A Stable Guide To Camera2 API
The Android Camera2 API was introduced in Android API level 21 corresponding to Android 5.0 Lollipop. Camera2 API introduced new features to control, capture and manipulate captured images.
Camera2 API offers developers more control over the camera hardware, allowing for fine-grained adjustments such as shutter speed (ISO), autofocus, and RAW capture. Camera2 API Reference provides detailed documentation.
For this tutorial, Camera2 API will be used to place the camera in a TextureView. This is part of the ongoing Chicken Webcam App series.
This is a native Java Android application, the HTML5 application that runs in a web browser. Java is a cross-platform, statically typed, general-purpose high-level programming language with type inference.
Requirements For Native Android Application
Glossary:
IP
Internet Protocol address is a numerical label that is assigned to a device connected to a computer network.
Wi-Fi
Wireless network protocols based on the IEEE 802.11 standards for local area networks.
WLAN
Wireless LAN is a computer network that links 2 or more devices.
TCP/IP
Transmission Control Protocol/Internet Protocol is a framework for computer networking.
USB
Universal Serial Bus is a standard for digital data transmission and power delivery between electronics.
ADB
Android Debug Bridge.
API
Application Programming Interface is a set of rules or protocols that enables software applications to communicate with each other to exchange data, features, and functionality.
UI
User Interface is the point of interaction between humans and machines, allowing effective operation and control of the machine from the human end.
TextureView
Android component used for rendering video and graphics into the Android UI hierarchy.
Android Devices
Name | Description | Recording | Power |
---|---|---|---|
Sony Xperia XA1 Ultra | Updated to Android 8.0 and latest web browser. | Takes photos and videos on front and back cameras. | USB Type-C 2.0 10W charging |
Samsung Galaxy S21 FE 5G | Updated to Android 14.0 and default camera application. | Takes photos and videos on front and back cameras. | USB Type-C 2.0 <25W charging |
Logitech HD Webcam C525 | External webcam connected to workstation via USB cable. | Takes audio, photos and videos on front camera. | USB 2.0 <2.5W draw |
Name | Description | Example |
Open Camera
CameraManager manager = (CameraManager) getSystemService(Context.CAMERA_SERVICE); Log.e(TAG, "is camera open"); try { cameraId = manager.getCameraIdList()[0]; CameraCharacteristics characteristics = manager.getCameraCharacteristics(cameraId); StreamConfigurationMap map = characteristics.get(CameraCharacteristics.SCALER_STREAM_CONFIGURATION_MAP); assert map != null; imageDimension = map.getOutputSizes(SurfaceTexture.class)[0]; // Add permission for camera and let user grant the permission if (ActivityCompat.checkSelfPermission(this, Manifest.permission.CAMERA) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION); return; } manager.openCamera(cameraId, stateCallback, null); } catch (CameraAccessException e) { e.printStackTrace(); } Log.e(TAG, "openCamera X");
Preview Camera
if(null == cameraDevice) { Log.e(TAG, "updatePreview error, return"); } captureRequestBuilder.set(CaptureRequest.CONTROL_MODE, CameraMetadata.CONTROL_MODE_AUTO); try { cameraCaptureSessions.setRepeatingRequest(captureRequestBuilder.build(), null, mBackgroundHandler); } catch (CameraAccessException e) { e.printStackTrace(); }
Download
The Android SDK tools can be downloaded from SDK Platform Tools and installed on your workstation.
Explanation
- Use the ADB tool to connect to your Android device if applicable.
- Generate an Android Layout XML file as app -> res/xml/activity_main.xml.
- Generate an Android Manifest Permissions of for Camera as app -> manifests/AndroidManifest.xml.
- Generate Android code snippet to test camera preview.
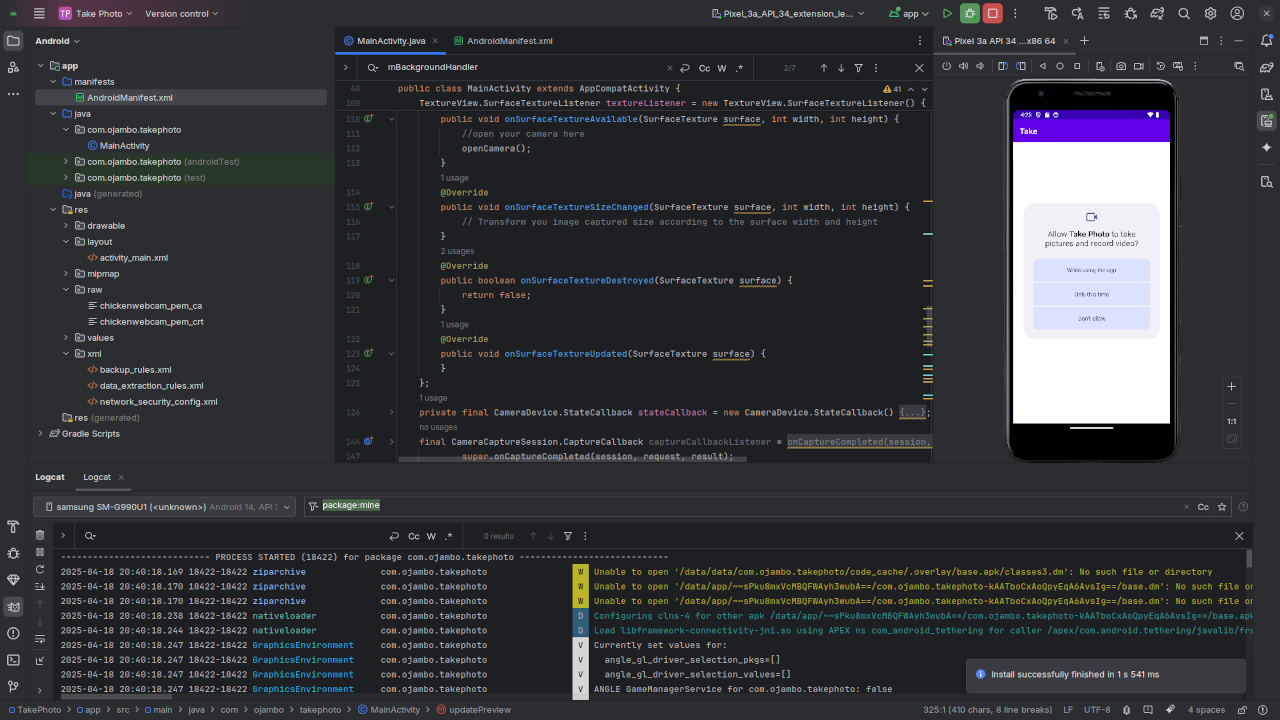
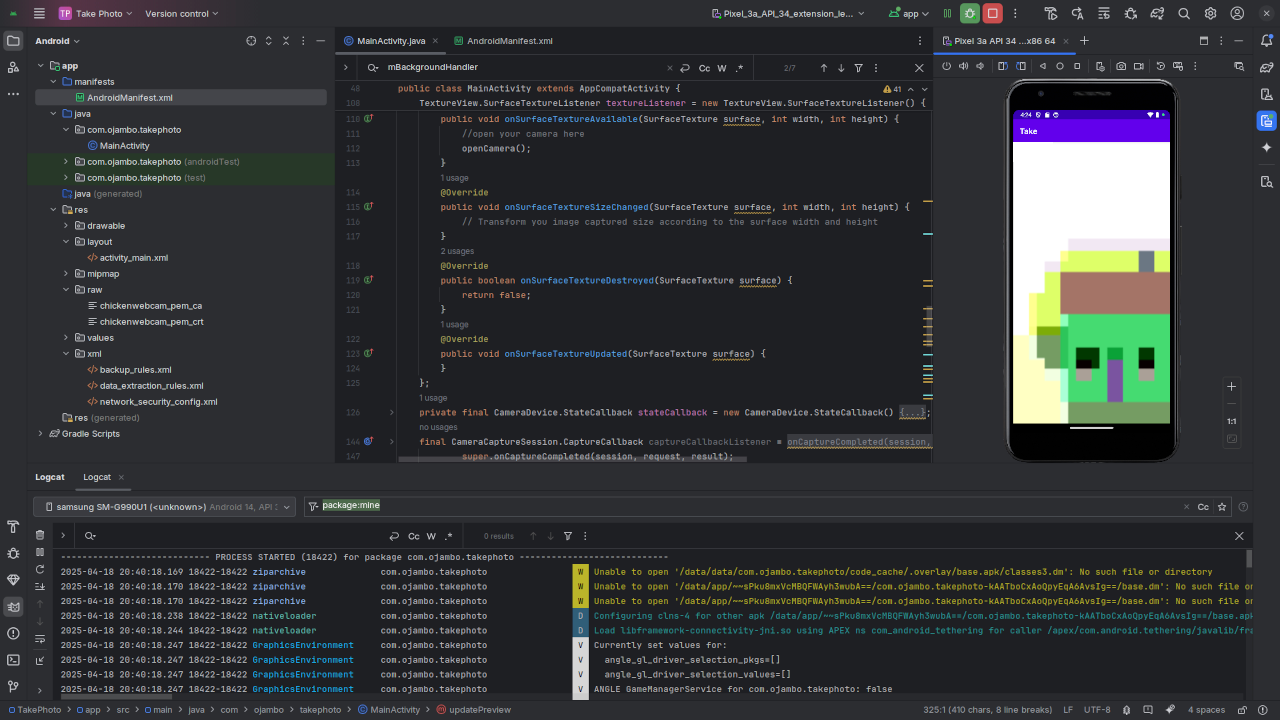
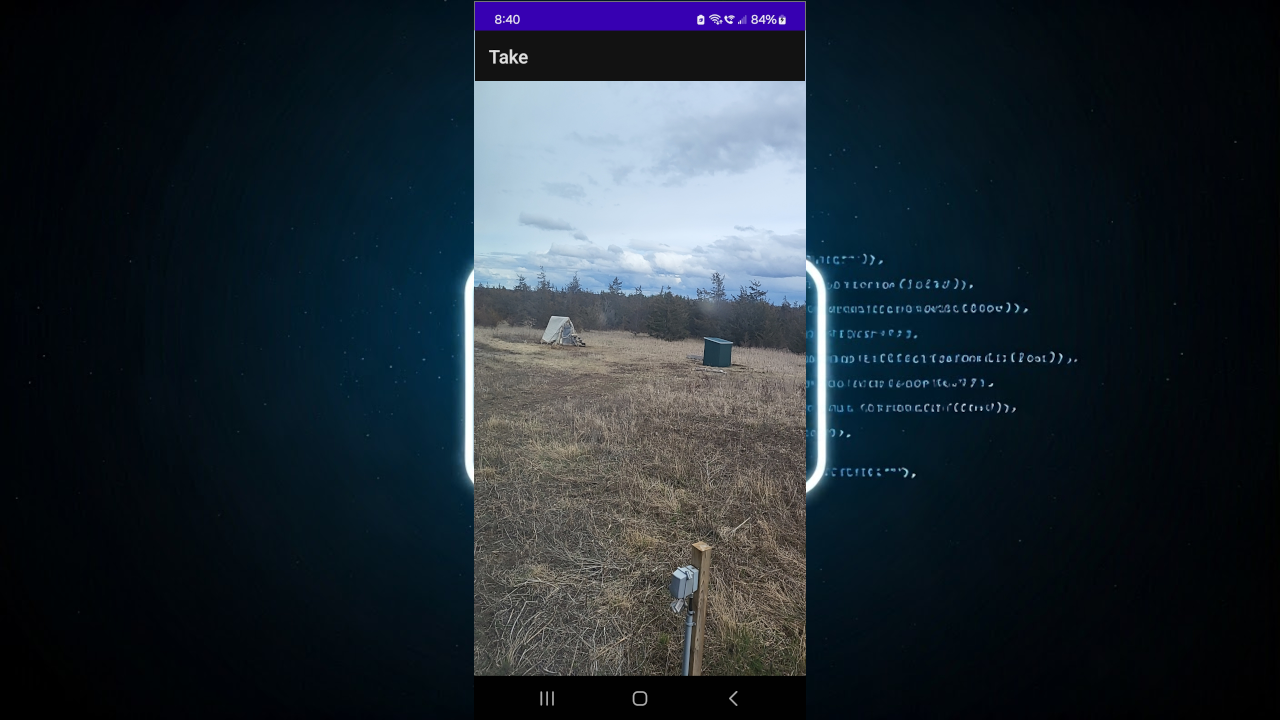
Usage
You can use any IDE or text editor to compile and execute Android code. For this tutorial, Android Studio IDE was used, but it was not needed for coding the camera preview.
Open Source
Java is dual-licensed under the GNU General Public License version 2 with classpath exception, and a proprietary license. The copyleft GPLv2 license comes with strict rules and requirements to ensure the software remains free and open-source. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
OpenJDK is licensed under the GNU General Public License version 2 with a linking exception, which prevents components that link to the Java Class Library from becoming subject to the terms of the GPL license. The copyleft GPLv2 license comes with strict rules and requirements to ensure the software remains free and open-source. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
Android is licensed under the Apache License version 2.0 for the userspace software and GNU General Public License (GPL) version 2 for the Linux kernel. The permissive license requires the preservation of the copyright notice and disclaimer, while the copyleft license comes with strict rules and requirements to ensure the software remains free and open-source. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
Conclusion:
The Android Camera2 API can be used by Java to preview the camera. The API is supported in Android versions starting from 5.0.
You can use an emulator to test the application but a real device is recommended via USB or WiFi connection for debugging.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books or simply donate here Ojambo.com Donate
References:
- Downloads & Installation Instructions For PHP
- SDK Platform Tools For Android
- Customer Sets Price Plugin for WooCommerce on Ojambo.com
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials