Build GUI For Desktop Applications Using Node.js And Tauri
Tauri is build using Rust. Rust is a programming language and Tauri is a JavaScript front-end lightweight GUI framework.
Tauri comes with the cross-platform application window creation library TAO and Webview rendering library WRY. Tauri v2 includes mobile iOS and Android support.
Combine HTML5 code with Tauri software framework to create a GUI desktop application. Tauri requires basic understanding of JavaScript, HTML and CSS.
HTML5 GUI Window For Desktop Application
Tauri Requirements
Name | Description | Version |
---|---|---|
Windows | Desktop operating system | 7+ |
macOS | Desktop operating system | 10.15+ |
Linux | Desktop operating system | webkit2gtk 4.0+ |
iOS | Mobile operating system | 9+ |
Android | Mobile operating system | 7+ |
Name | Description | Version |
Tauri Node.js Snippet
npm create tauri-app@latest cd tauri-app npm install npm run tauri dev
HTML Code Snippet
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <link rel="stylesheet" href="styles.css" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Tauri Name App</title> <script type="module" src="/main.js" defer></script> </head> <body> <div class="container"> <h1>HTML5 Desktop App</h1> <form class="row" id="name-age-form"> <input class="name-input" type="text" placeholder="Please enter your name" required /> <input class="age-input" type="number" placeholder="Please enter your age" required /> <button type="submit">Check</button> </form> <p id="output-msg">Output</p> </div> </body> </html>
JavaScript Code Snippet
const { invoke } = window.__TAURI__.tauri; let nameInputEl; let ageInputEl; let outputMsgEl; async function nameage() { outputMsgEl.textContent = await invoke("nameage", { name: nameInputEl.value, age: Number(ageInputEl.value) }); } window.addEventListener("DOMContentLoaded", () => { nameInputEl = document.querySelector(".name-input"); ageInputEl = document.querySelector(".age-input"); outputMsgEl = document.querySelector("#output-msg"); document.querySelector("#name-age-form").addEventListener("submit", (e) => { e.preventDefault(); nameage(); }); });
Rust Code Snippet
// Prevents additional console window on Windows in release, DO NOT REMOVE!! #![cfg_attr(not(debug_assertions), windows_subsystem = "windows")] // Learn more about Tauri commands at https://tauri.app/v1/guides/features/command #[tauri::command] fn nameage(name: &str, age: i32) -> String { format!("Hello, {0}, I see that you are {1} years old", name, age) } fn main() { tauri::Builder::default() .invoke_handler(tauri::generate_handler![nameage]) .run(tauri::generate_context!()) .expect("error while running tauri application"); }
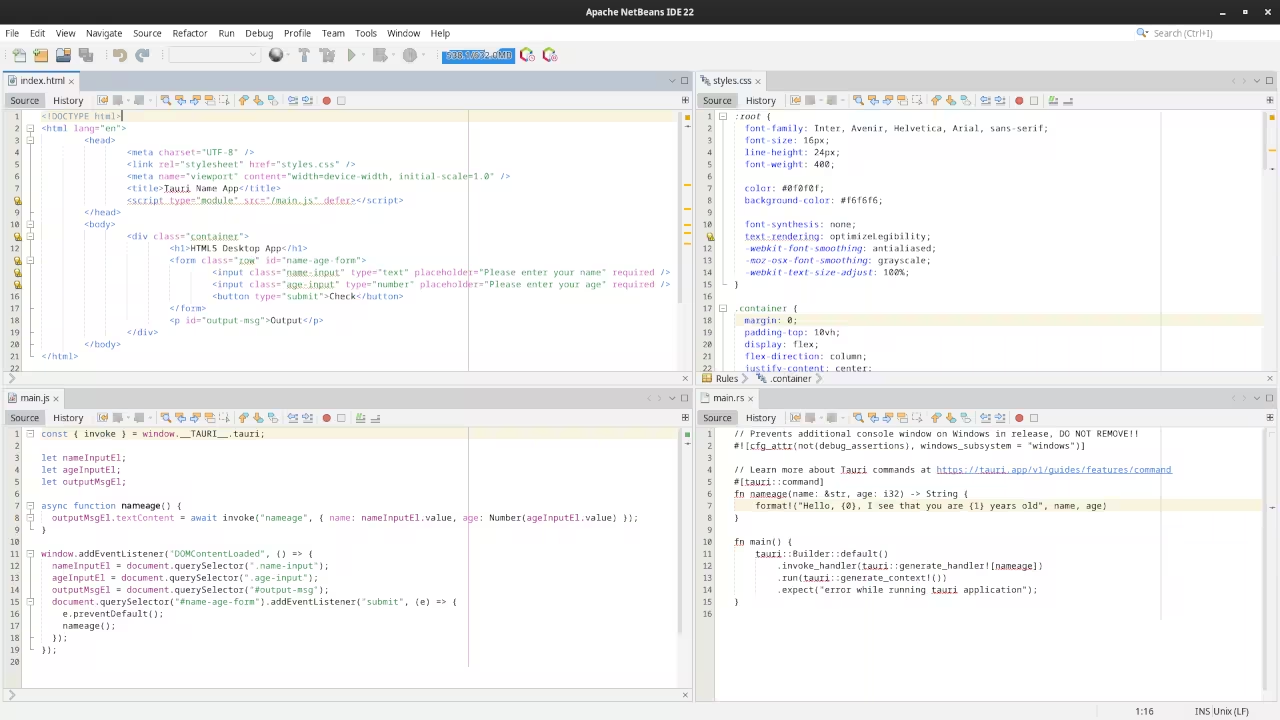
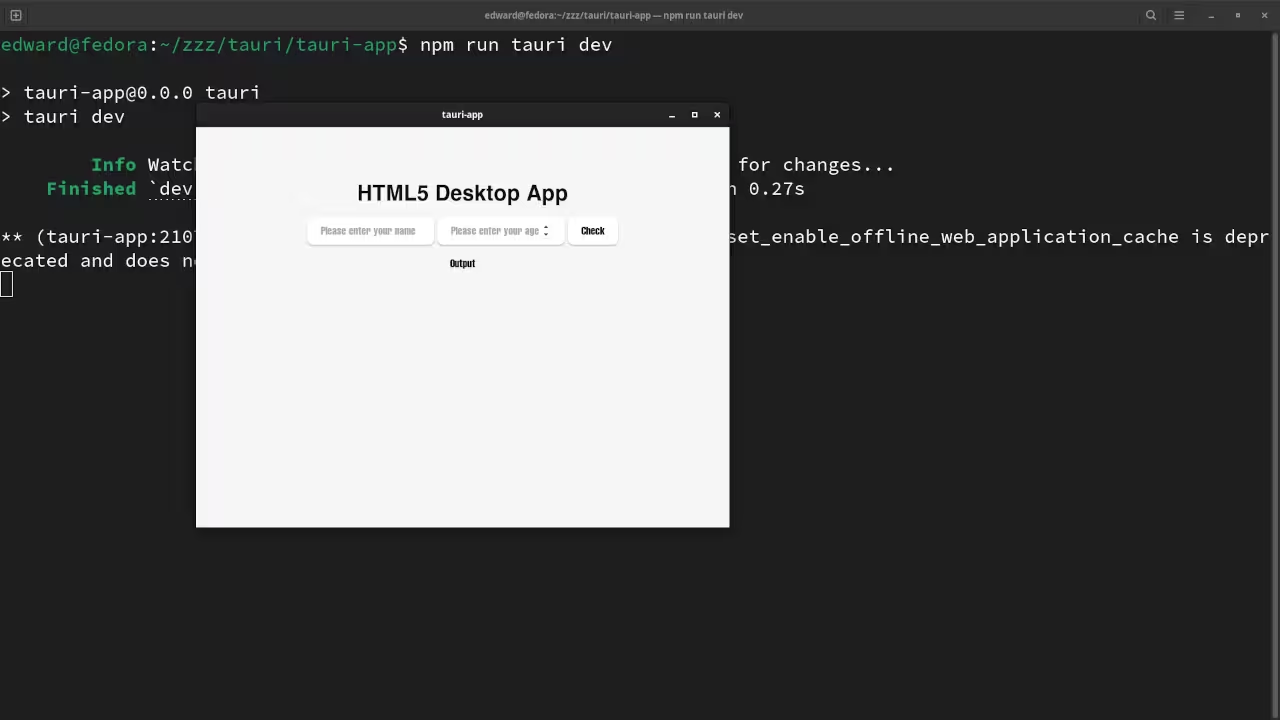
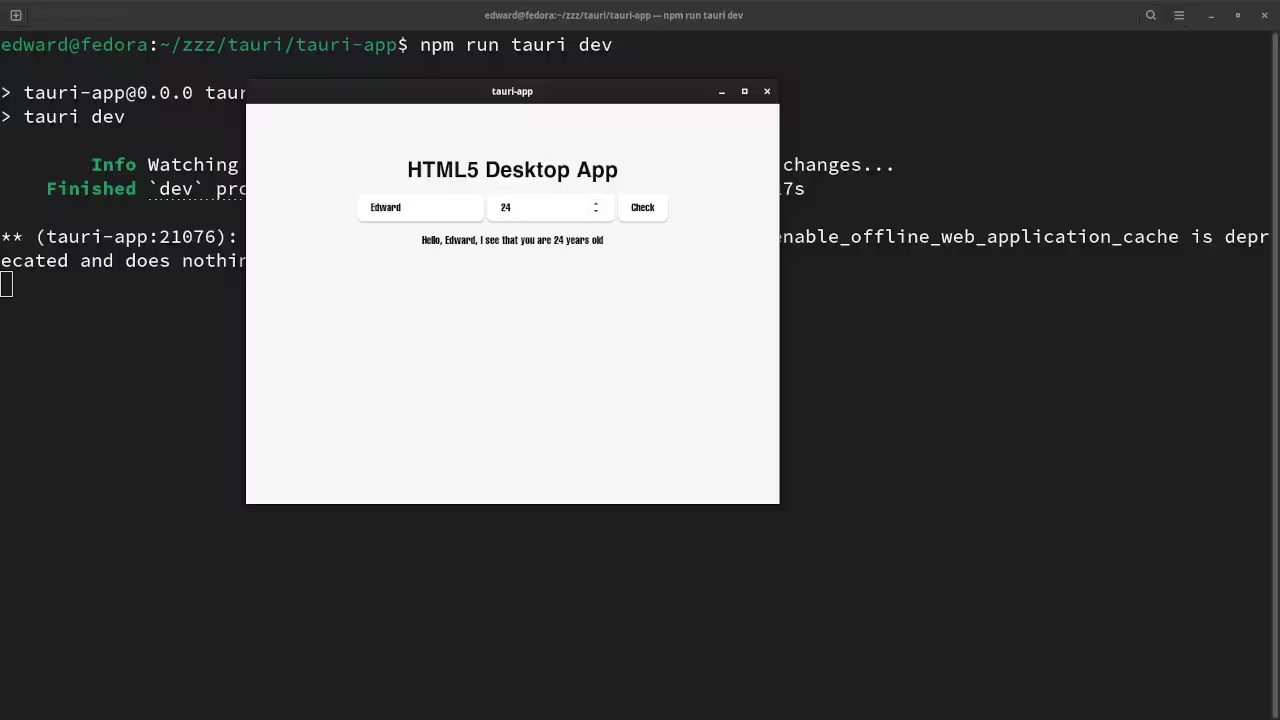
Usage
You can use any IDE or text editor and the command line to generate an application using Tauri. In this tutorial, HTML5 was used to create a desktop application for Linux.
Open Source
Tauri is is licensed under the permissive MIT License and the permissive Apache License version 2.0. This allows commercial use, modification, distribution, and allows making private derivatives. The MIT License was drafted before software patents were recognized under US Law. The Apache License includes an explicit contributor’s patent license.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
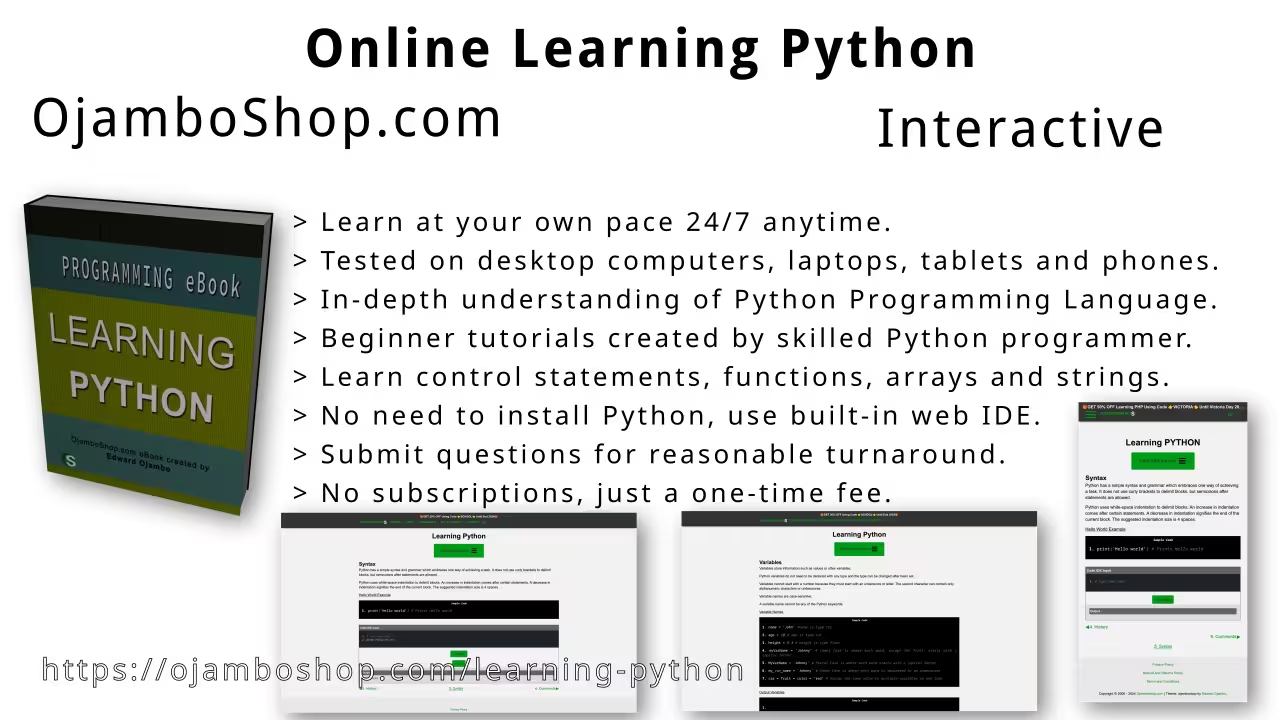
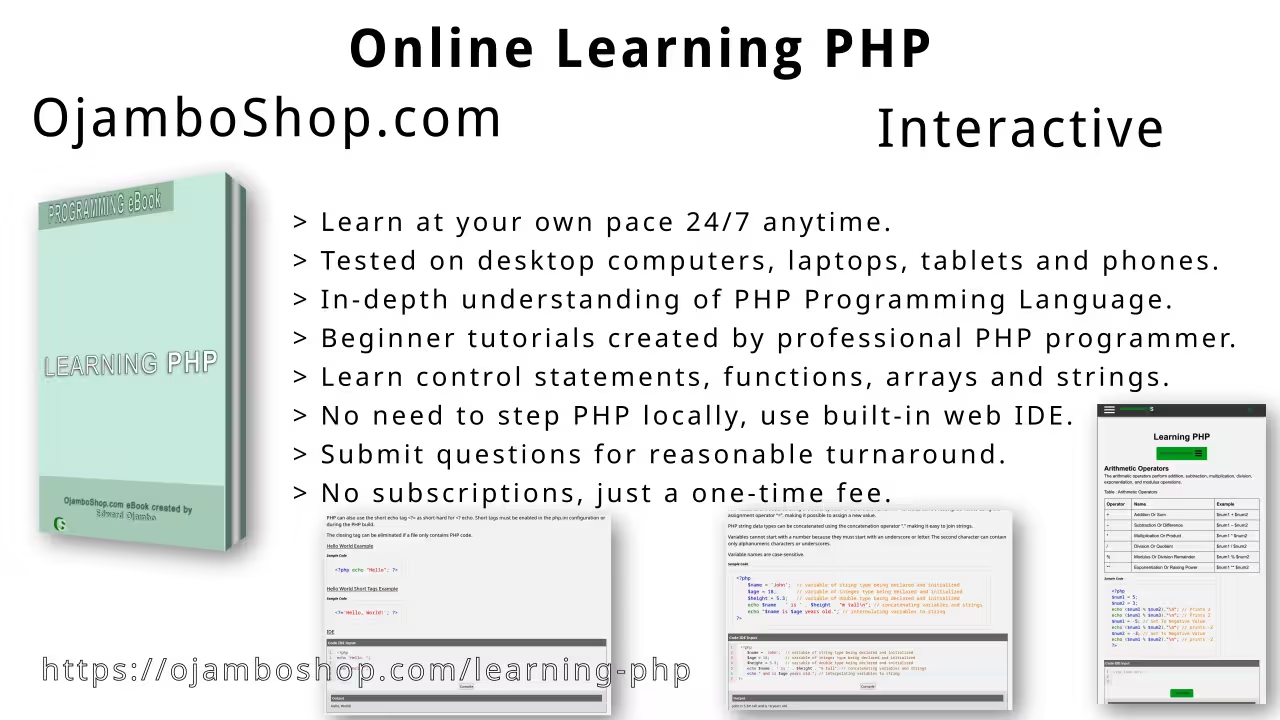
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python Course or for Learning PHP Course until End Day 2024.
Conclusion:
Python makes it easy to create a GUI for command line services or code. Command line ouput is controlled by the subprocess module and the GUI is generated using Tkinter.
Take this opportunity to learn the Python or PHP programming language by making a one-time purchase at Learning Python Course or Learning PHP Course. A web browser is the only thing needed to learn Python or PHP in 2024 at your leisure. All the developer tools are provided right in your web browser.
References:
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Tauri Software Framework
- TAO Window Creation Library
- WRY Webview Rendering Library