Perform Bitwise OR Assignment Operations In JavaScript
A bitwise operation takes values and operates at the most basic unit of information whose logical state is represented by 1 or 0.
JavaScript bitwise operators convert numbers to 32-bit integers otherwise it will use BigInt if applicable. Therefore bitwise OR (|) binary operation compares each bit of 2 operands and if one bit is 1 will return 1, otherwise will return 0.
An operand is the object of a mathematical operation. Operands for the bitwise OR are integers in the form of numbers of BigInt and a logical inclusive OR operation is performed on each.
Bitwise OR Assignment Operators For JavaScript
Glossary:
Binary Operation
Mathematical rule for combining two operands to produce another.
Logical Inclusive OR (||)
Indicates where one operands is true.
BigInt
Used to represent numeric values too large for number data type.
Truth Table
Illustrate the behavior of bitwise logical operators.
Bitwise OR Assignment Operators
Name | Description | Example | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|= | Bitwise OR assignment result to left operand | num1 |= 5; | |||||||||||||||
| | Bitwise OR | num1 | num2;
|
|||||||||||||||
Name | Description | Example |
JavaScript Bitwise OR Assignment Operators Snippet
// OjamboShop.com Learning JavaScript Bitwise OR Assignment Operators Tutorial let num1 = 5; // Binary 101 let num2 = 3; // Binary 11 let num3 = 1234567890123456789012345678901234567890n; // Binary 1110100000110010010010000001110101110000001101101111110011101110001010110010111100010111111001011011001110001111110000101011010010 let num4 = 12n; // Binary 1100 console.log(num1 | num2); console.log(num2 | num1); console.log(3 | num1); console.log(5 | num2); // BitInt console.log(num3 | num4); console.log(num4 | num3); console.log(5n | 3n); // Bitwise Or Assignment let num5 = 10; // Binary 1010 num5 |= 6; // Binary 110 console.log(num5);
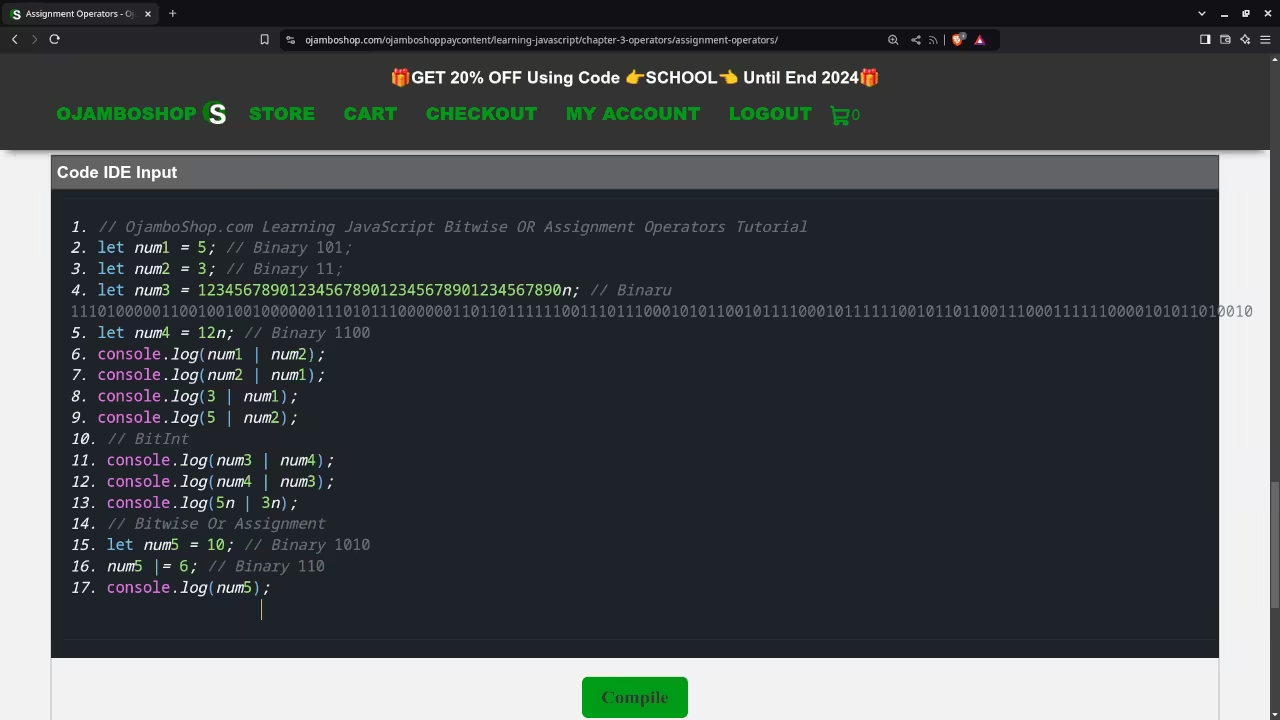
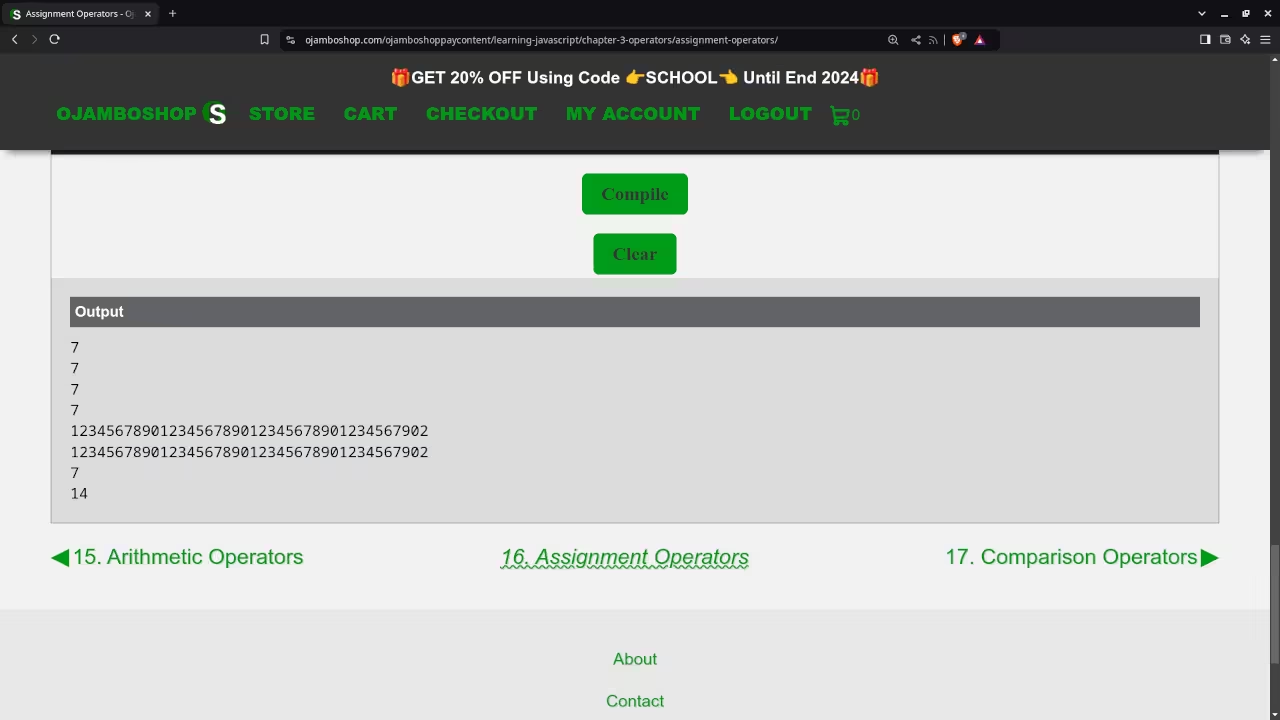
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the bitwise OR assignment operators.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
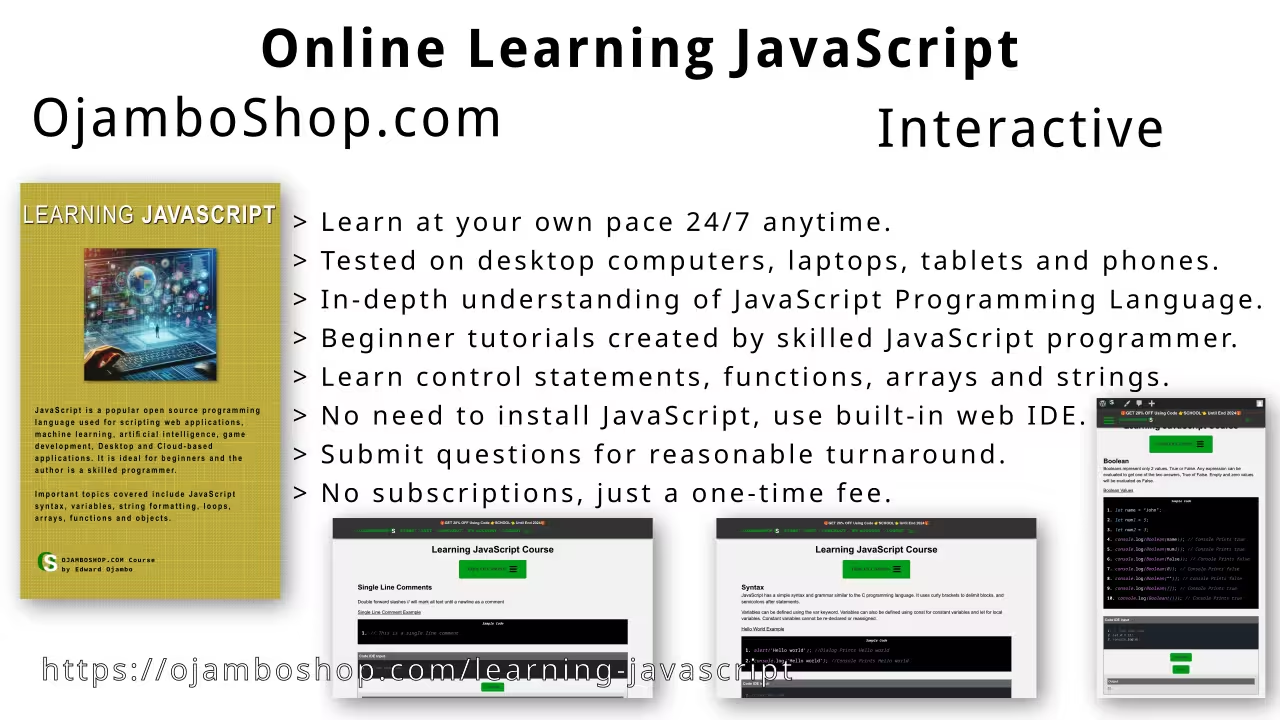
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning JavaScript Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
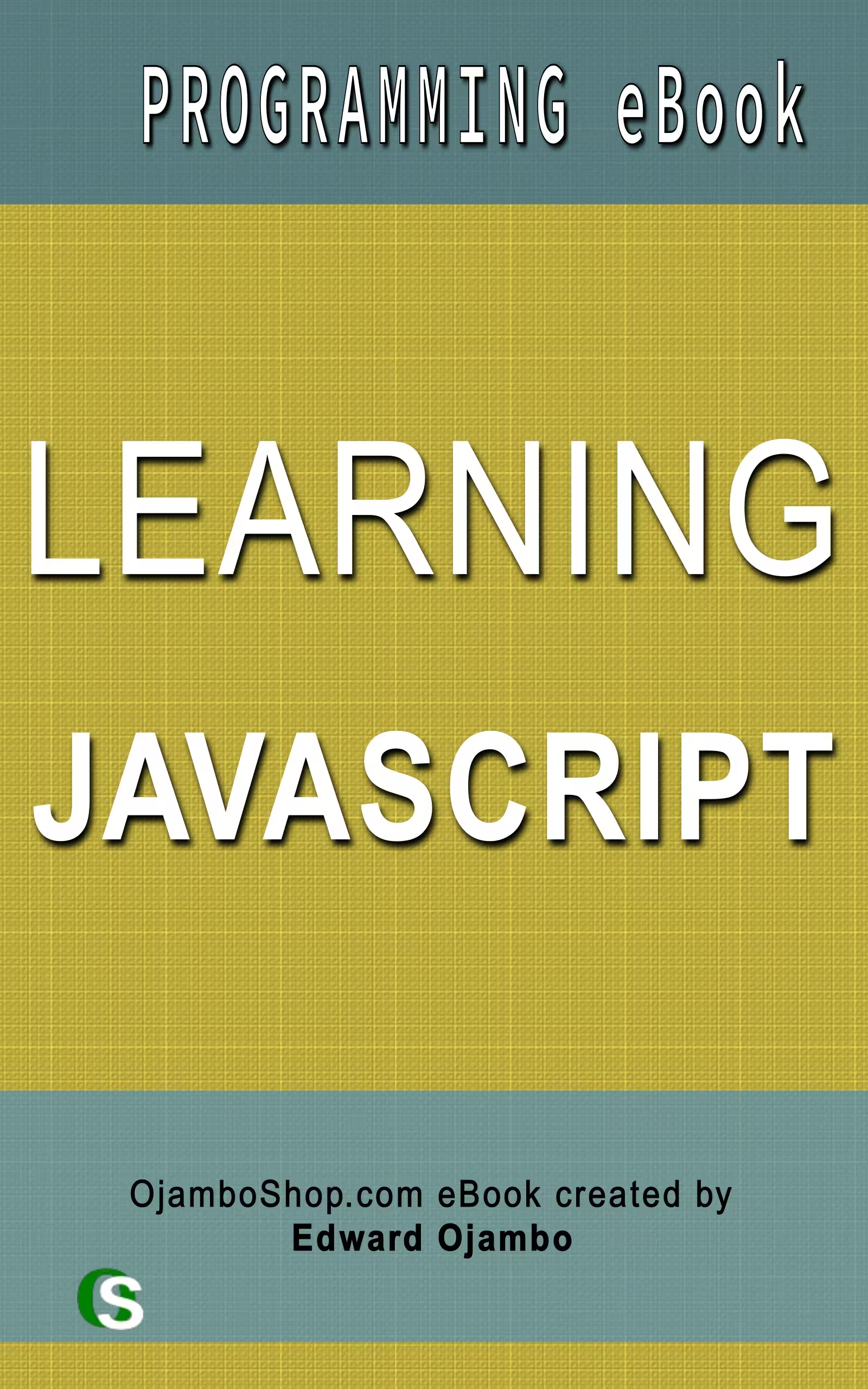
Conclusion:
JavaScript makes it easy to use the bitwise OR assignment operators. Use bitwise OR assignment operators to compare two values of the same numeric integer type to return 1 if one matches.
Take this opportunity to learn the JavaScript programming language by making a one-time purchase at Learning JavaScript Course. A web browser is the only thing needed to learn JavaScript in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning JavaScript Ebook
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials