A Guide To Provide Secure Communication
OpenSSL is a software suite that provides secure communications over computer networks. To enable SSL for LAN uploads on an Android device, the upload server needs to support SSL. HTTPS/SSL Certificates that are self-signed can be use on the upload server and trusted in the LAN via the router, web browser of client application.
For Android projects in the Android Studio IDE, self-signed certificates can be packaged and used as a keystore for both a LAN use and remote server use.
For this tutorial, OpenSSL will be used to generate an SSL Certificate that can work for both a local domain name and IP address. The self-signed SSL certificate will be copied to the Android project for use in the LAN. The ongoing Chicken Webcam App that accessed HTML5 Camera permissions requires an SSL Certificate. Migrating to a native Android application also requires an SSL Certificate for the file upload process.
Next, you need to navigate to the Android device Google Chrome web browser and enter the URL and port number. For this tutorial, the port number is original set by the PHP built-in web server. PHP also receives the images at a specified interval. PHP can be replaced with another HTTP web server and framework for handling image uploads. JavaScript is used for the client side of the application
An SSL Certificate for requests will not be needed since the URL will contain localhost and a port number.
Requirements For Locally Hosted PWA
Glossary:
IP
Internet Protocol address is a numerical label that is assigned to a device connected to a computer network.
Wi-Fi
Wireless network protocols based on the IEEE 802.11 standards for local area networks.
WLAN
Wireless LAN is a computer network that links 2 or more devices.
PWA
Progressive Web App is built using web platforms to provide platform-specific experiences.
SSL
Secure Sockets Layer is an encryption security protocol.
TLS
Transport Layer Security is a cryptographic protocol for secure communication over a network.
HTTPS
Hypertext Transfer Protocol Secure is an extension of the Hypertext Transfer Protocol (HTTP).
SSL Certificate
Digital certificate that authenticates a website’s identity and enables an encrypted connection between the web server and the browser.
CA
Certificate Authority or Certification Authority is an entity that stores, signs, and issues digital certificates.
Android Devices
Name | Description | Recording | Power |
---|---|---|---|
Sony Xperia XA1 Ultra | Updated to Android 8.0 and latest web browser. | Takes photos and videos on front and back cameras. | USB Type-C 2.0 10W charging |
Samsung Galaxy S21 FE 5G | Updated to Android 14.0 and default camera application. | Takes photos and videos on front and back cameras. | USB Type-C 2.0 <25W charging |
Name | Description | Example |
Generate Certificate Authority Configuration
# Create Certificate Authority Configuration File # cat > CA.conf << 'EOF' [ req ] prompt = no distinguished_name = req_distinguished_name [ req_distinguished_name ] C = CA ST = Localzone L = localhost O = Ojambo Show Tutorial Certificate Authority OU = Chicken Webcam CN = 192.168.1.22 emailAddress = edward@ojamboshow.local EOF
Generate Domain And IP Address Configuration
# Create Domain And IP Address Configuration FIle # cat > chickenwebcam.conf << 'EOF' [req] default_bits = 2048 distinguished_name = req_distinguished_name req_extensions = req_ext x509_extensions = v3_req prompt = no [req_distinguished_name] countryName = CA stateOrProvinceName = ON localityName = Localhost organizationName = Certificate signed by Ojambo Show commonName = 192.168.1.22 [req_ext] subjectAltName = @alt_names [v3_req] subjectAltName = @alt_names [alt_names] IP.1 = 192.168.1.22 EOF
Generate CA Private Key And CA Certificate
# Create CA Private Key And CA Certificate With 365 Day Validity # openssl req -nodes -new -x509 -keyout CA.key -out CA.crt -days 365 -config CA.conf
Generate Web Server Secret Key And CSR
# Create Web Server Secret Key And CSR # openssl req -sha256 -nodes -newkey rsa:2048 -keyout chickenwebcam.key -out chickenwebcam.csr -config chickenwebcam.conf
Generate Certificate And Sign
# Create Certificate And Sign It Via Own Certificate Authority With 90 Day Validity # openssl x509 -req -days 90 -in chickenwebcam.csr -CA CA.crt -CAkey CA.key -CAcreateserial -out chickenwebcam.crt -extensions req_ext -extfile chickenwebcam.conf
Optionally Generate PEM Format
# Optional Convert to PEM Format # openssl x509 -in CA.crt -out chickenwebcam_pem_ca openssl x509 -in chickenwebcam.crt -out chickenwebcam_pem_crt
Optionally Run PHP Web Server And Stunnel For SSL
# PHP Built-in Web Server And Stunnel # cat chickenwebcam.crt chickenwebcam.key > stunnel.pem cat > stunnel.conf << 'EOF' [https] accept = 192.168.1.22:8443 connect = 192.168.1.22:8000 key = chickenwebcam.key cert = chickenwebcam.crt CAfile = CA.crt EOF stunnel stunnel.conf & php -S 192.168.1.22:8000
Generate Android Project Network Security Configuration
<?xml version="1.0" encoding="utf-8"?> <network-security-config> <base-config> <trust-anchors> <certificates src="system" /> <certificates src="user" /> </trust-anchors> </base-config> <domain-config> <domain includeSubdomains="true">192.168.1.22</domain> <trust-anchors> <certificates src="@raw/chickenwebcam_pem_ca" /> <certificates src="@raw/chickenwebcam_pem_crt" /> </trust-anchors> </domain-config> </network-security-config>
Generate Android Manifest Permissions
<uses-permission android:name="android.permission.INTERNET" />
Generate Android Project Kotlin Code
Thread(Runnable { val url = "192.168.1.22:8443/" try { val connection: URLConnection = URL(url).openConnection() connection.connect() val inputStream = connection.getInputStream() val reader = BufferedReader(InputStreamReader(inputStream)) reader.use { val content = it.readText() println(content) } } catch (e: MalformedURLException) { println("Malformed URL: ${e.message}") } catch (e: IOException) { println("IO Exception: ${e.message}") } catch (e: UnknownHostException) { println("Unknown Host: ${e.message}") } catch (e: SocketTimeoutException) { println("Socket Timeout: ${e.message}") } }).start()
Download
The OpenSSL tools can be downloaded from OpenSSL Library and installed on your workstation.
Explanation
- Generate a Certificate Authority.
- Generate a Certificate and sign it using the Certificate Authority.
- Convert the Certificate to PEM format if you are targeting older Android versions.
- Add the Certificate to the res/raw folder in the Android Project.
- Generate an Android Network Security Configuration XML file as app -> res/xml/network_security_config.xml.
- Generate an Android Manifest Permissions of for Internet as app -> manifests/AndroidManifest.xml.
- Generate Android code snippet to test URL connection.
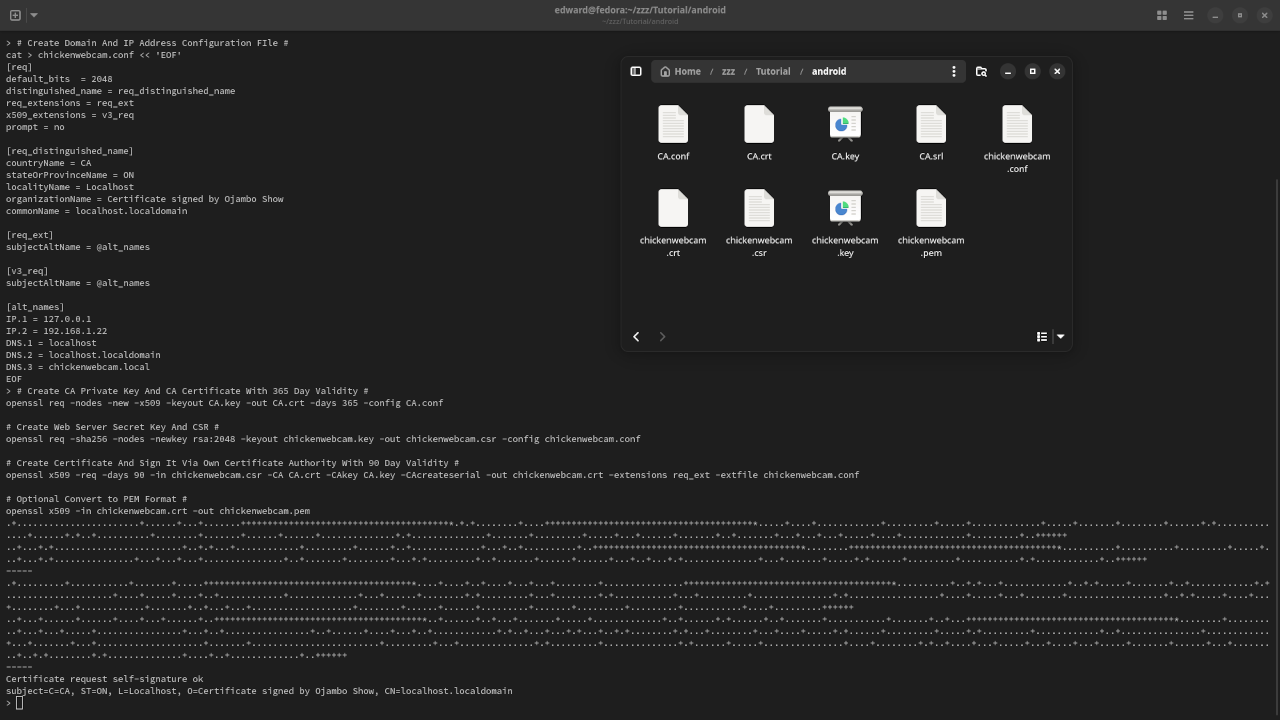
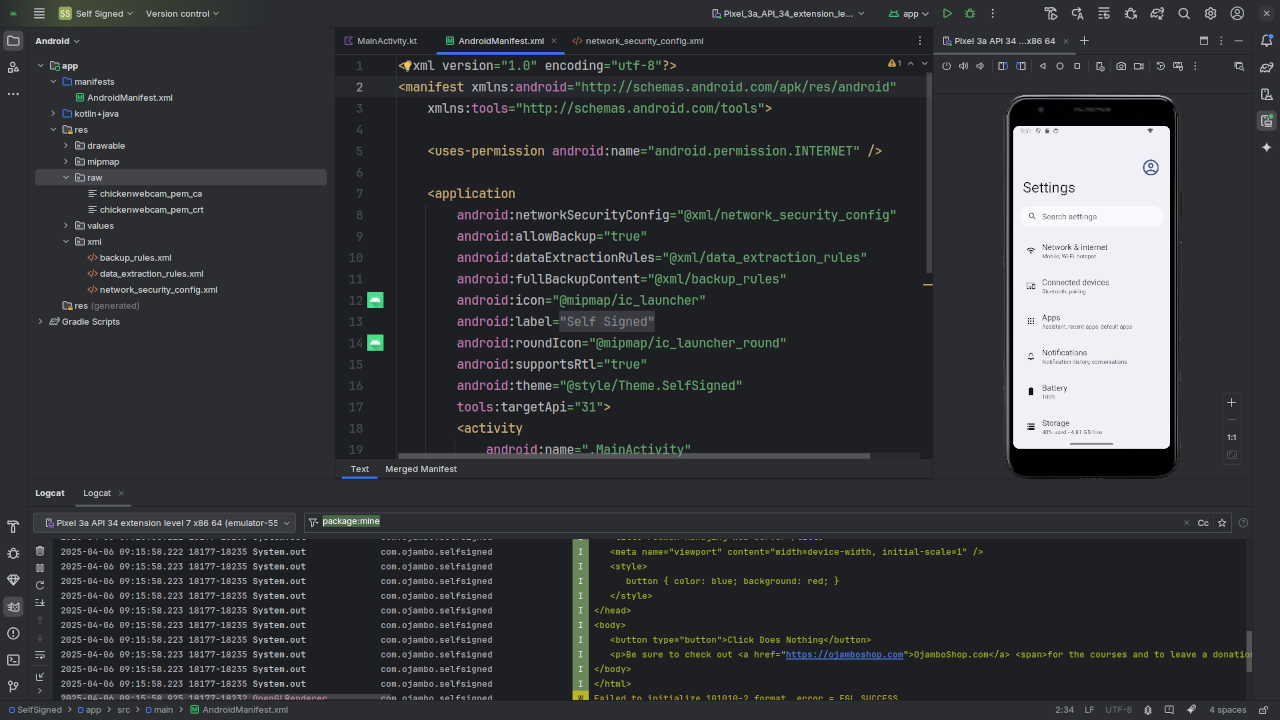
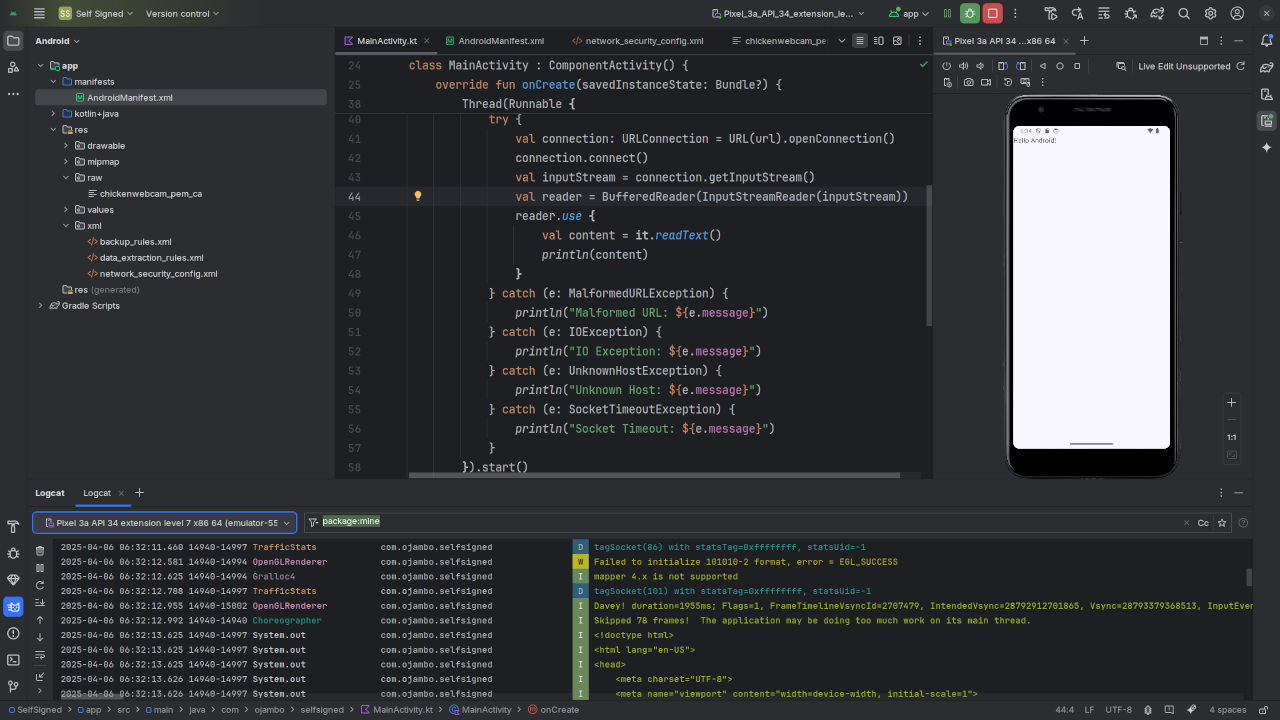
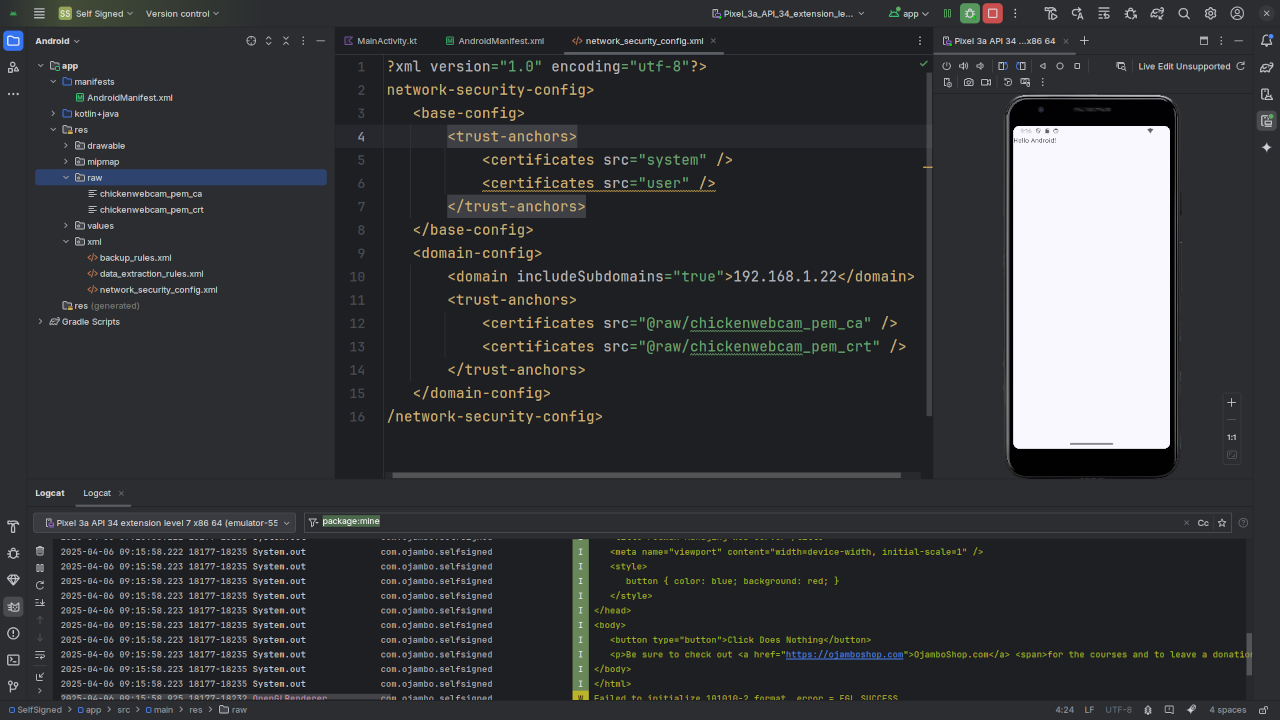
Usage
You can use any IDE or text editor to compile and execute Android code. For this tutorial, Android Studio IDE was used, but it was not needed for the SSL Certificate placement.
Open Source
OpenSSL is licensed under the Apache License 2.0. The permissive license has conditions requiring preservation of copyright and license notices. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
Stunnel is licensed under the terms of the GNU General Public License (GPL) version 2 or later with OpenSSL exception. The copyleft license comes with strict rules and requirements to ensure the software remains free and open-source. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
The PHP License is an open-source under which the PHP scripting language is released. The permissive license has conditions requiring preservation of copyright and license notices. Redistribution is permitted in source or binary form with or without modifications, consult the license for more specific details.
Kotlin is licensed under the Apache License version 2.0. The permissive license requires the preservation of the copyright notice and disclaimer. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
Android is licensed under the Apache License version 2.0 for the userspace software and GNU General Public License (GPL) version 2 for the Linux kernel. The permissive license requires the preservation of the copyright notice and disclaimer, while the copyleft license comes with strict rules and requirements to ensure the software remains free and open-source. It allows commercial use, modification, distribution, and allows making derivatives proprietary, consult the license for more specific details.
Conclusion:
An SSL Certificate is required when an Android application attempts to upload to a server. For a LAN application, a self-signed Certificate can be packaged and used in the Android application. OpenSSL can generate a Certificate Authority and the SSL Certificate signed by the CA.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books or simply donate here Ojambo.com Donate
References:
- OpenSSL Implementation Of SSL And TLS Protocols
- SDK Platform Tools For Android
- Customer Sets Price Plugin for WooCommerce on Ojambo.com
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials