Perform BigInt Datatype In JavaScript
In JavaScript, assigning of a value will indicate the data type. Get the data type of any object using the built-in typeof function.
The BigInt type is for storing large integers that can be beyond the safe integer limit. Unsigned right shift (>>>) can not be done on a BigInt.
BigInt is designed to work with arbitrary-precision integers, not floating-point numbers. BigInt data types can be positive or negative. Scientific numbers with the letter “e” can be used to indicate the power of 10..
Common Syntax Of BigInt Datatype In JavaScript
Glossary:
Data Type
Collection or grouping of data values.
Precision
Measure of detail in which the quantity is expressed.
Accuracy
Measures the deviation between the set point and the actual output.
Common BigInt Datatype
Name | Description | Example |
---|---|---|
toString() | Returns number as a string. | str1 = num1.toString(); |
toExponential() | Returns number in exponential notation. | num2 = num1.toExponential(2); |
valueOf() | Returns number as number. | num2 = num1.valueOf(); |
Number() | Returns converted number. | num2 = Number(10n); |
parseInt() | Returns whole number. | num2 = parseInt(“10n”); |
Name | Description | Example |
JavaScript BigInt Datatype Snippet
// OjamboShop.com Learning JavaScript BigInt Datatype Tutorial // Data Type my_int = 10n; console.log(my_int); // Console Prints 10n console.log(typeof(my_int)); // Console Prints bigint // Casting Integers let int1 = Number(5n); // Will be 5 let int2 = Number(9n); // Will be 9 console.log(int1); // Console Prints 5 console.log(int2); // Console Prints 9 // Integer Numbers let int4 = 5n; let int5 = 3n; let int6 = -9n; console.log(int4); // Console Prints 5n console.log(int5); // Console Prints 3n console.log(int6); // Console Prints -9n // Manipulating Numbers let int7 = 1234567890123456789012345678901234567890n; console.log(int7.toString()); console.log(int7.toLocaleString('en-US', {notation: 'scientific', maximumFractionDigits: 2})); // Exponential console.log(int7.valueOf(2)); console.log(Number(int7)); console.log(parseInt(int7));
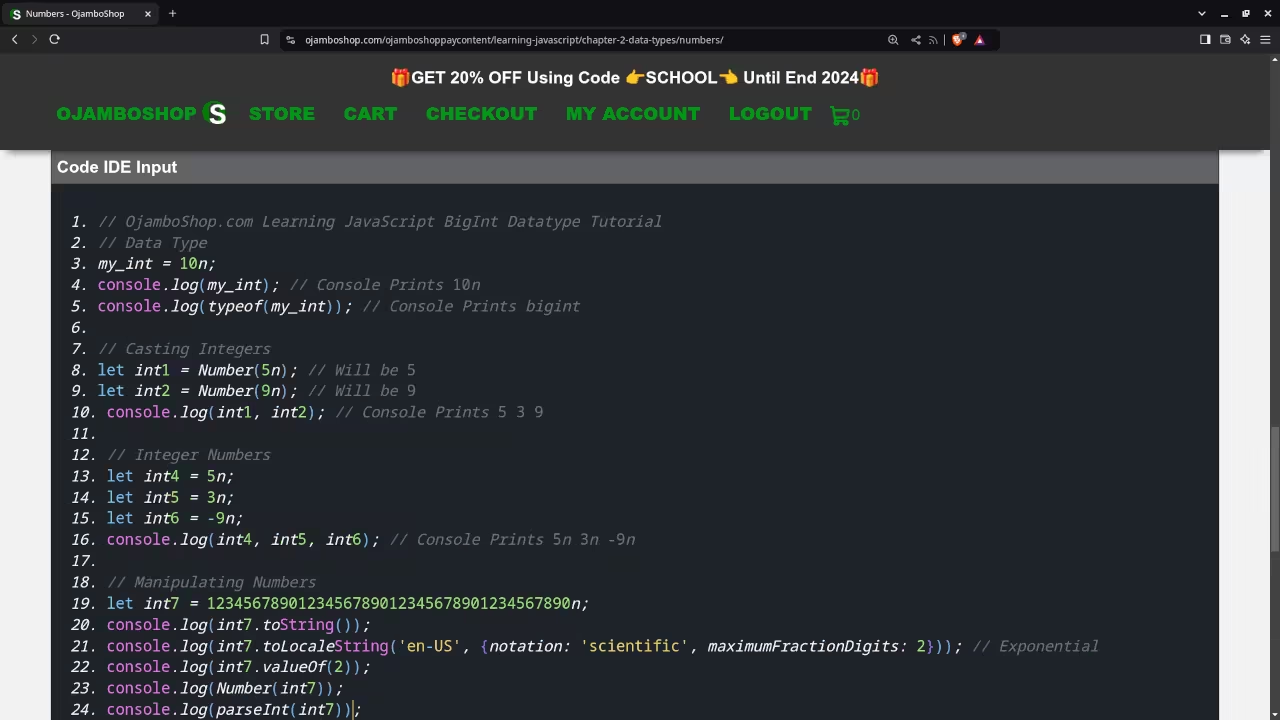
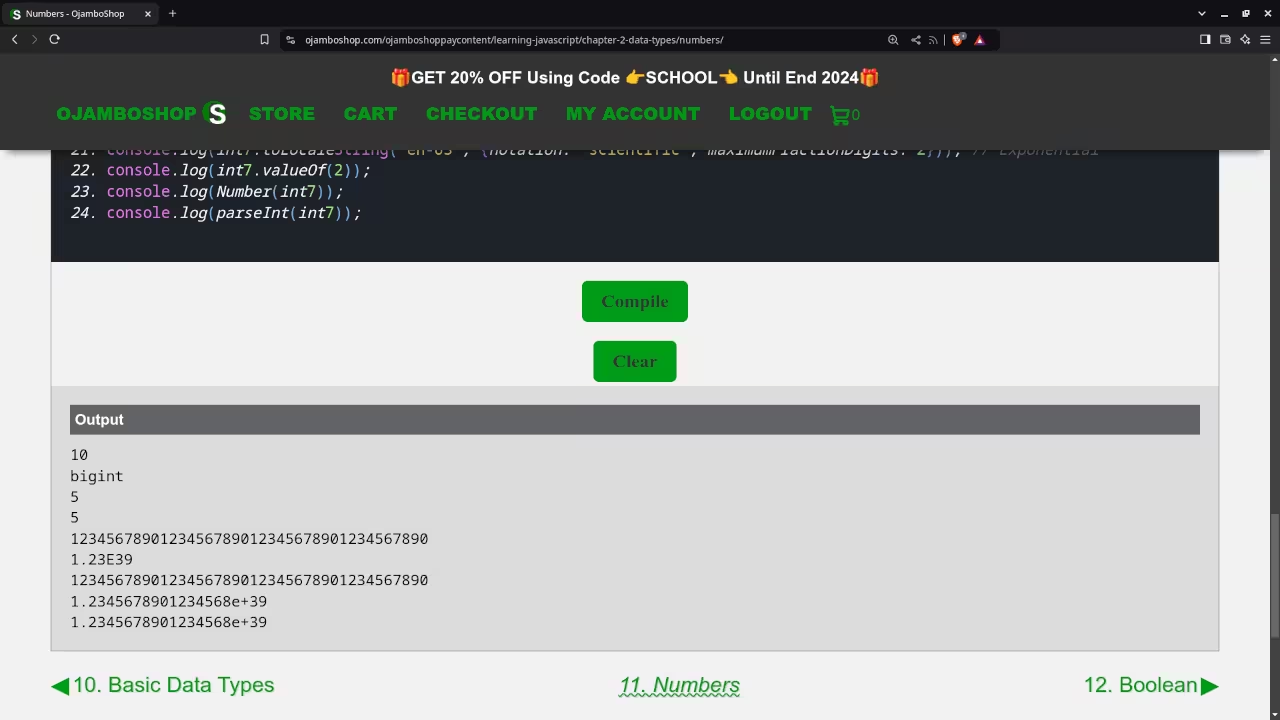
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the BigInt data type.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
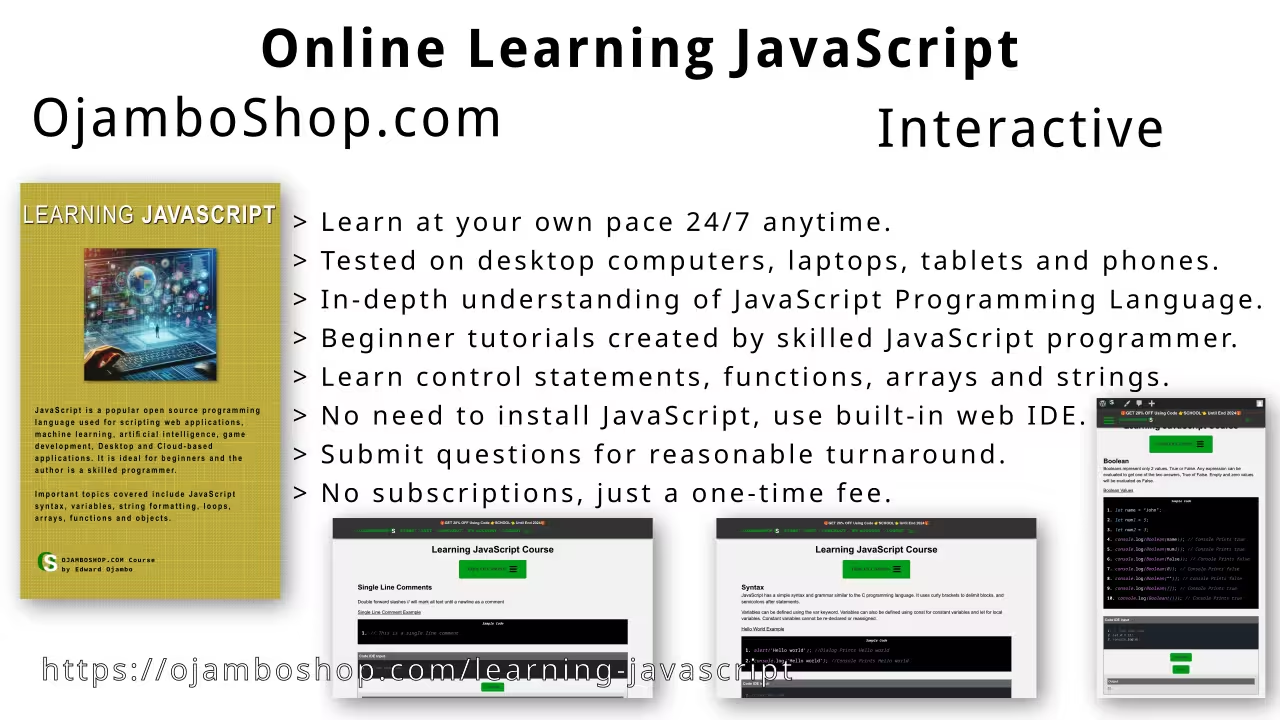
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning JavaScript Course until End Day 2024.
Learn Programming Books:
Ebooks can be downloaded to your reader of choice.
Paperback version is now available.
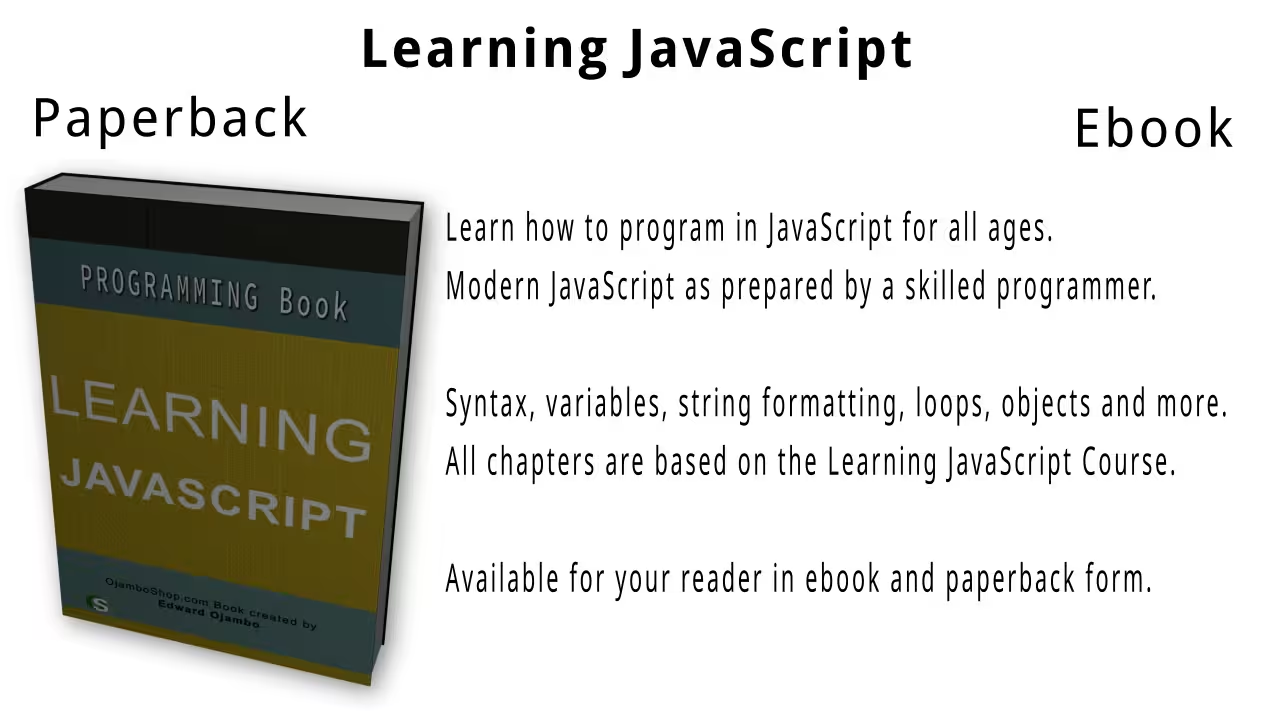
Conclusion:
JavaScript makes it easy to use the BigInt data type. Use the BigInt data type to store integer values that are too big to be represented by a normal numeric data type.
Take this opportunity to learn the JavaScript programming language by making a one-time purchase at Learning JavaScript Course. A web browser is the only thing needed to learn JavaScript in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning JavaScript Ebook
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback and Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials