Implement Array Lists In Python
An array specifies a variable that can be indexed as a list in rows and columns.
The first index is zero as is common is most programming languages. Python does not have an array data type. Instead the data type list, tuple, set or dictionary can be used.
A list is a collection which is ordered and changeable allowing duplicate members. Lists are created using square brackets..
Common Functions For Manipulating Lists In Python
Common List Functions
Name | Description | Example |
---|---|---|
len() | Determine how many items | len(even_numbers) |
append() | Append items | even_numbers.append(12) |
insert() | Insert item at specified index | even_numbers.insert(1, 2) |
remove() | Remove specified item | even_numbers.remove(2) |
pop() | Pop the item at specified index | even_numbers.pop(1) |
del | Delete the item at specified index | del even_numbers[1] |
clear() | Remove all values | even_numbers.clear() |
sort() | Sort in ascending order | even_numbers.sort() |
reverse() | Sort in reverse order | even_numbers.reverse() |
copy() | Make a copy | even_numbers.sort() |
extend() | Add elements from one list | list1.extend(list2) |
count() | Returns number of elements with specified value | list1.count(2) |
index() | Returns index of first element with specified value | list1.count(4) |
Name | Description | Example |
Python List Snippet
# OjamboShop.com Learning Python Array List Tutorial # even_numbers = [2,4,6,8,10] print(even_numbers) print(len(even_numbers)) # Number Of Items In List duplicate_list = [2,4,6,8,10,2,4,6,8,10] print(duplicate_list) print(duplicate_list[1]) # Access List Item By Index Number print(duplicate_list[2:5]) # Range Of Indexes even_numbers[4] = 12 # Change Item Value By Index Number print(even_numbers) even_numbers.append(14) # Add Item To End print(even_numbers) even_numbers.insert(1, 2) # Insert Item Value At Index Number print(even_numbers) even_numbers.remove(2) # Remove Item Value print(even_numbers) even_numbers.pop(1) # Pop Item At Index Number print(even_numbers) del even_numbers[1] # Remove Item At Index Number print(even_numbers) even_numbers.clear() # Remove All Items print(even_numbers) even_numbers.sort() # Sort All Items In Ascending Order print(even_numbers) even_numbers.reverse() # Sort All Items In Reverse Order print(even_numbers) copied_list = even_numbers.copy() # Make A Copy print(copied_list) list1 = ["John", "Jake"] list2 = even_numbers.copy() list3 = list1 + list2 # Join Two Lists list1.extend(list2) # Add Elements From One List print(list1) print(list3) print(list1.count("John")) # Returns Number Of Times The Value "John" Appears print(list1.index("John")) # Returns Index Of First Time The Value "John" Appears
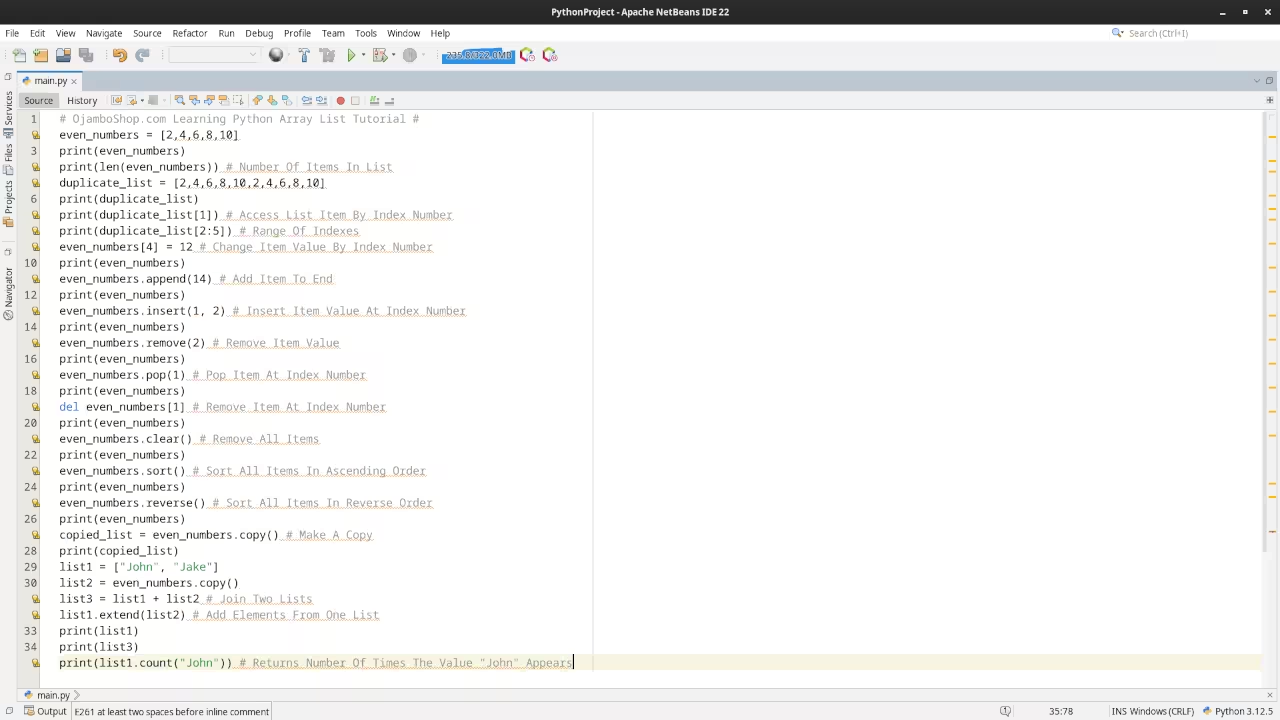
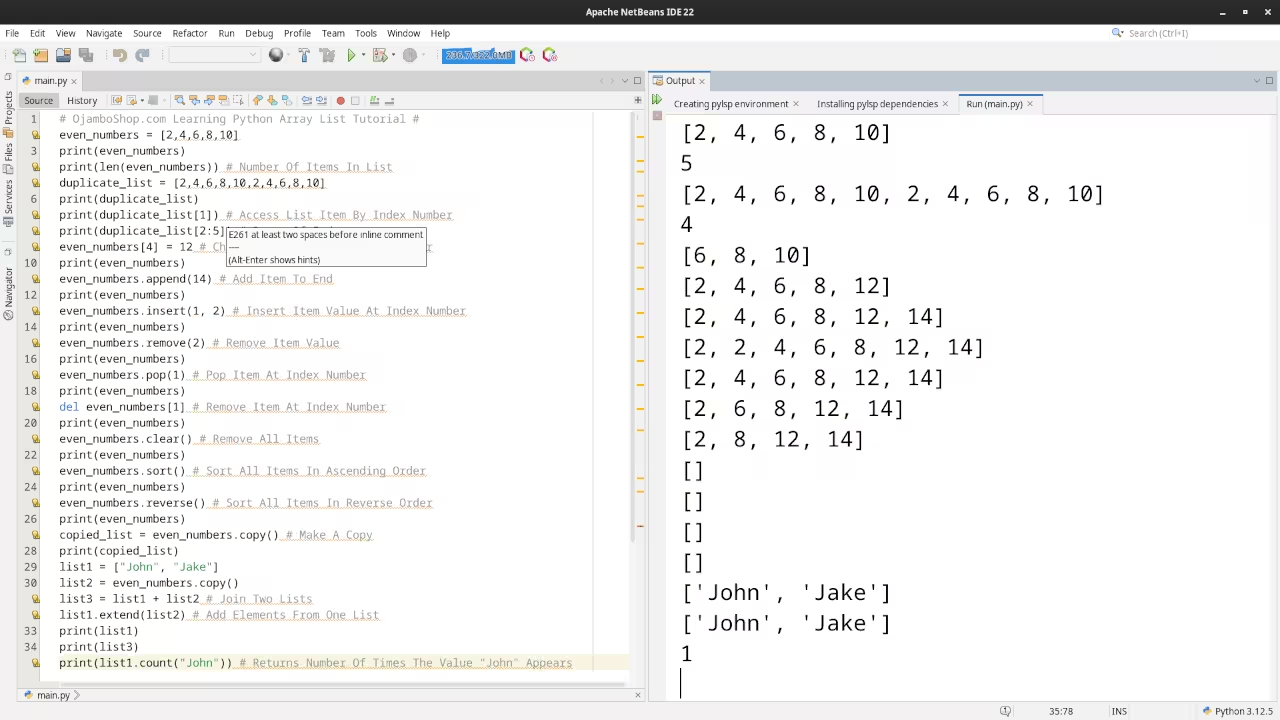
Usage
You can use any IDE or text editor and the command line to compile and execute Python code. For this tutorial, the OjamboShop.com Learning Python Course Web IDE can used to input and compile Python code for lists.
Open Source
Python is licensed under the Python Software Foundation License. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
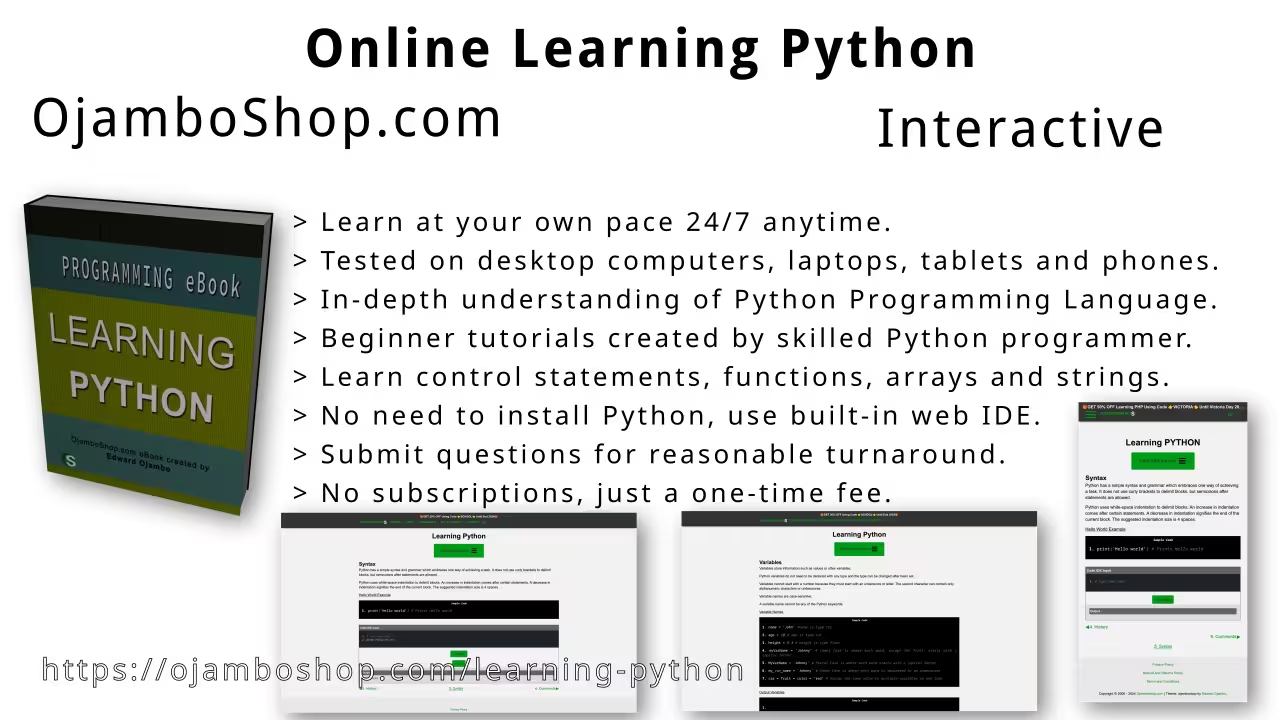
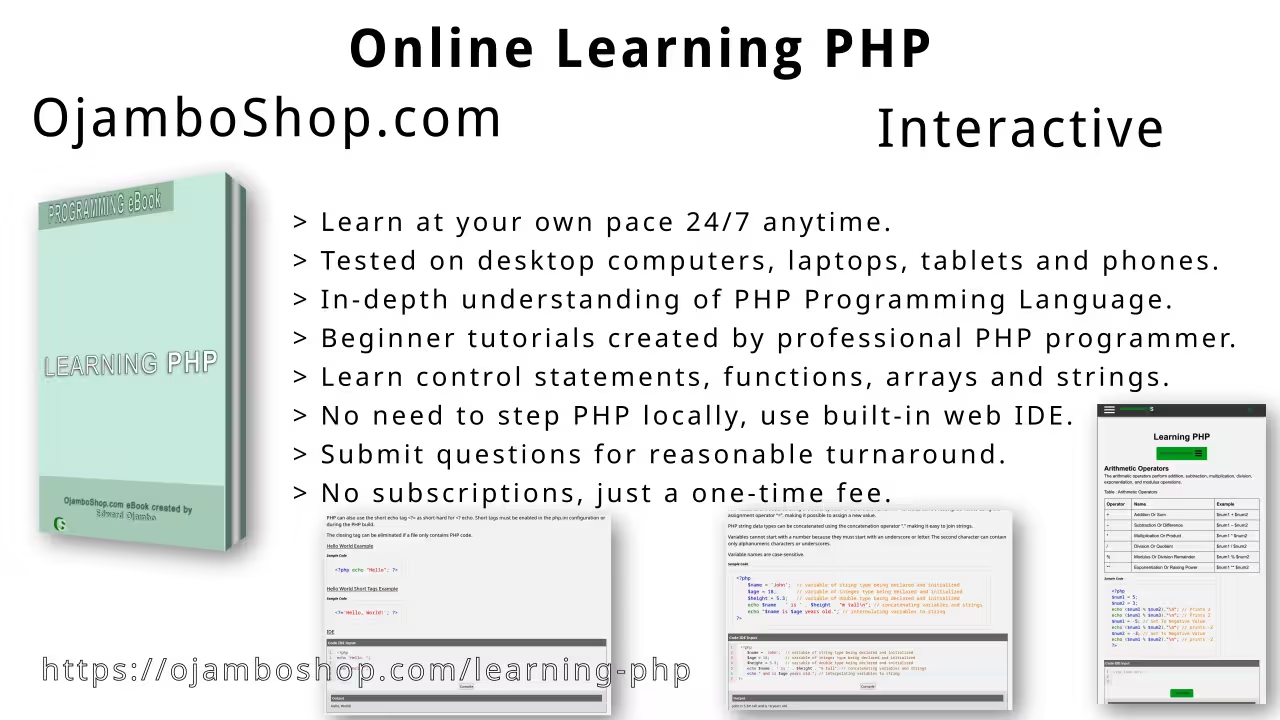
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python Course or for Learning PHP Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
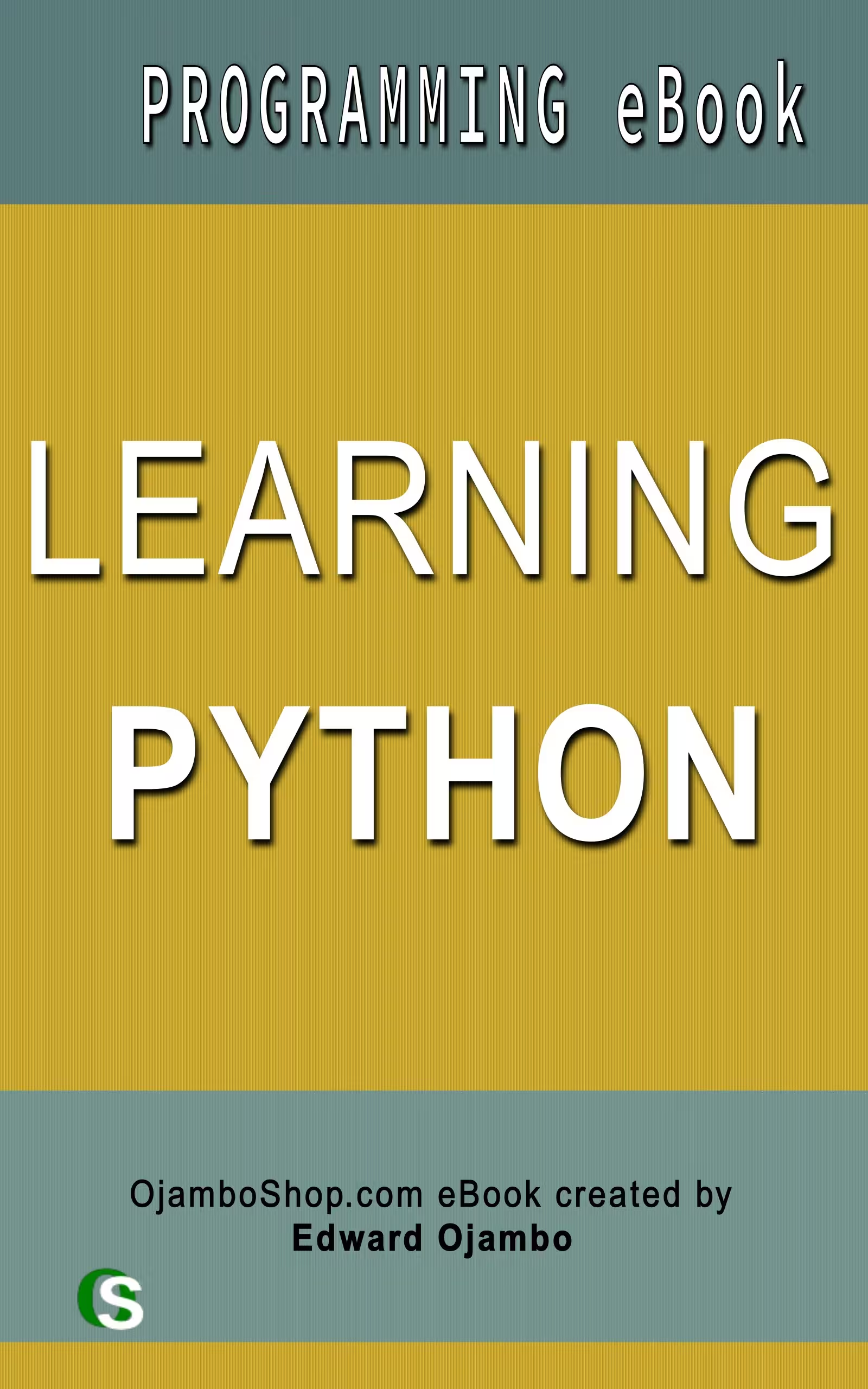
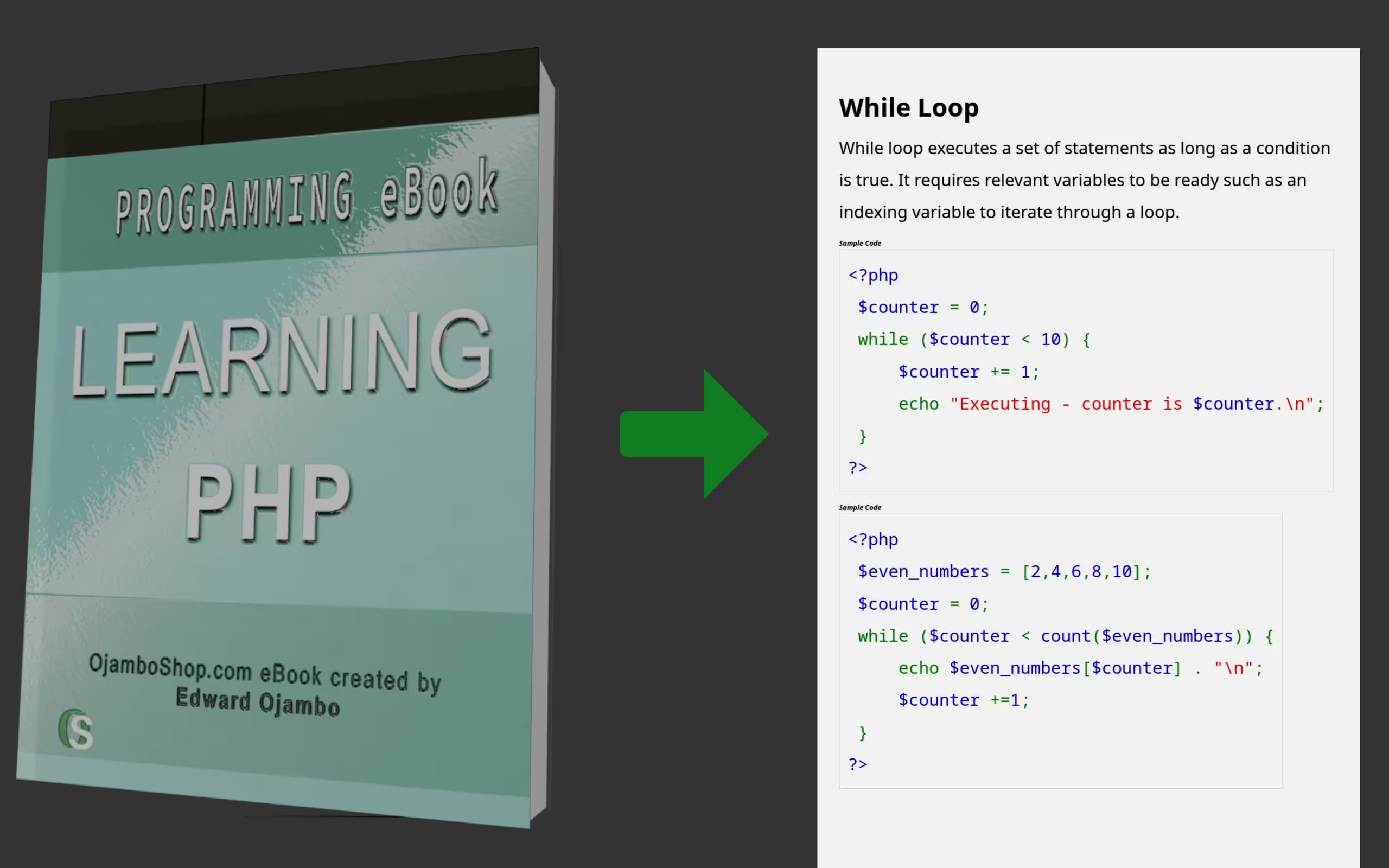
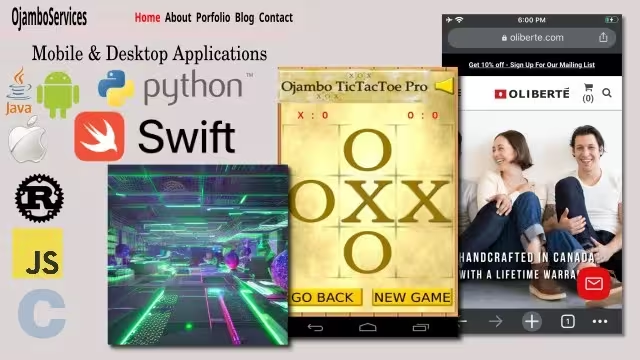
Conclusion:
Python makes it easy to use lists. Use lists when you need an ordered, changeable array that might have duplicate members.
Take this opportunity to learn the Python or PHP programming language by making a one-time purchase at Learning Python Course or Learning PHP Course. A web browser is the only thing needed to learn Python or PHP in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning Python Ebook or Learning PHP Ebook
For custom websites, app development and one-on-one tutorials, go to OjamboServices.com.
References:
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials