Create Nested Functions In JavaScript
In JavaScript, a function declaration defines a function with the specified parameters and code block.
Functions can be called before their creation due to hoisting. A JavaScript function is a subprogram that can be called by code externally or internally to the function. Nested functions are defined within other functions.
Nested functions have access to the variables of their parent function.
Common Syntax Of Nested Functions In JavaScript
Glossary:
Hoisting
The interpreter moves function declaration to the top of their scope.
Scope
The accessibility of variables and functions within certain parts of your code.
Declaration
Used to define variables, functions, and constants.
Method
Function defined within a object.
Arrow Functions
Syntax for creating anonymous expressions.
Closures
Access variables from outer scope even after outer function has finished.
Common Nested Functions
Name | Description | Example |
---|---|---|
function one() {function two() {}} | Nested Function. | function one() {function two() {}} |
myFunc() | Closure | let m = myFunc(); |
Name | Description | Example |
JavaScript Nested Functions Snippet
// OjamboShop.com Learning JavaScript Nested Functions Tutorial // Nested Function function outFunc() { let name = 'John'; function inFunc() { console.log('The name is ' +name); } inFunc(); } outFunc(); // Closure function showName() { let name = 'Jane'; function displayName() { console.log(name); } return displayName; } let myFunc = showName(); myFunc(); // Closure Arguments function addTwo(num1) { return function(num2) { return num1+num2; } } let myClosure = addTwo(5); console.log(myClosure(3)); // IIFE Arrow Function Closure let calculateSum = (function(num1, num2) { let sum = 0; return function() { sum = num1+num2; return sum; } })(5,3); calculateSum();
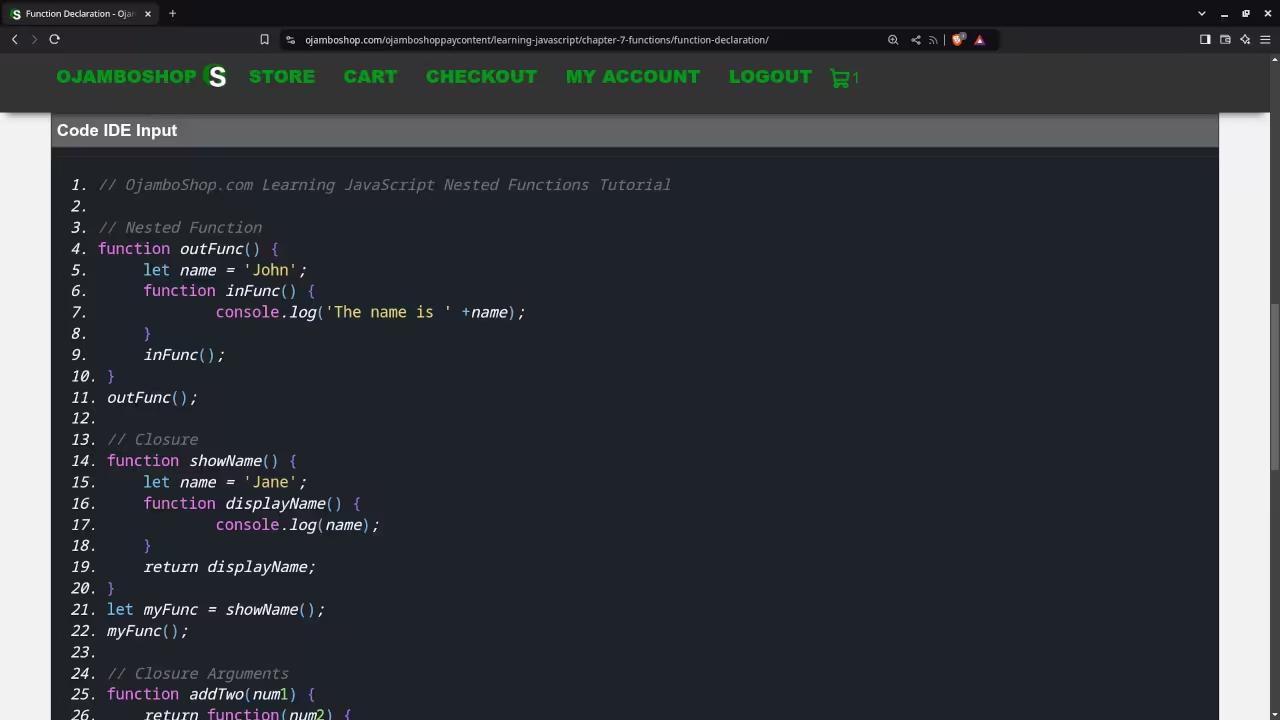
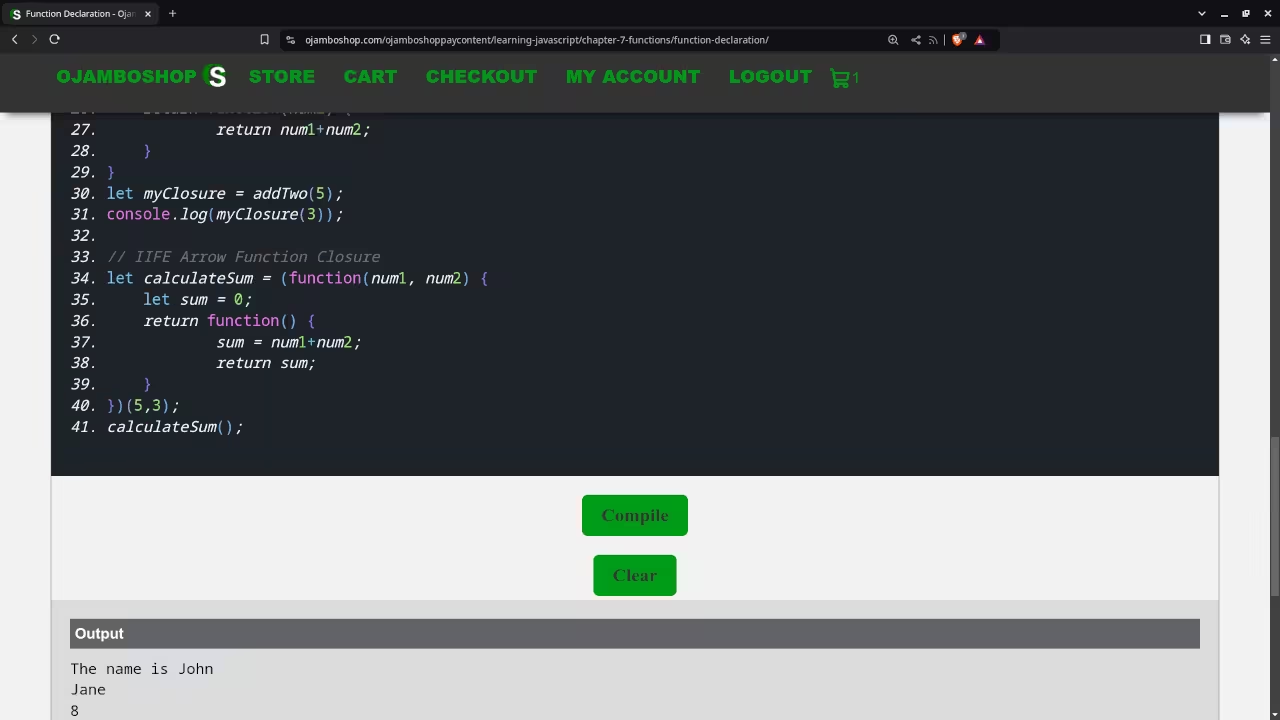
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the nested functions.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
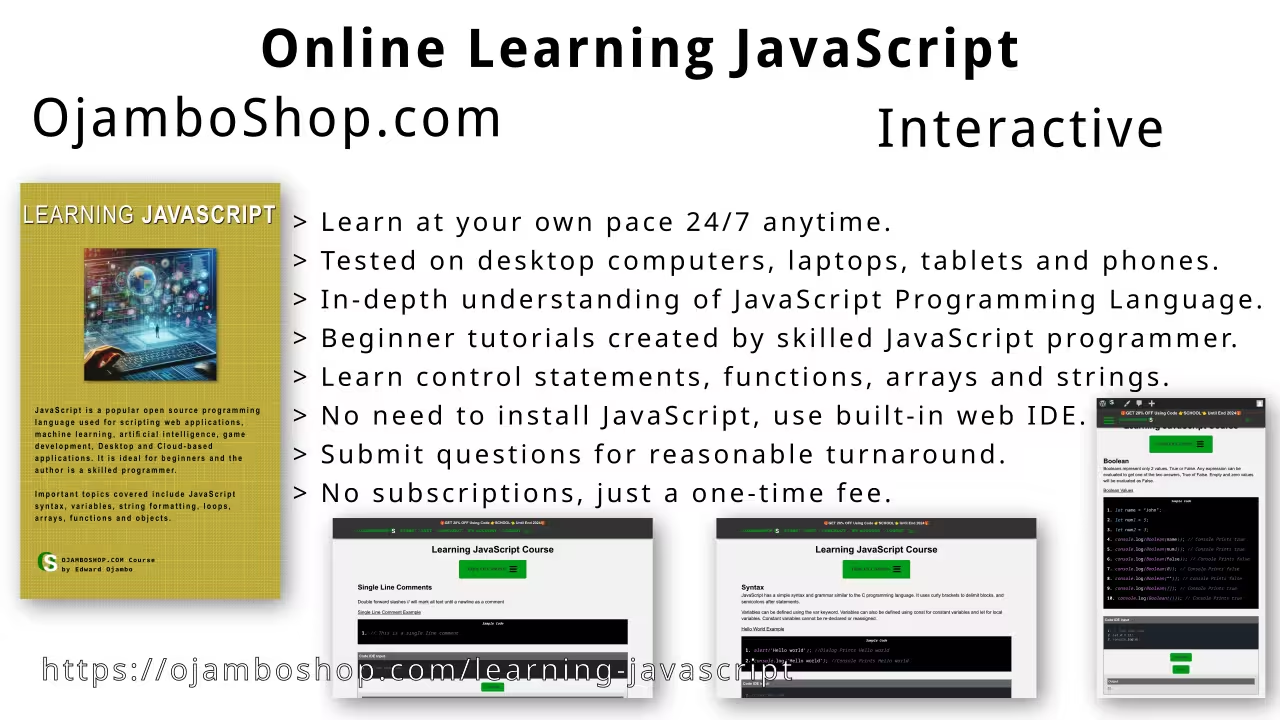
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
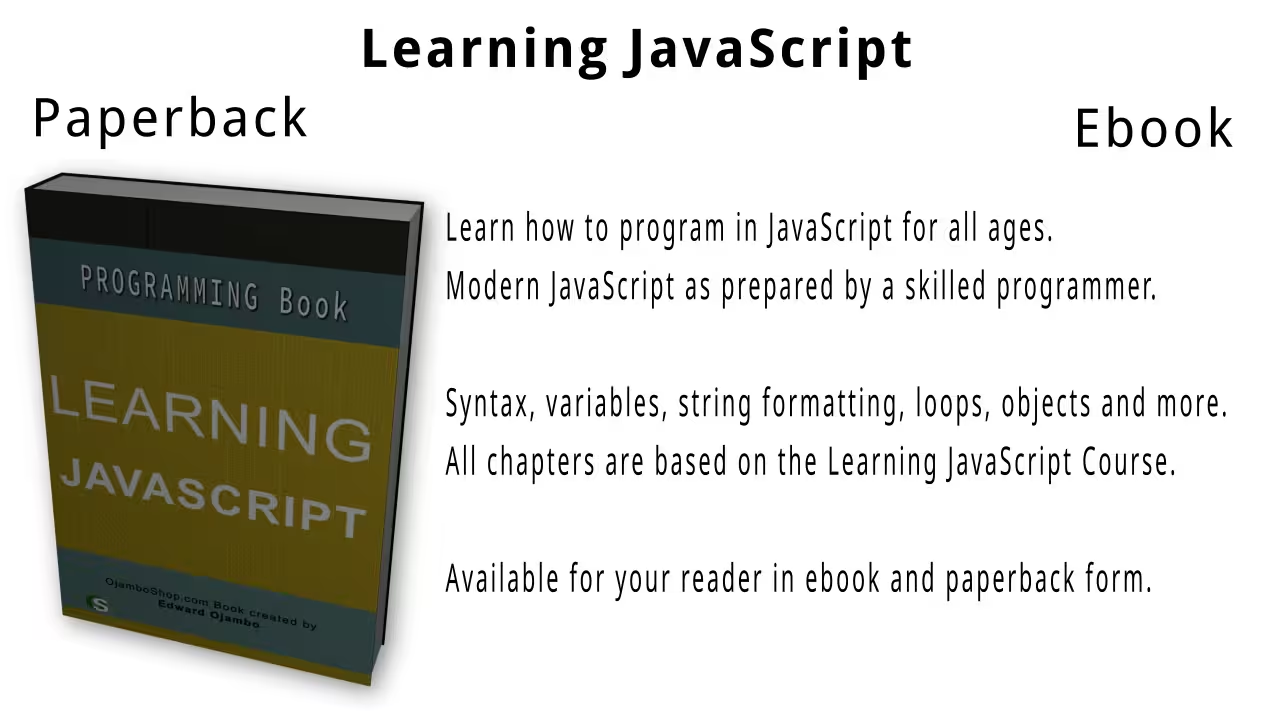
Conclusion:
JavaScript makes it easy to use create nested functions. Define functions within other functions in order to access variables of their parent function.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials