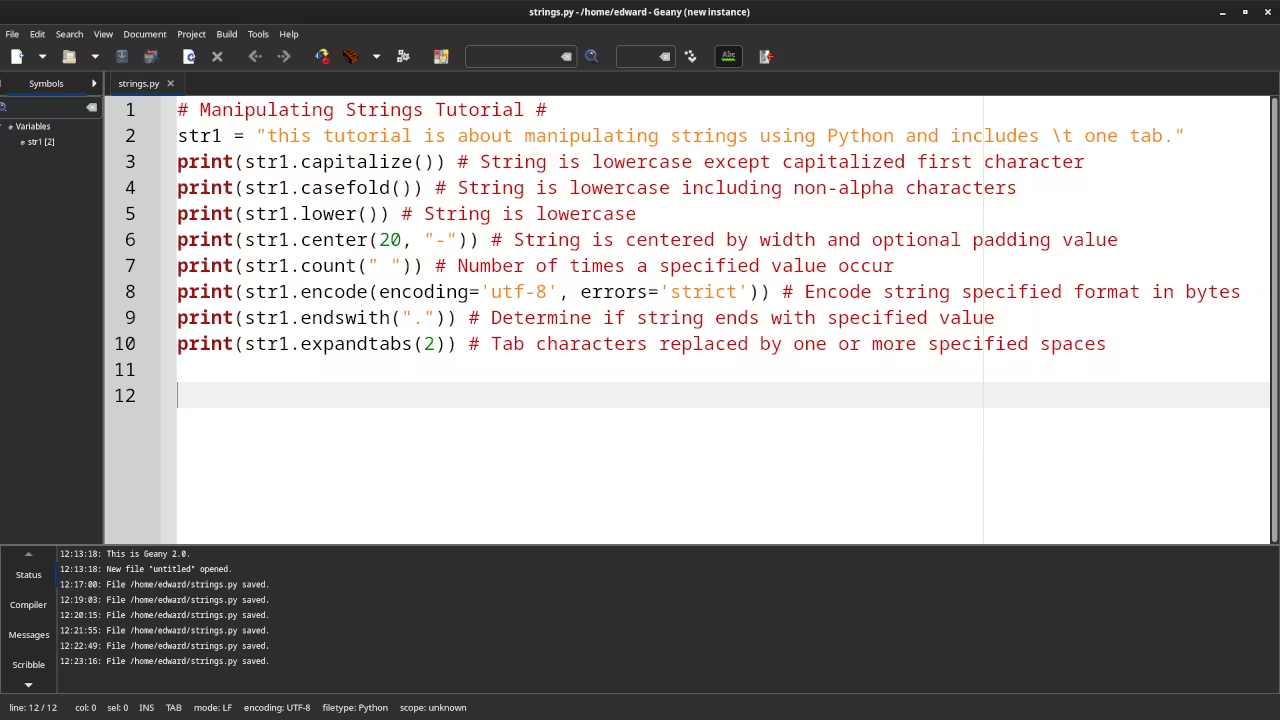
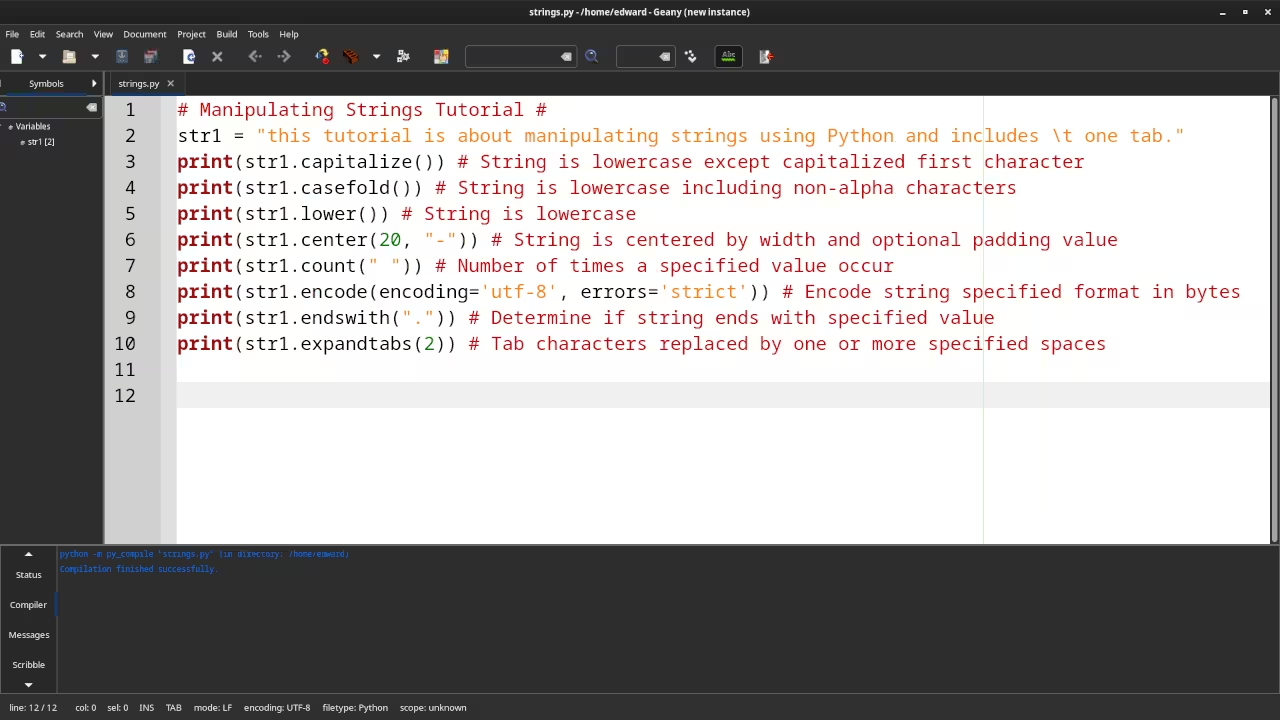
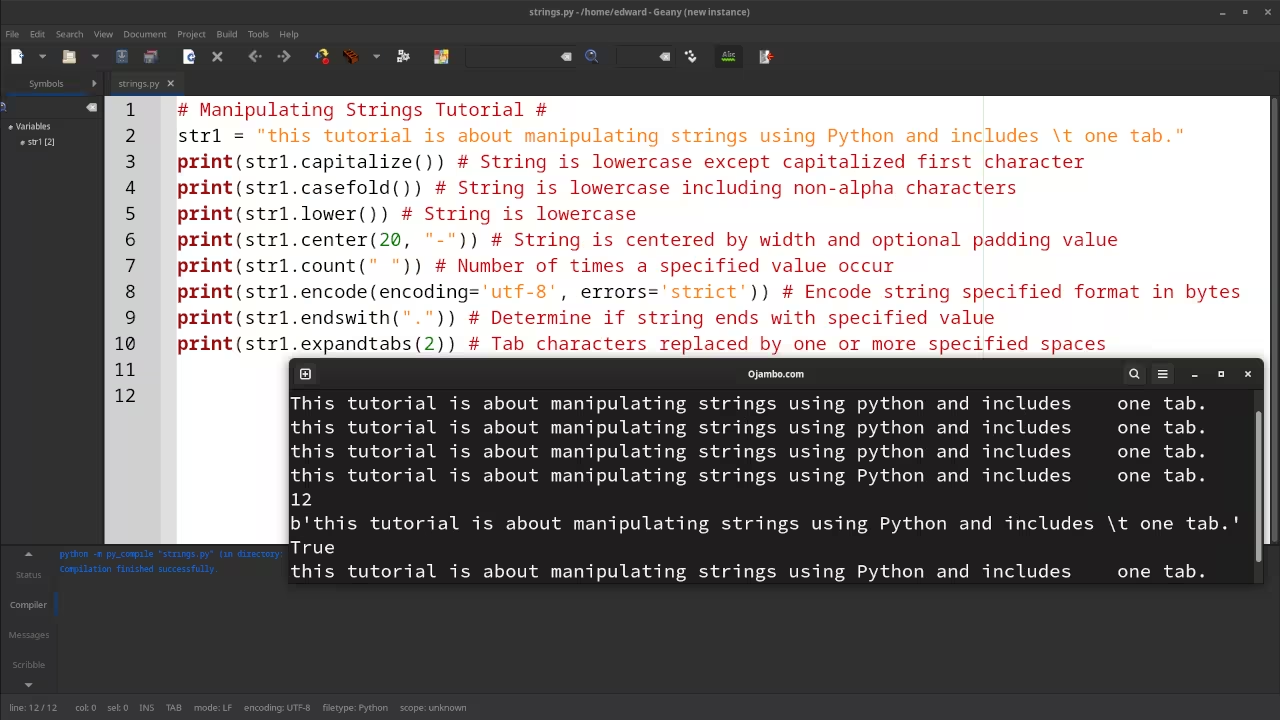
Usage
You can use any IDE, text editor or the Python interactive shell to manipulate strings. In this tutorial, strings are manipulated using the built-in Python methods.
Open Source
Python is licensed under the Python Software Foundation License. This allows commercial use, modification, distribution, and allows making private derivatives. The PSF License is compatible with other open source licenses..
Learning Python:
Course is optimized for your web browser on any device.
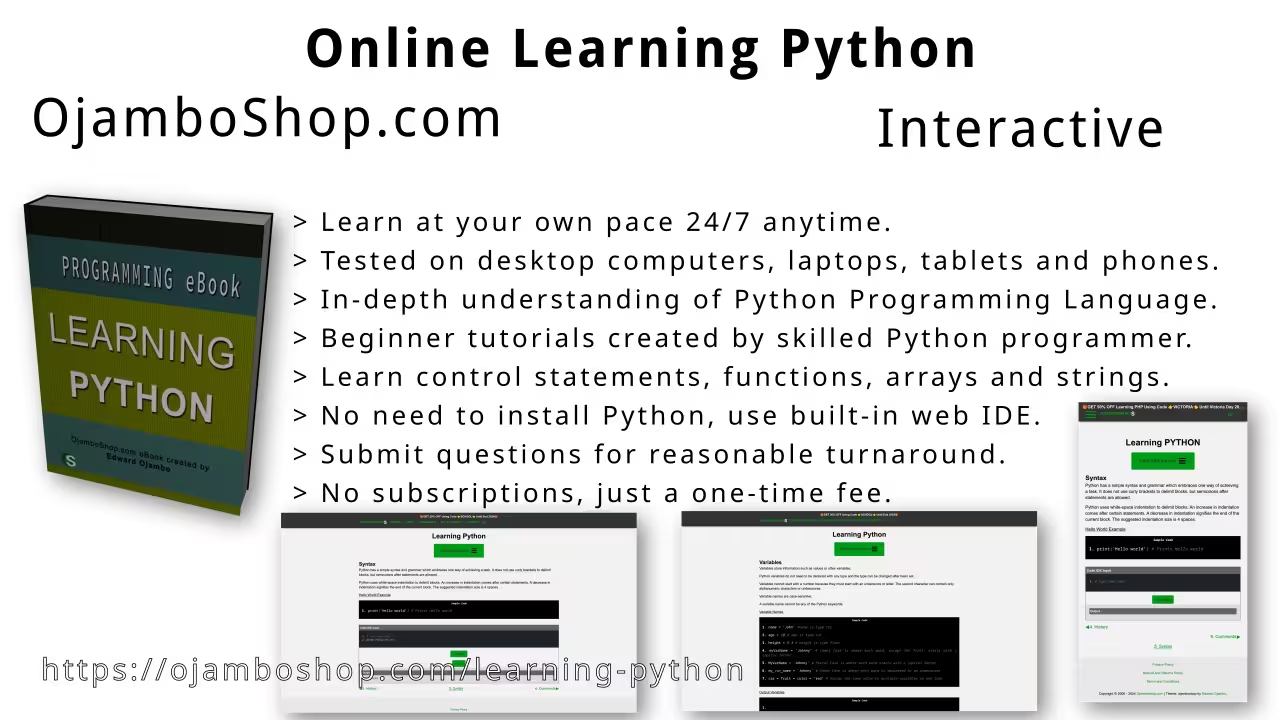
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python until End Day 2024.
Conclusion:
Python makes it easy to manipulate strings using the built-in string methods. Strings can be wrapped in single or double quotes.
Take this opportunity to learn Python programming language by making a one-time purchase at Learning Python. A web browser is the only thing needed to learn Python in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- Python String Methods
- Python Software Foundation License