Parse Text Files
Python has built-in functions for creating, reading, modifying and deleting files.
The Python open() function is used for file handling.
Care must be taken when manipulating files to prevent errors such as editing the wrong file and deleting the content of a file by accident.
Python File Handling
Open Function
Name | Description | Example |
---|---|---|
r | Opens a file for reading. | f = open(“myfile.txt”, “r”) |
a | Opens a file for appending | f = open(“myfile.txt”, “a”) |
w | Opens a file for writing | f = open(“myfile.txt”, “w”) |
x | Create a specified file. | f = open(“myfile.txt”, “x”) |
t | Text mode | f = open(“myfile.txt”, “rt”) |
b | Binary mode | f = open(“myimage.avif”, “rb”) |
import json # Open JSON File with open("myjson.json") as json_file: # Parse JSON File pythonobj = json.load(json_file) # Read Values By Key name = pythonobj['name'] age = pythonobj['age'] graduate = pythonobj['graduate'] combined = f"My name is {name}, and I am {age} years old and it is {graduate} that I am a graduate." print(combined)
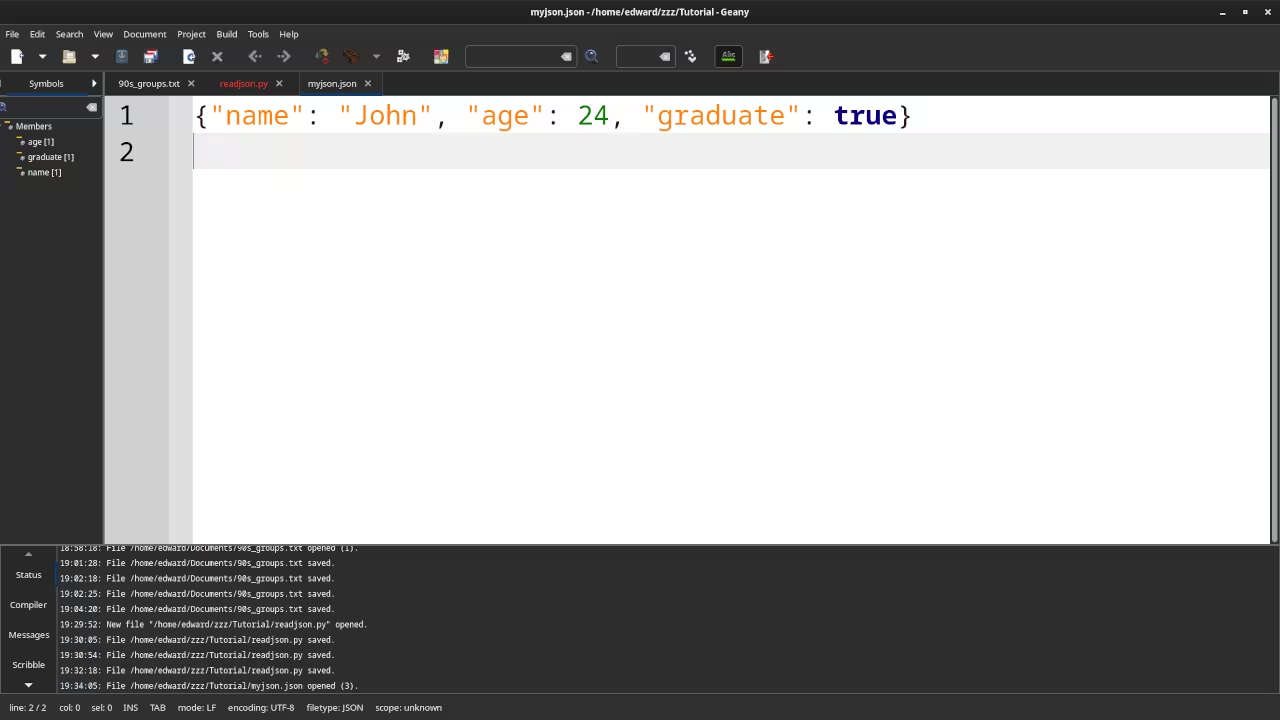
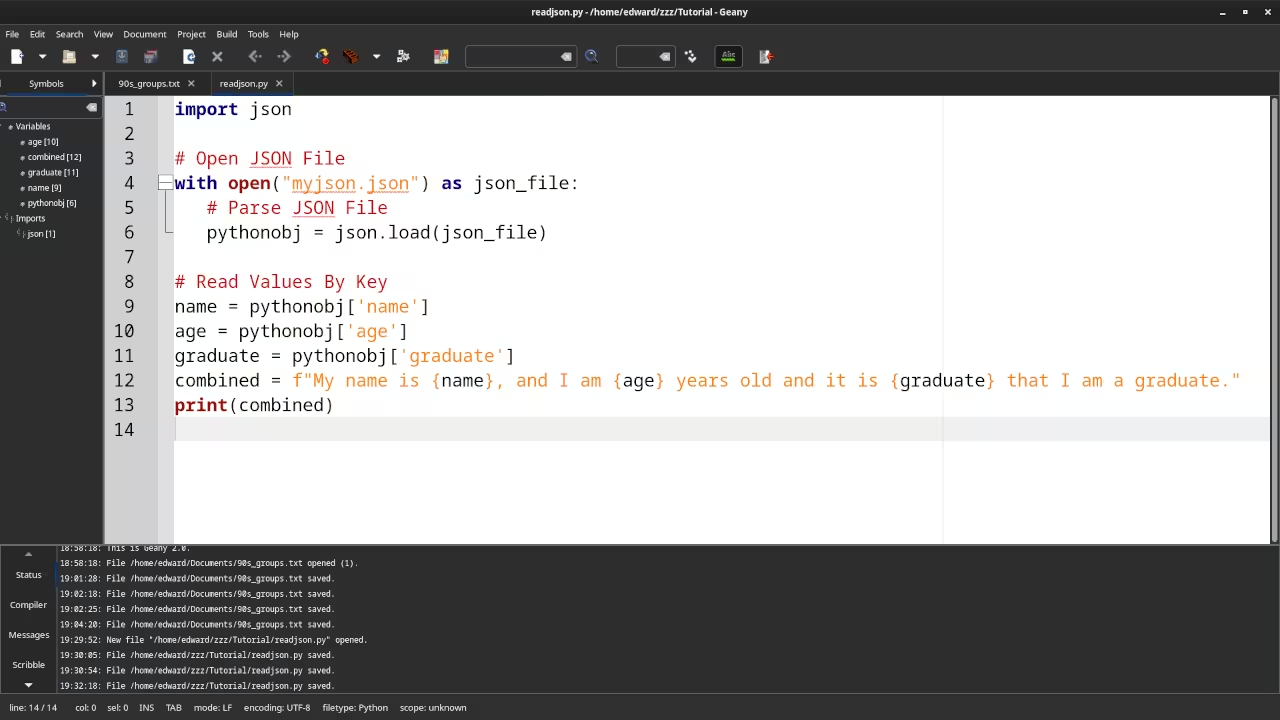
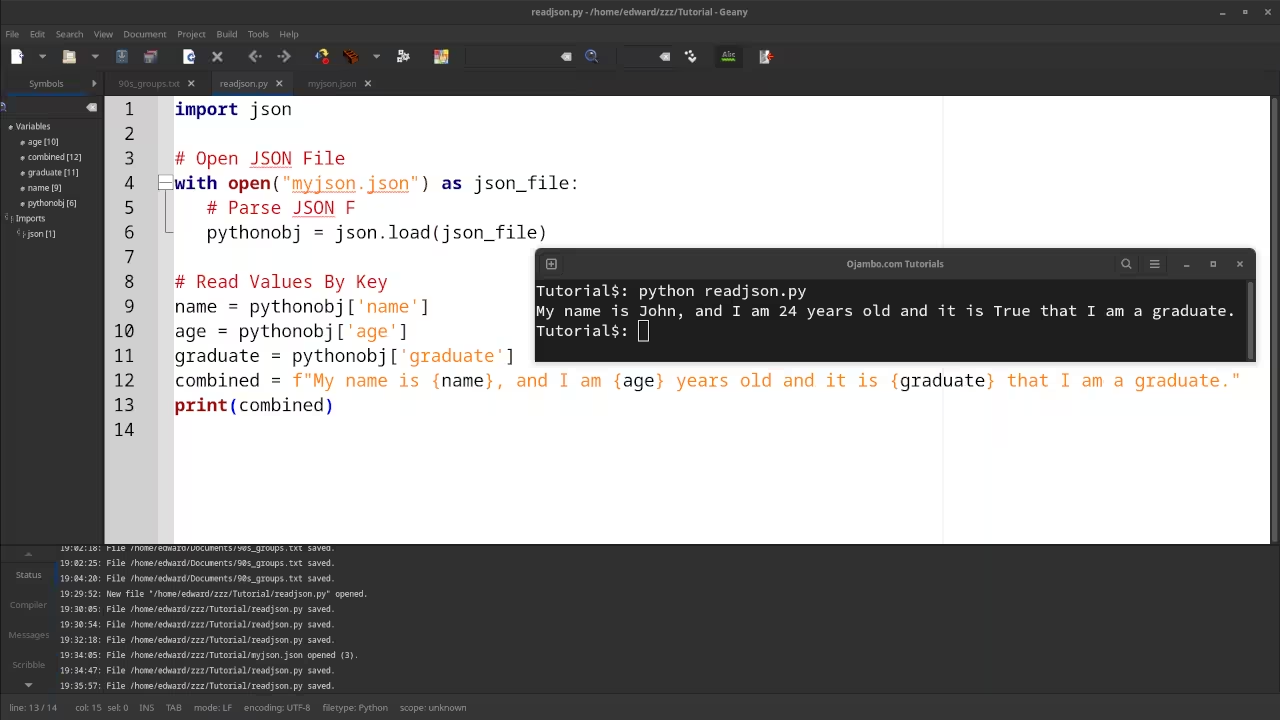
Usage
You can use any IDE, text editor or the Python interactive shell to parse text files. In this tutorial, a JSON string in a text file was used, but the code can easily be expanded to read the contents of a other text files and parse.
Morality Source
JSON is licensed under the JSON License. This allows commercial use, modification, distribution, and allows making derivatives proprietary. The clause “The Software shall be used for Good, not Evil” is incompatible with other open source licenses.
Learning Python:
Course is optimized for your web browser on any device.
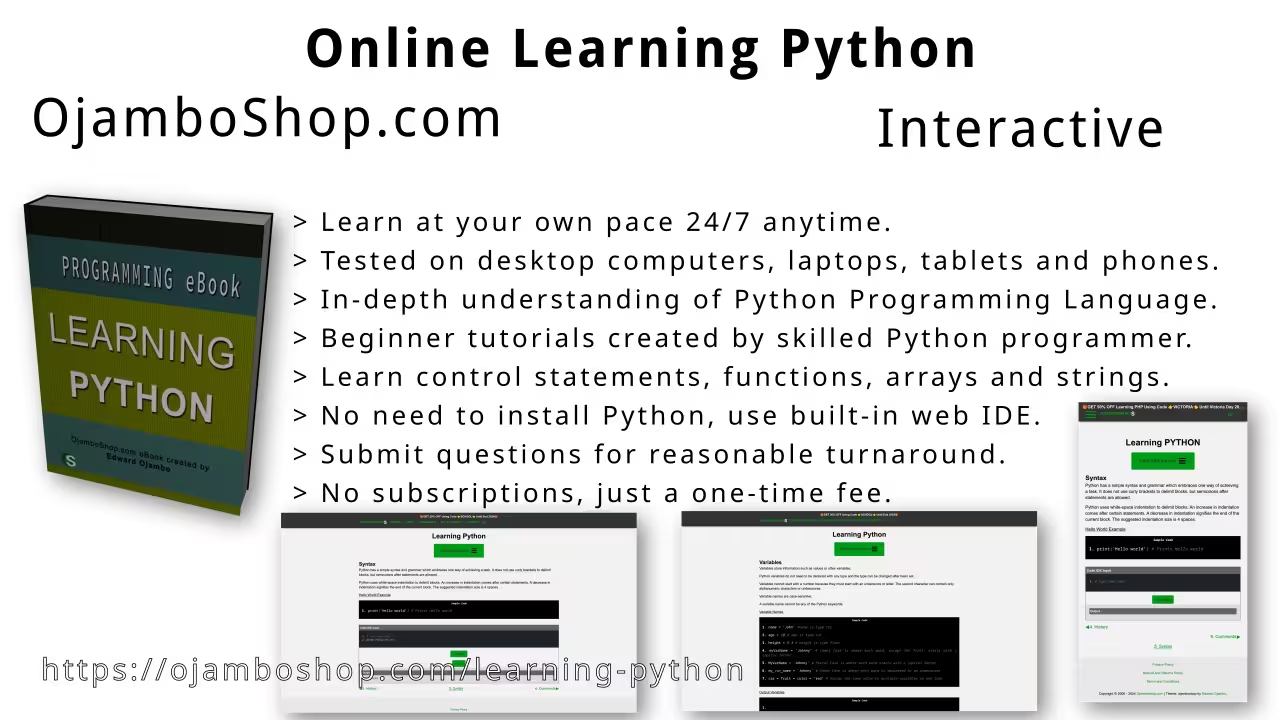
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python until End Day 2024.
Conclusion:
Python makes it easy to parse JSON data after importing the JSON module. JSON can be parsed as a string or from an external file.
Take this opportunity to learn Python programming language by making a one-time purchase at Learning Python. A web browser is the only thing needed to learn Python in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- JSON Data Interchange Standard
- JSON License