For In Loop In JavaScript
Loops execute one or more statements up to a specific number of times.
Loops allow repetitive tasks to be executed using minimal code. For in loop iterates over the enumerable properties on an object.
Loops allow complex tasks to be simplified by automating similar operations.
Common Syntax For In Loop In JavaScript
Common For In Loop Conditions
Name | Description | Example |
---|---|---|
for (key in object) | loop assigns object key | for (let key in pickup_truck) |
for (key in array) | loop assigns array key | for (let n in even_numbers) |
break | End execution of current structure | break; |
continue | Skip rest of current loop iteration | continue; |
Name | Description | Example |
JavaScript For In Loop Snippet
// OjamboShop.com Learning JavaScript For In Loop Tutorial // let pickup_truck = {"brand":"Ford", "model":"F-Series", "year":1984}; let even_numbers = [2,4,6,8,10]; // Without Loops console.log(pickup_truck["brand"]); console.log(pickup_truck.brand); console.log(pickup_truck.model); console.log(pickup_truck.year); console.log(even_numbers[0]); console.log(even_numbers[1]); console.log(even_numbers[2]); console.log(even_numbers[3]); console.log(even_numbers[4]); // For In Loop for (let key in pickup_truck) { console.log(key + ": " + pickup_truck[key] + "\n"); } for (let n in even_numbers) { console.log(n + ": " + even_numbers[n] + "\n"); }
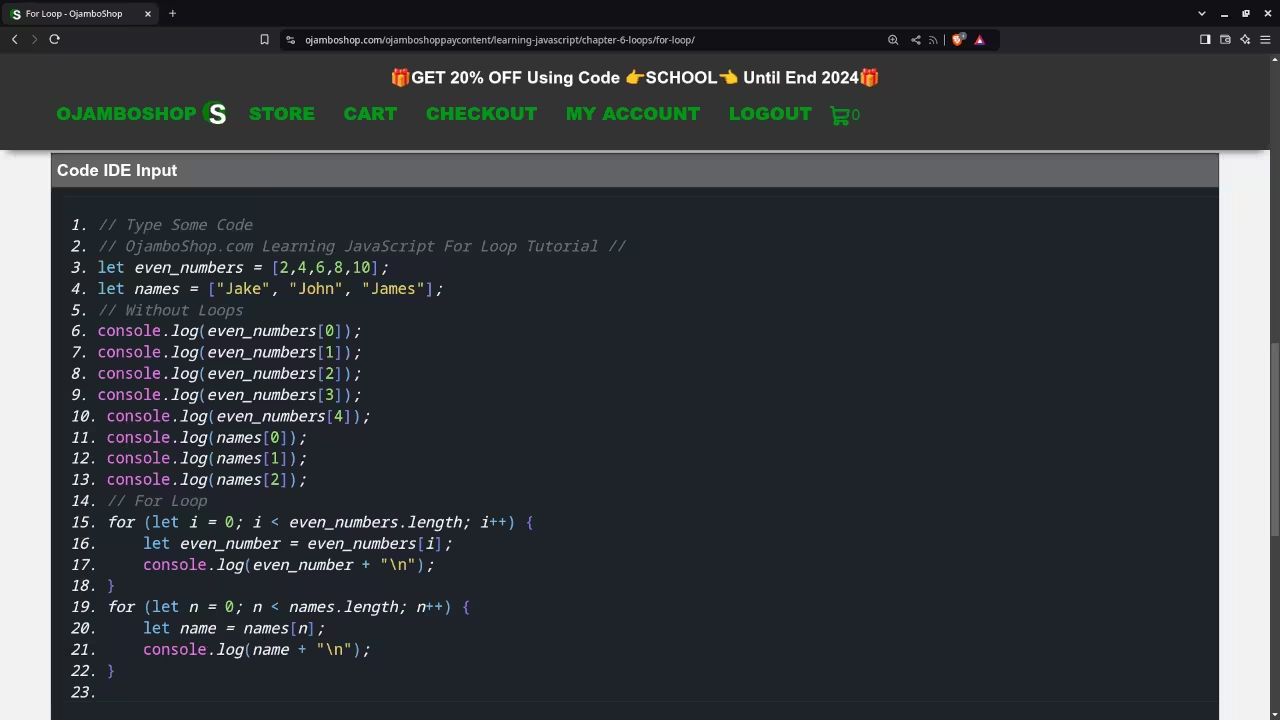
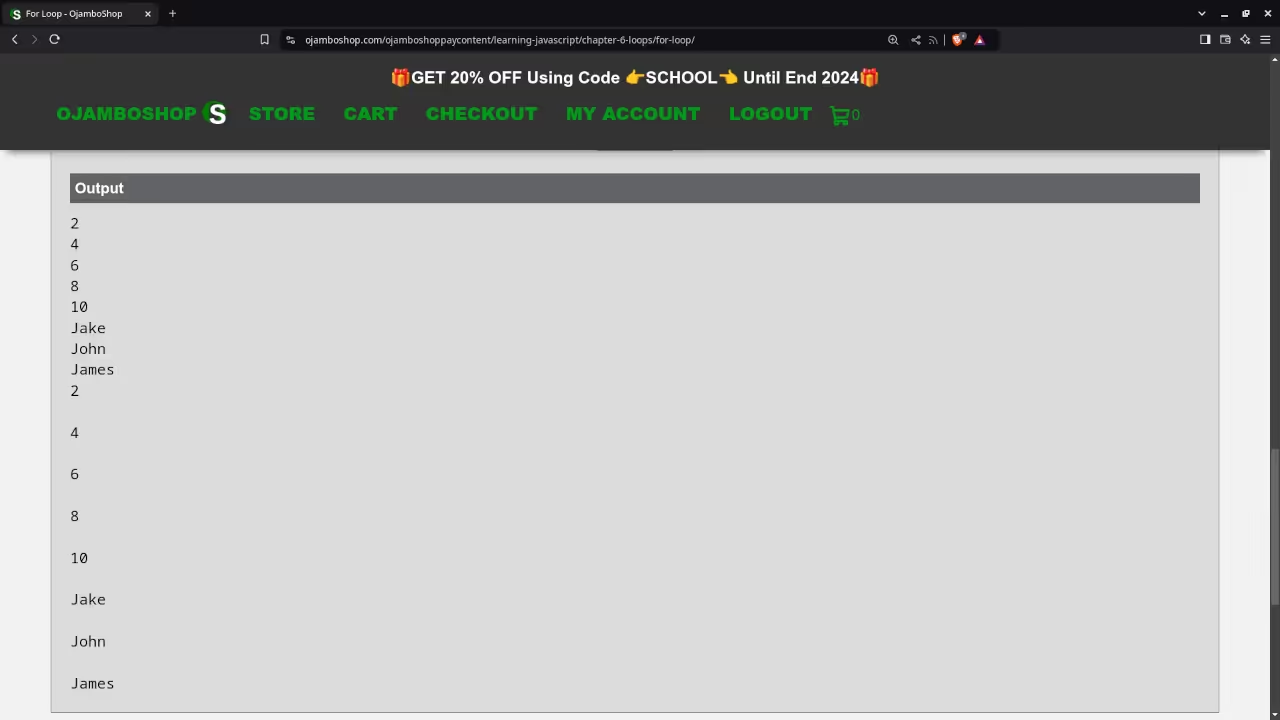
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE was used to input and compile JavaScript code for the for in loop.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
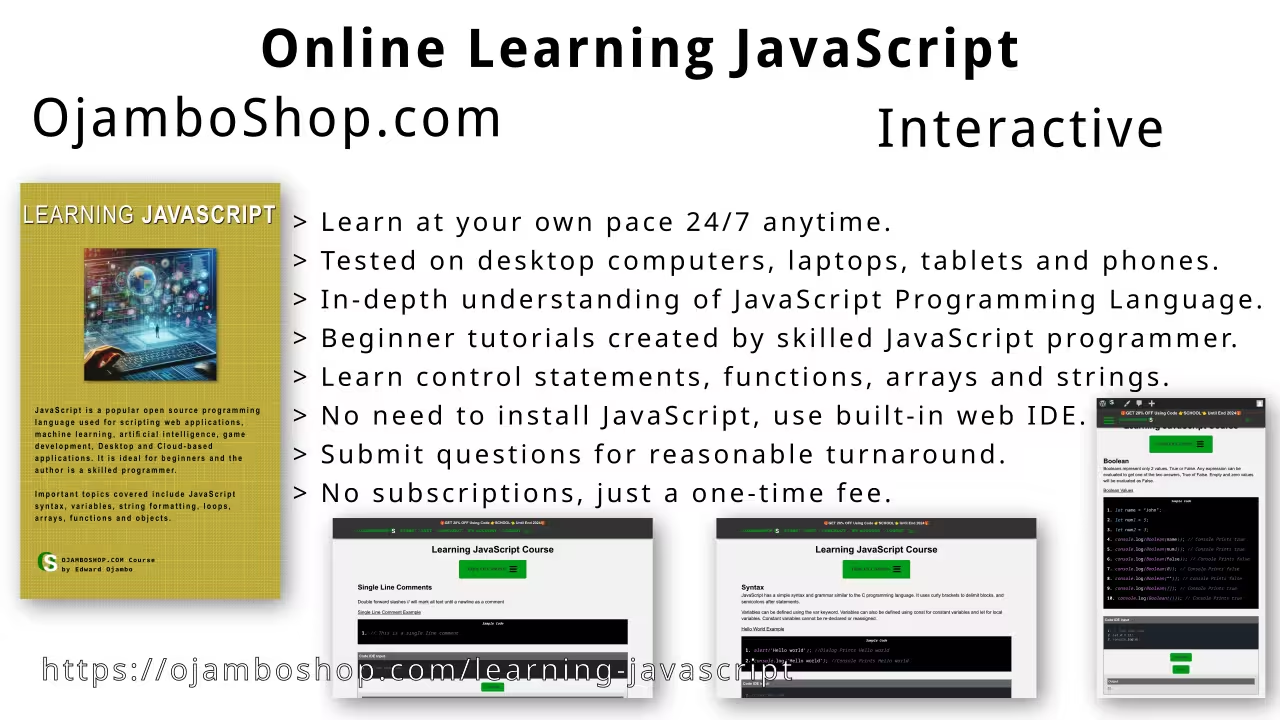
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning JavaScript Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
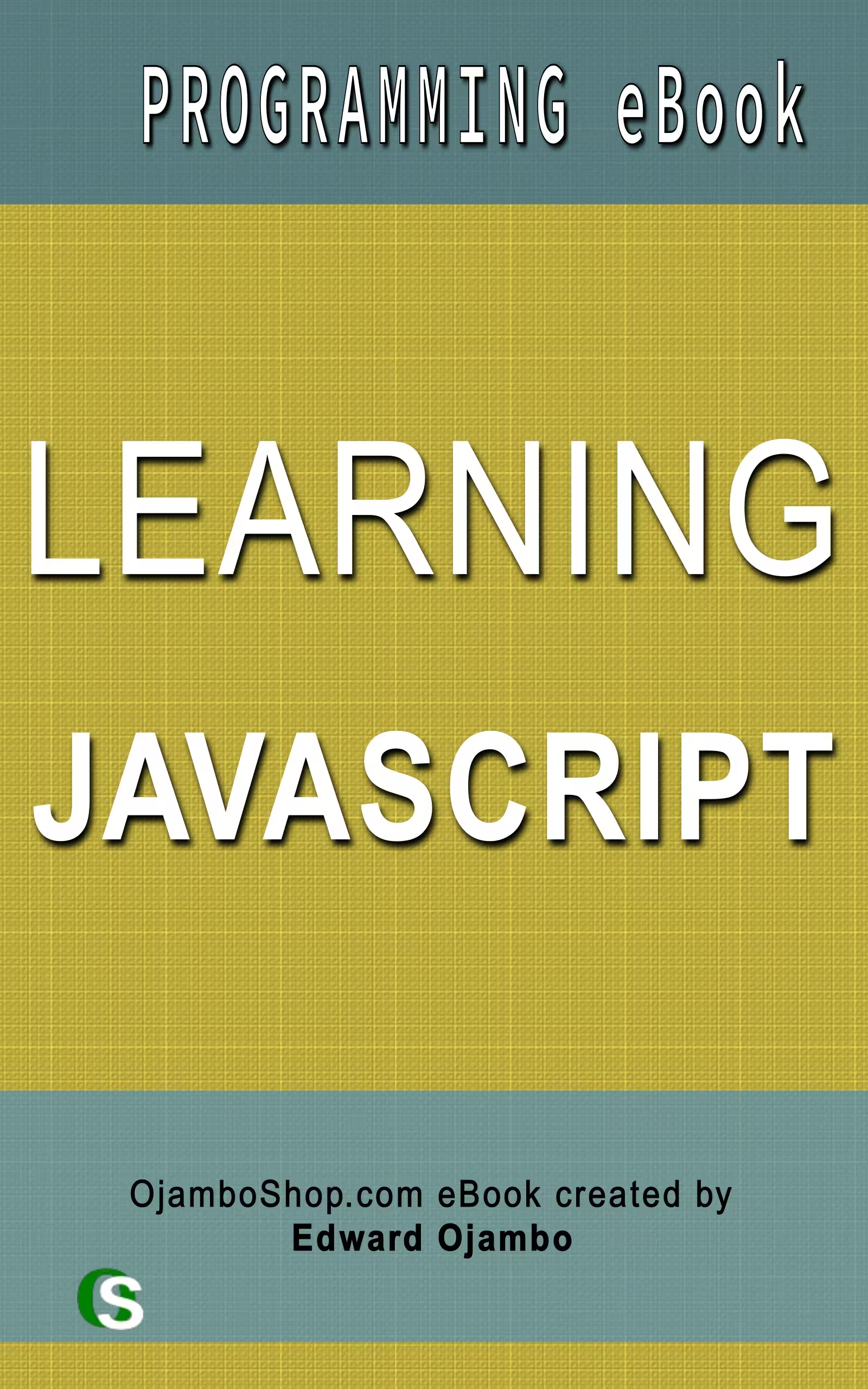
Conclusion:
JavaScript makes it easy to use for in loop statements. Use a for in loop to iterate over the enumerable properties on an object.
Take this opportunity to learn the JavaScript programming language by making a one-time purchase at Learning JavaScript Course. A web browser is the only thing needed to learn JavaScript in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning JavaScript Ebook
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials