Using Python GUI To Run Command Line Programs
The output of command line terminal programs can be manipulated by Python. Python is a programming language and Tkinter is a lightweight GUI framework.
Tkinter comes bundled with Python version 3 on Linux, macOS and Windows. It is the Python interface to the Tk GUI toolkit. Th python subprocess module is used with Popn interface directly.
Combine command line code with GUI Tkinter and let Python control everything. You can find more information about Tkinter GUI in the Cross Platform Python Desktop GUI Using Tkinter article.
Python GUI Window For Command Line Programs
Check If MySQL Service Is Running
Name | Description | Example |
---|---|---|
ps [OPTION …] | Report a snapshot of the current processes | ps aux |
grep [OPTION …] PATTERNS [FILE …] | print lines that match patterns | grep “mysqld” |
head [OPTION …] [FILE …] | Output the first part of files | head -1 |
systemctl [OPTION …] COMMAND [UNIT …] | Control the systemd system and service manager | systemctl start mysqld |
pipe | Redirect standard ouput, input, or error of one process to another | Command-1 | Command-2 | … | Command-N |
Name | Description | Example |
# GUI To Execute Command Line Service Or Code Using Python Inspired By OjamboShop.com/learning-python Course # Import Tkinter Module And Create Instance Of Tkinter's Tk Class import tkinter as tk import subprocess window = tk.Tk() window.title("My App") window.geometry("400x400") class App: def __init__(self, master): self.services = [['mysql', r'ps aux | grep "mariadbd" | grep -v grep | head -1 | grep basedir', 'systemctl start mariadb', 'systemctl stop mariadb']] # Text Widget self.txt_output = tk.Text(master, height=10) self.txt_output.pack() self.running_services() # Start Button self.btn_start = tk.Button(master, text="Start Services", command=self.start_services) self.btn_start.pack() # Stop Button self.btn_stop = tk.Button(master, text="Stop Services", command=self.stop_services) self.btn_stop.pack() def running_services(self): for service, check, start, stop in self.services: out, err = self.run_command(check) if service in str(out).lower(): self.txt_output.insert(1.0, service + " is running\n") else: self.txt_output.insert(1.0, service + " is not running\n") def start_services(self): self.txt_output.delete(1.0, tk.END) for service, check, start, stop in self.services: out, err = self.run_command(check) if service in str(out).lower(): self.txt_output.insert(1.0, service + " is running\n") else: out, err = self.run_command(start + ' && ' + check) if service in str(out).lower(): self.txt_output.insert(1.0, service + " is running\n") else: self.txt_output.insert(1.0, service + " is not running " + str(err)) def stop_services(self): self.txt_output.delete(1.0, tk.END) for service, check, start, stop in self.services: out, err = self.run_command(stop + ' && ' + check) if service in str(out).lower(): self.txt_output.insert(1.0, service + " is running\n") else: self.txt_output.insert(1.0, service + " is not running\n") def run_command(self, cmd): proc = subprocess.Popen(cmd, shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE) outs, errs = proc.communicate() return outs, errs # Create Object Named app app = App(window) # Run Tkinter Event Loop window.mainloop()
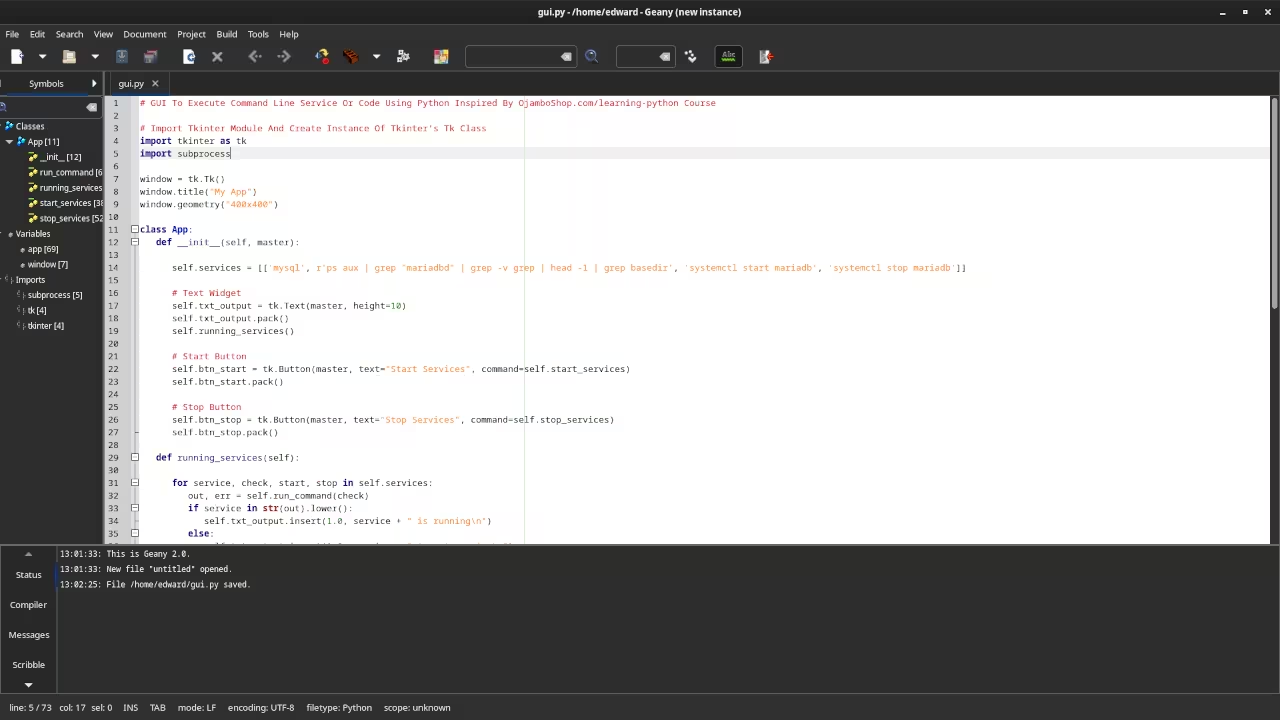
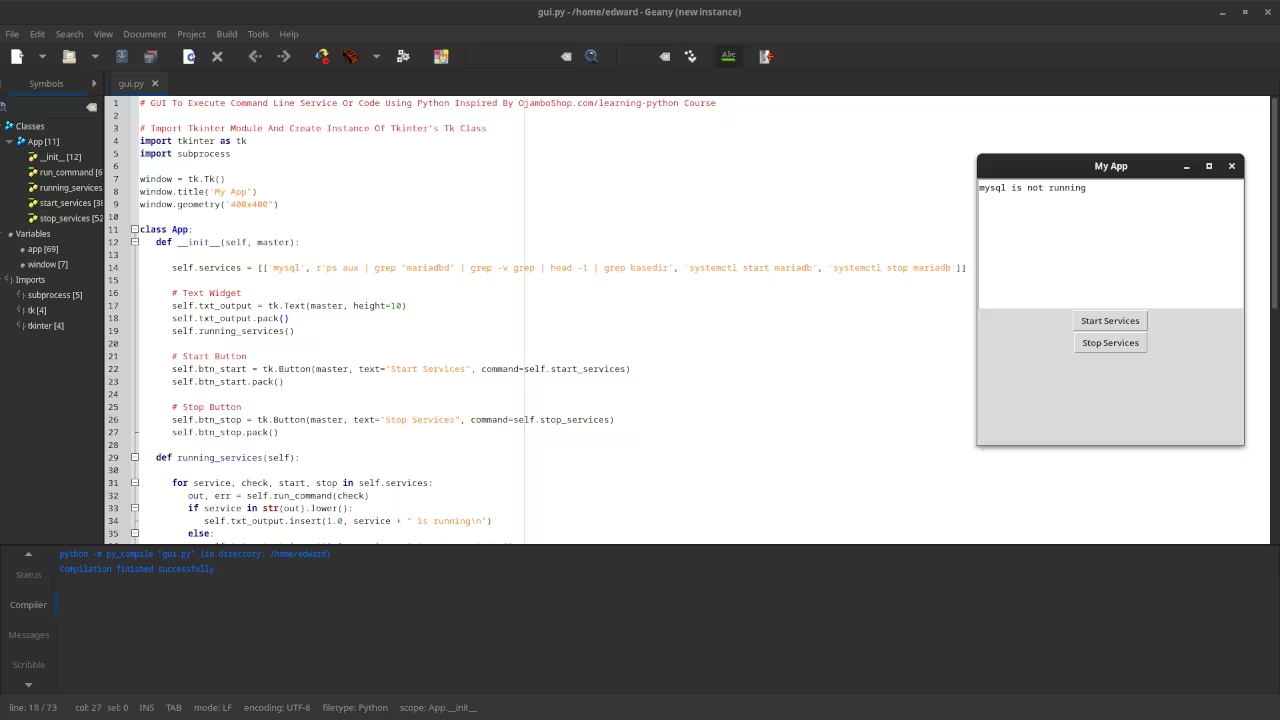
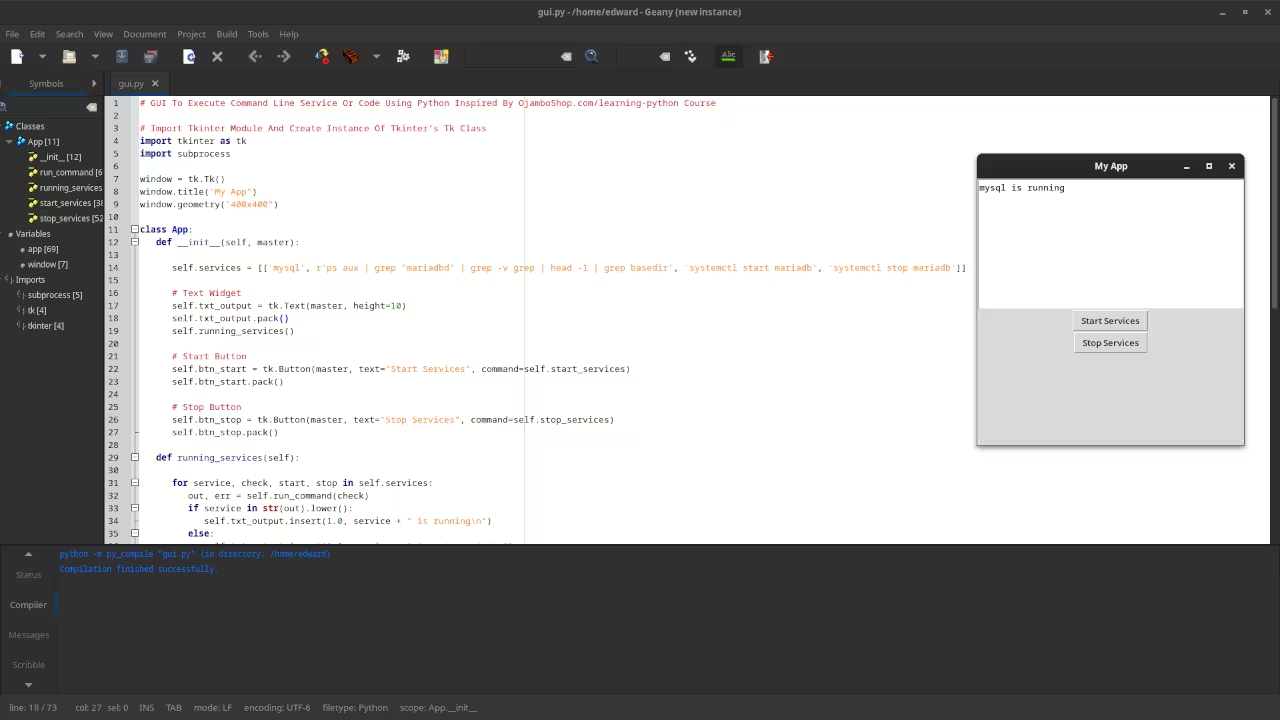
Usage
You can use any IDE, text editor or the Python interactive shell to execute command line code in a GUI using Python. In this tutorial, command line programs were used to determine in the MySQL service is running, Tkinter was used to generate a GUI and Python was used for the logic.
Open Source
Python is licensed under the Python Software Foundation License. This allows commercial use, modification, distribution, and allows making private derivatives. The PSF License is compatible with other open source licenses.
Learning Python:
Course is optimized for your web browser on any device.
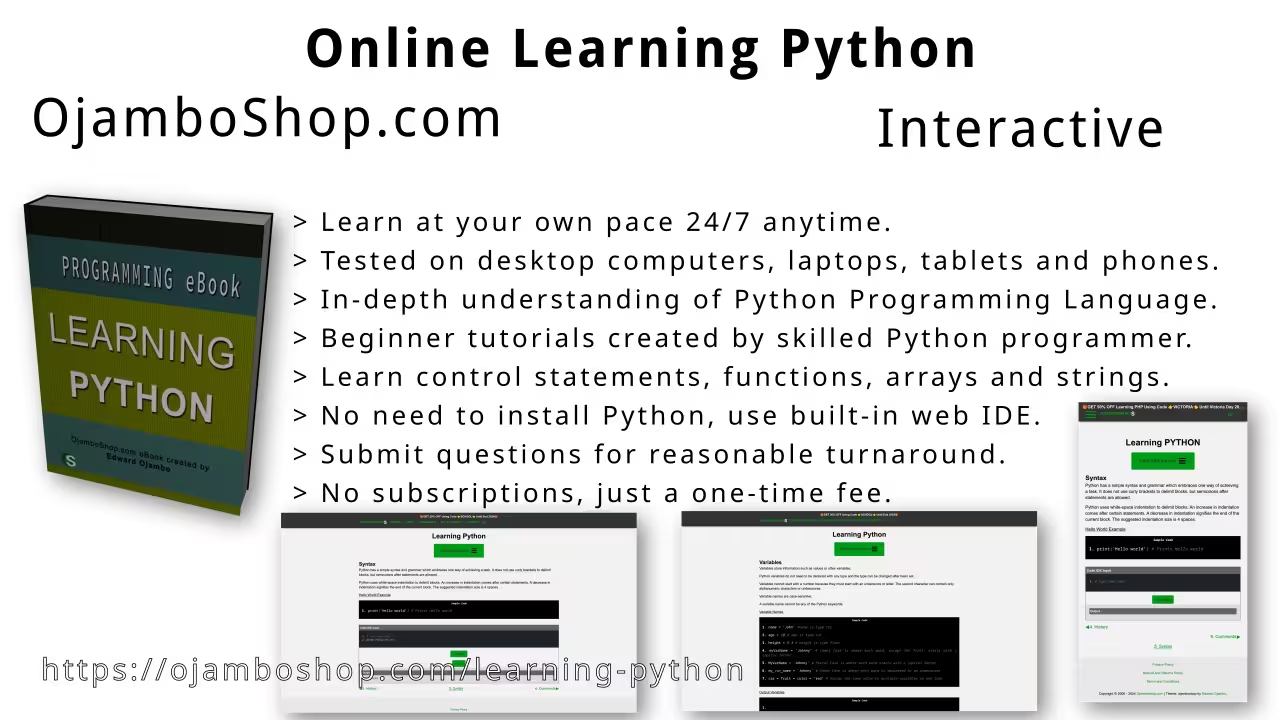
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python until End Day 2024.
Conclusion:
Python makes it easy to create a GUI for command line services or code. Command line ouput is controlled by the subprocess module and the GUI is generated using Tkinter.
Take this opportunity to learn Python programming language by making a one-time purchase at Learning Python. A web browser is the only thing needed to learn Python in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- GNU Bash Project Shell
- Cross Platform Python Desktop GUI Using Tkinter Article
- Python Software Foundation License