Create Map Methods In JavaScript
A Map holds key-value pairs and the keys can be of any data type.
The map maintains the insertion order of keys and provides a size property. Common methods for working with maps include set(), get(), delete() and clear().
Map Methods can be iterated directly and have built-in methods for managing key-value pairs. Map Methods unlike JavaScript Objects, do not have default keys.
Common Syntax Of Map Methods In JavaScript
Glossary:
Iterable
Object values can be looped in a “for of” loop.
Default
Define default values in none provided.
Declaration
Used to define variables, functions, and constants.
Method
Function defined within a object.
Constructor
Special method within a class used to initialize and create objects of that class.
Common Methods For Map Methods
Name | Description | Example |
---|---|---|
Map() | Create a new Map. | new Map(); |
set() | Add elements to a Map. | set(“age”, 24); |
get() | Get value of a key in a Map. | get(“age”); |
size | Return number of elements in a map. | prices.size; |
delete() | Removes an element in a map. | prices.delete(“Bread”); |
has() | Returns true if specified key exists in a map. | prices.has(‘Bread’); |
clear() | Removes all elements in a map. | prices.clear(); |
Name | Description | Example |
JavaScript Map Methods Snippet
// OjamboShop.com Learning JavaScript Map Methods Tutorial // Create Map Via Passing Array let prices = new Map([ ["Milk", 10], ["Butter", 5], ["Bread", 2], ]); console.log(typeof prices); console.log(JSON.stringify(Object.fromEntries(prices))); // Create Map Elements Via Set let costs = new Map(); costs.set("Juice", 3); costs.set("Cereal", 4); costs.set("Soap", 6); console.log(typeof costs); console.log(JSON.stringify(Object.fromEntries(costs))); // Retrieve Values console.log("Milk price: " + prices.get("Milk")); console.log("Butter price: " + prices.get("Butter")); console.log("Bread price: " + prices.get("Bread")); console.log("Juice cost " + costs.get("Juice")); console.log("Cereal cost " + costs.get("Cereal")); console.log("Soap cost " + costs.get("Soap")); // List All Elements In Map prices.forEach (function(value, key) { console.log(`${key} price: ${value}`); }); costs.forEach (function(value, key) { console.log(`${key} cost: ${value}`); }); // Number Of Elements console.log("The number of elements for prices is " + prices.size); console.log("The number of elements for costs is " + costs.size); // Boolean Check console.log("Milk check is " + prices.has("Milk")); console.log("Juice check is " + costs.has("Juice")); // Remove Some Elements prices.delete("Milk"); costs.delete("Juice"); console.log("Milk check is " + prices.has("Milk")); console.log("Juice check is " + costs.has("Juice")); // Remove All Elements prices.clear(); costs.clear(); console.log("The number of elements for prices is " + prices.size); console.log("The number of elements for costs is " + costs.size);
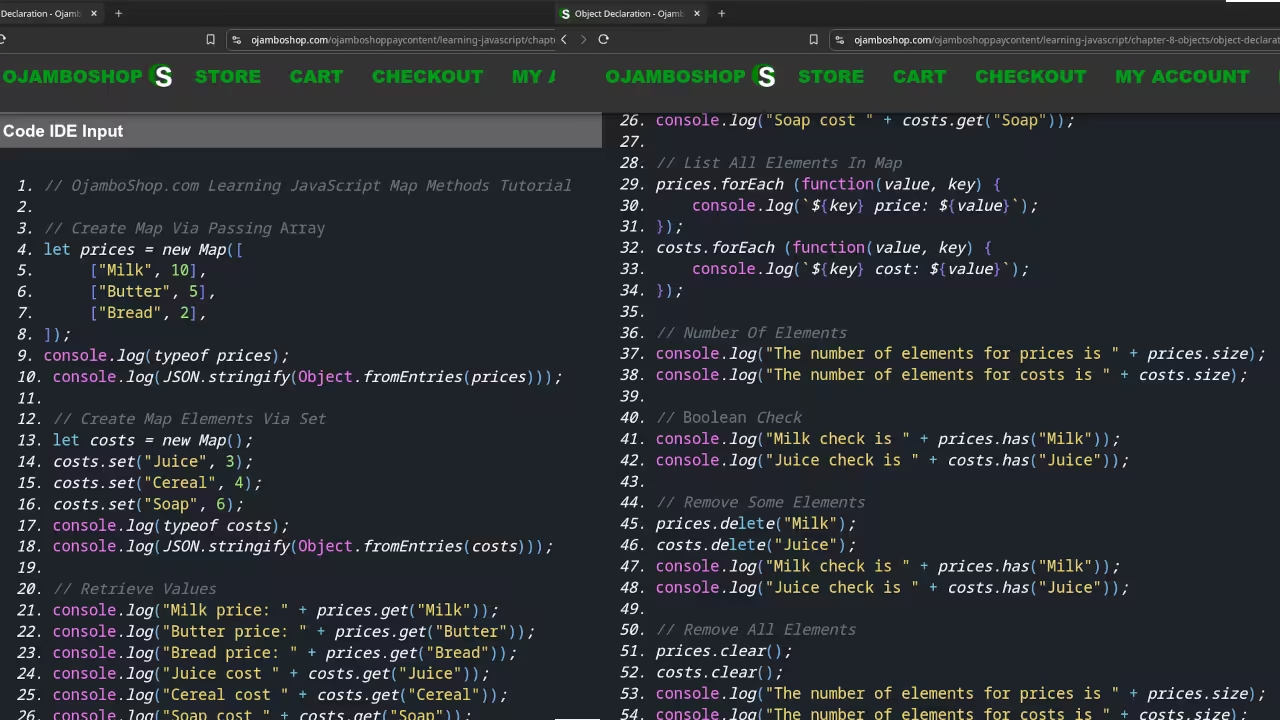
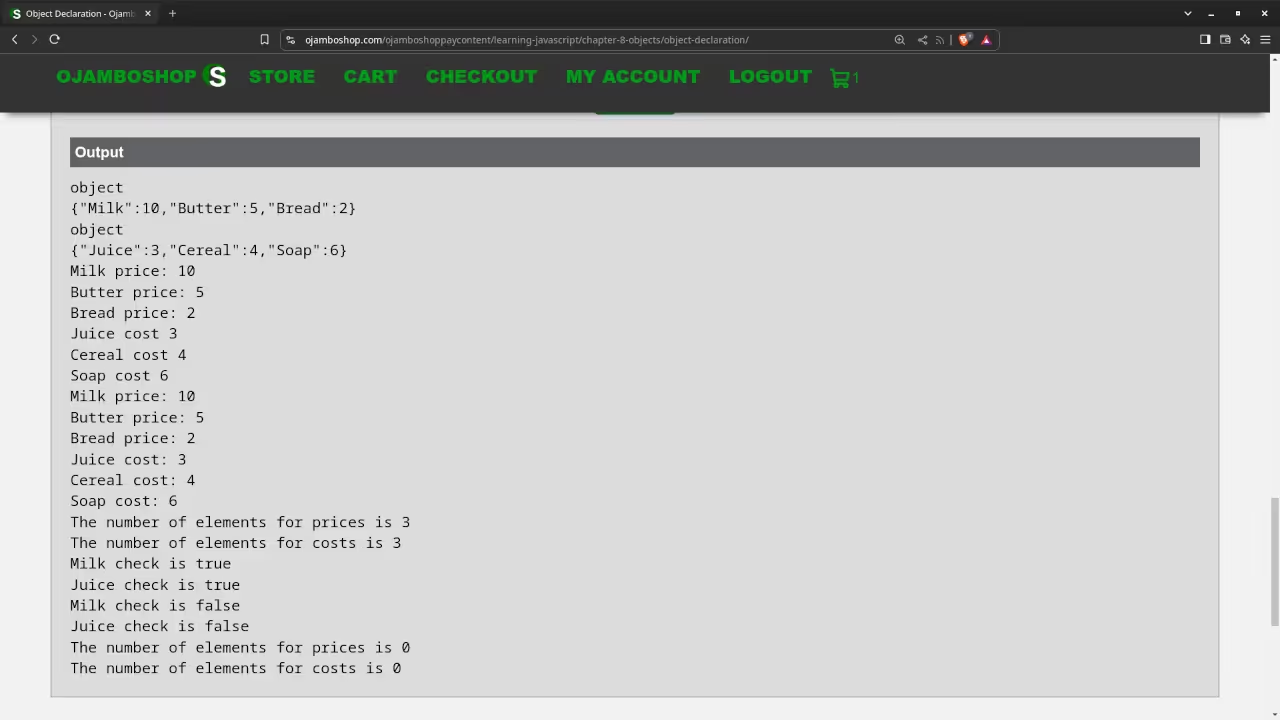
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the Map Methods.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Live Stream
Every Friday, you can join a live stream and ask questions. Check Ojambo.com for details and instructions.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
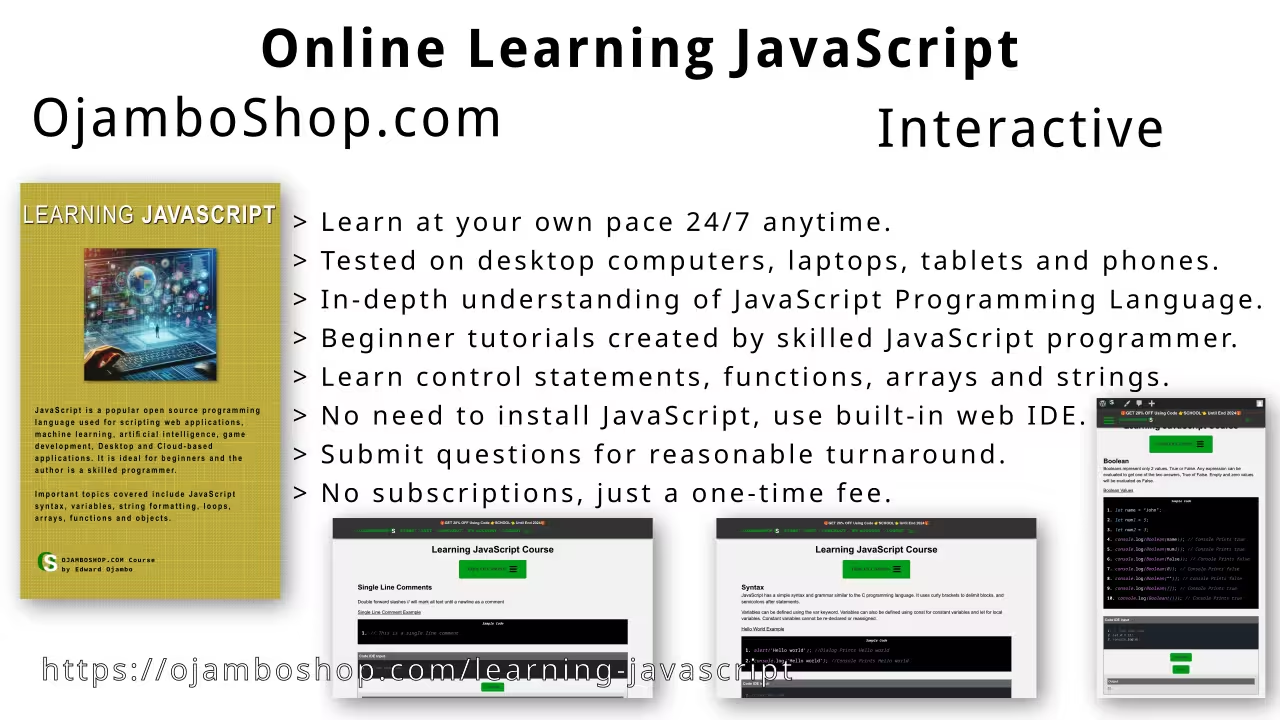
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
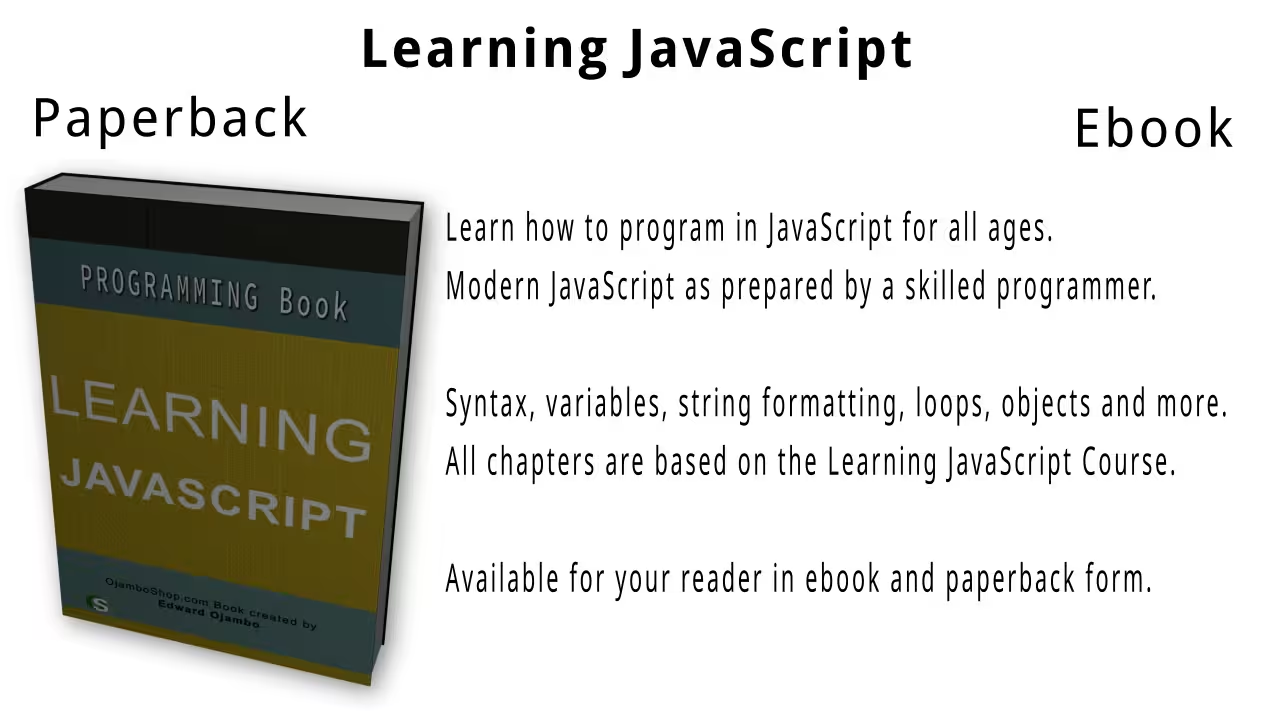
Conclusion:
JavaScript makes it easy to create Maps and use Map Methods. Map are used to store key-value pairs of any data type and Map Methods manipulate elements and values.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials