Create Anonymous Functions In JavaScript
In JavaScript, a function declaration defines a function with the specified parameters and code block.
Functions can be called before their creation due to hoisting. A JavaScript function is a subprogram that can be called by code externally or internally to the function. Anonymous functions do not have a name and are often assigned as variables passed as parameters to other functions.
Anonymous functions can be assigned as local variables with closures. Anonymous functions are most useful for event handlers and callbacks.
Common Syntax Of Anonymous Functions In JavaScript
Glossary:
Hoisting
The interpreter moves function declaration to the top of their scope.
Scope
The accessibility of variables and functions within certain parts of your code.
Declaration
Used to define variables, functions, and constants.
Method
Function defined within a object.
Arrow Functions
Syntax (=>) for creating anonymous expressions.
Closures
Access variables from outer scope even after outer function has finished.
Recursion
Method to call itself to solve a problem.
Constructor
Special method within a class used to initialize and create objects of that class.
Implicit Return
Return a value without using the return keyword.
Common Anonymous Functions
Name | Description | Example |
---|---|---|
function() {} | Anonymous function. | function() { return “John”; } |
(() => {} )(); | Anonymous function with arrow function. | (() => { return “John”; })() |
Name | Description | Example |
JavaScript Anonymous Functions Snippet
// OjamboShop.com Learning JavaScript Anonymous Functions Tutorial // Anonymous Function let namef = function() { return "John"; }; console.log("The anonymous function returned: " + namef()); // Anonymous Function Arrow Function let namefa = () => { return "Jane"; }; console.log("The anonymous function arrow function returned: " + namefa()); // Anonymous Function Arrow Function Arguments let namefaa = (name) => { return "The anonymous function arrow function with argument returned: " + name; }; console.log(namefaa('Jake')); // Self-Executing Anonymous Function (function () { console.log("The self-executing anonymous function is complete"); })(); // Self-Executing Anonymous Function As Callback let counter = 0; let interval = setInterval(function () { counter += 1; if (counter === 5) { clearInterval(interval); } console.log("The self-executing anonymous function counter is: " + counter); }, 1000); // Runs Every 1000 milliseconds
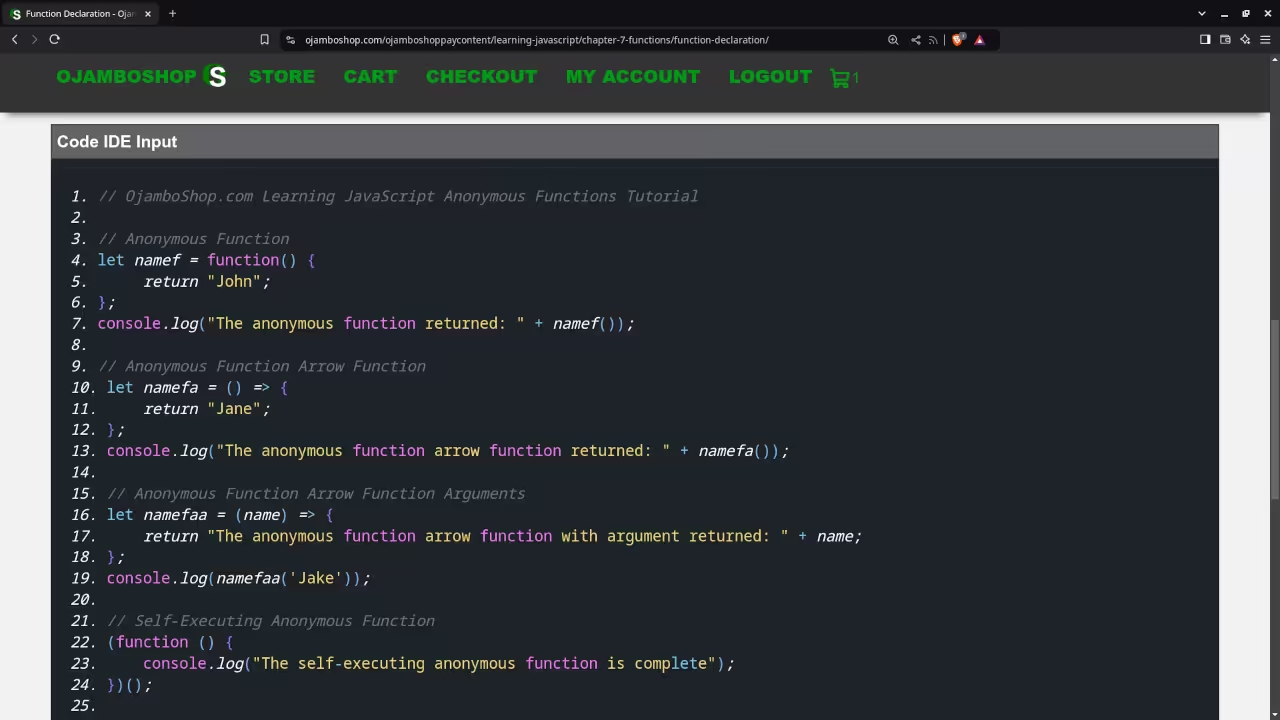
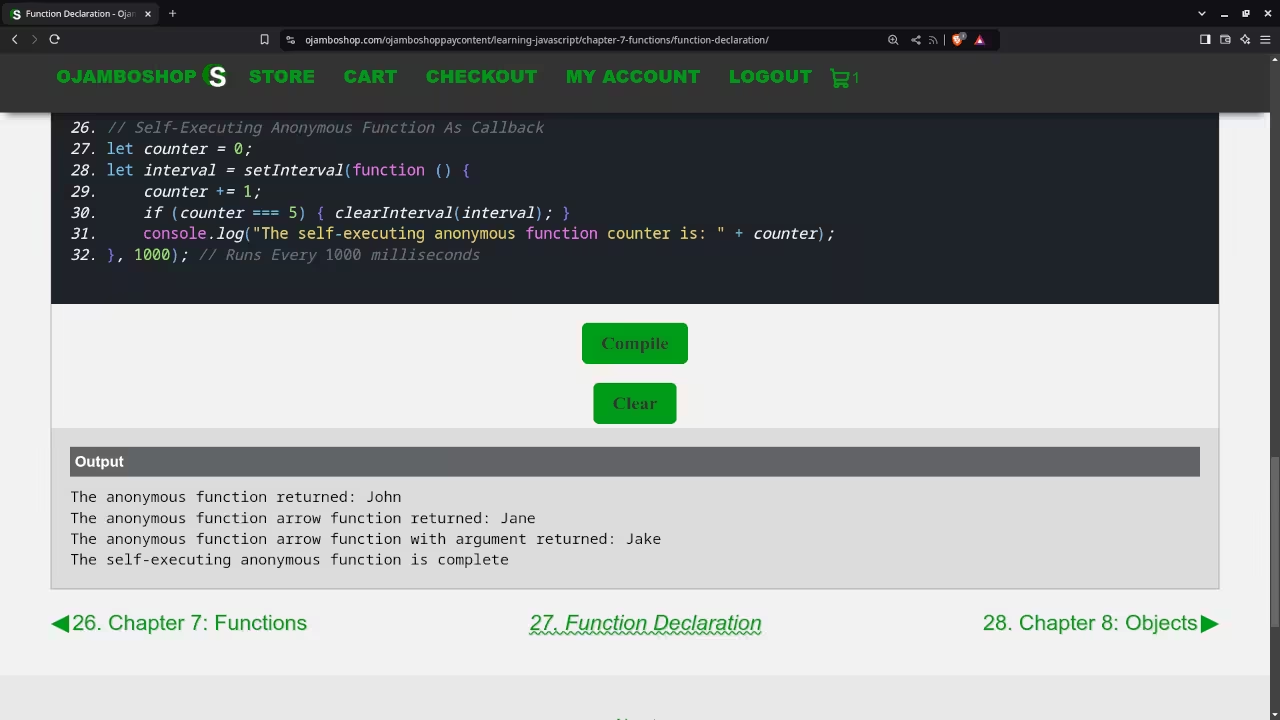
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the anonymous functions.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Live Stream
Every Friday, you can join a live stream and ask questions. Check Ojambo.com for details and instructions.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
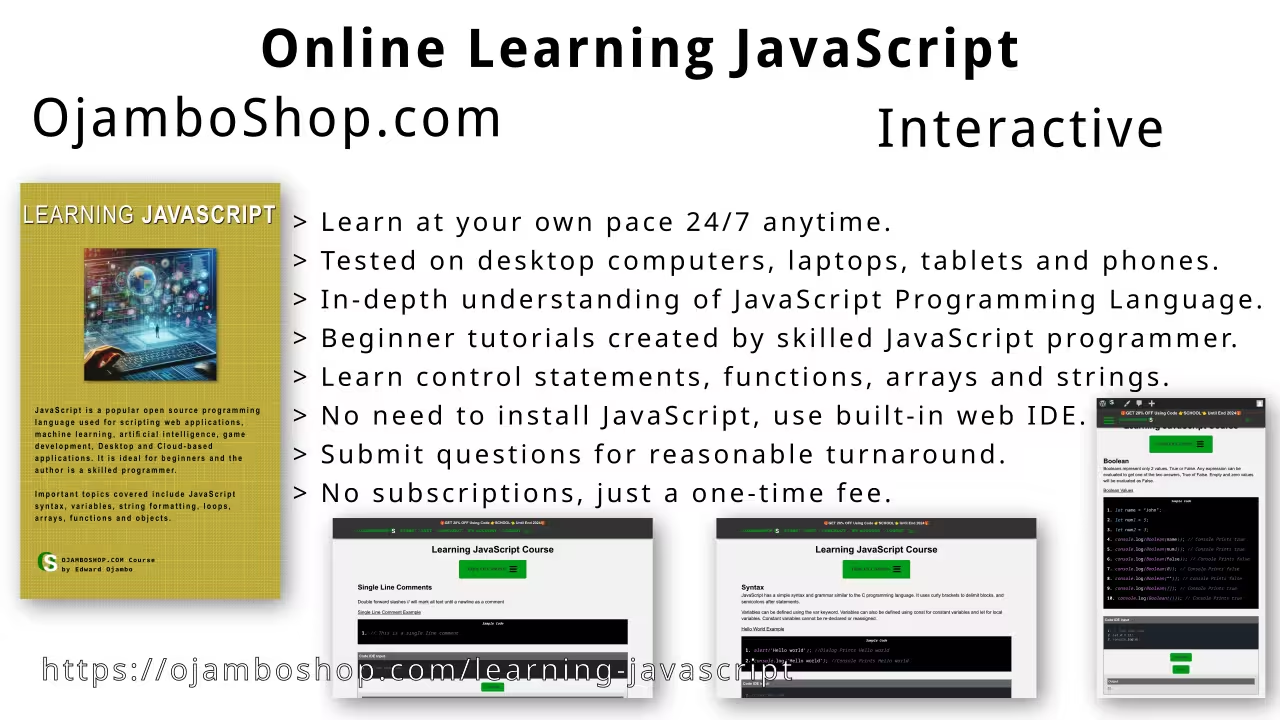
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
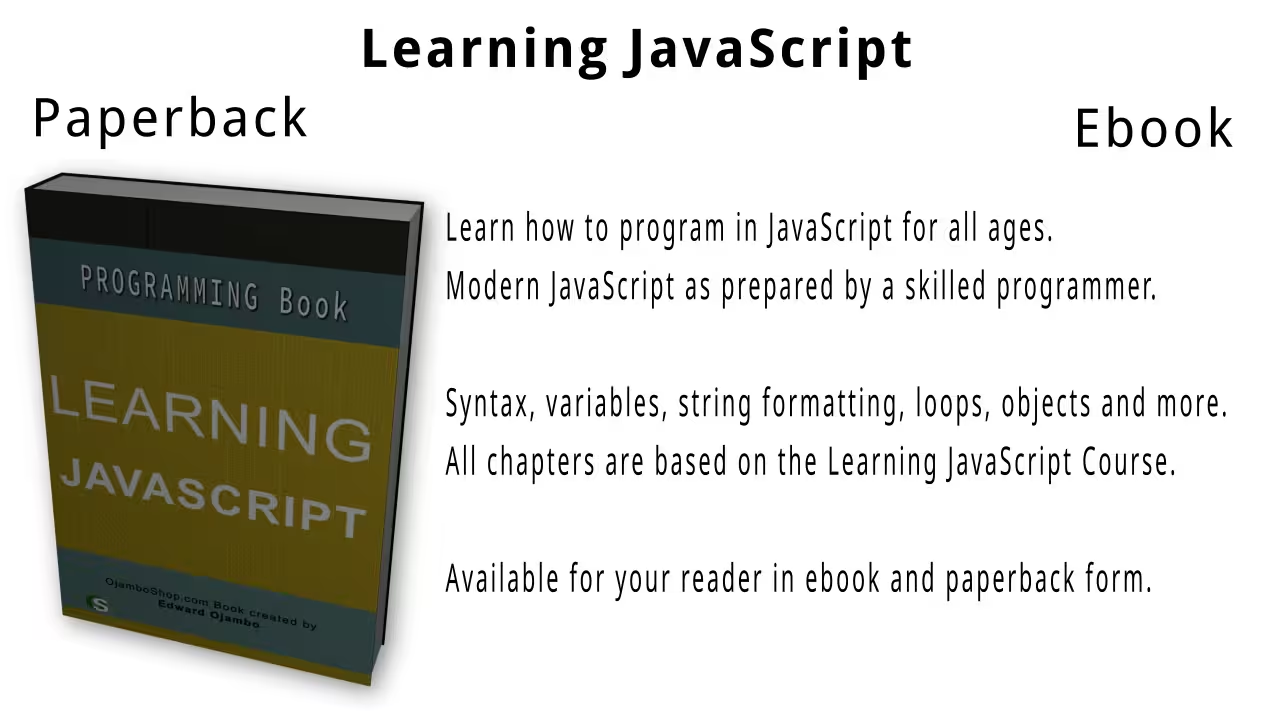
Conclusion:
JavaScript makes it easy to use create anonymous functions. Anonymous functions do not have a name and can be defined inline to be passed as arguments or assigned to variables.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials