Create Arrow Functions In JavaScript
In JavaScript, a function declaration defines a function with the specified parameters and code block.
Functions can be called before their creation due to hoisting. A JavaScript function is a subprogram that can be called by code externally or internally to the function. Arrow functions use the arrow symbol as a syntax for writing function expressions.
Arrow functions cannot be used as constructors or define methods within objects. Arrow functions allow for an implicit return.
Common Syntax Of Arrow Functions In JavaScript
Glossary:
Hoisting
The interpreter moves function declaration to the top of their scope.
Scope
The accessibility of variables and functions within certain parts of your code.
Declaration
Used to define variables, functions, and constants.
Method
Function defined within a object.
Arrow Functions
Syntax (=>) for creating anonymous expressions.
Closures
Access variables from outer scope even after outer function has finished.
Recursion
Method to call itself to solve a problem.
Constructor
Special method within a class used to initialize and create objects of that class.
Implicit Return
Return a value without using the return keyword.
Common Arrow Functions
Name | Description | Example |
---|---|---|
myFunc = () => {} | Arrow function. | name = () => { return “John”; } |
myFunc = () => val; | Arrow function implicit return. | name = () => “John”; |
myFunc = (val) => val; | Arrow function arguments. | name = (fname) => fname; |
Name | Description | Example |
JavaScript Arrow Functions Snippet
// OjamboShop.com Learning JavaScript Arrow Functions Tutorial // Function let namef = function() { return "John"; }; console.log("The function returned: " + namef()); // Arrow Function let namefa = () => { return "Jane"; }; console.log("The arrow function returned: " + namefa()); // Arrow Function Arguments let namefaa = (name) => { return "The arrow function with argument returned: " + name; }; console.log(namefaa('Jake')); // Arrow Function Arguments Implicit Return let namefaai = (name) => "The arrow function with argument implicit returned: " + name; console.log(namefaai('Jill'));
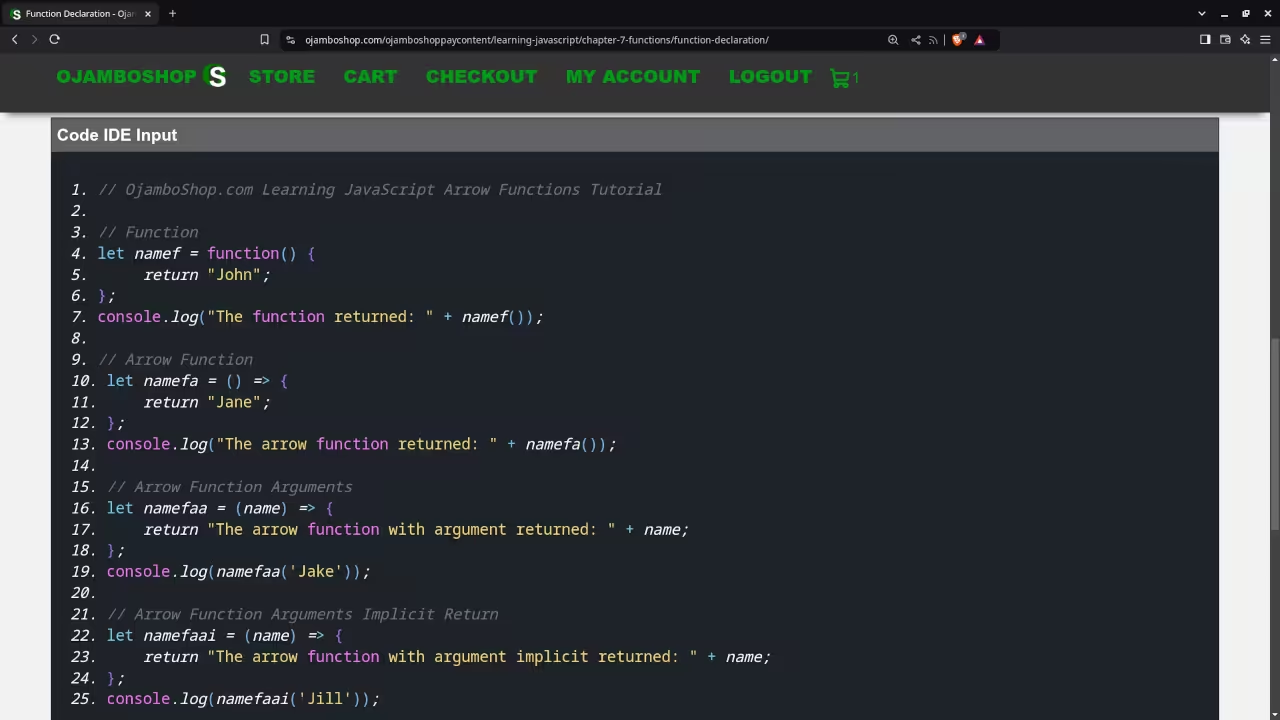
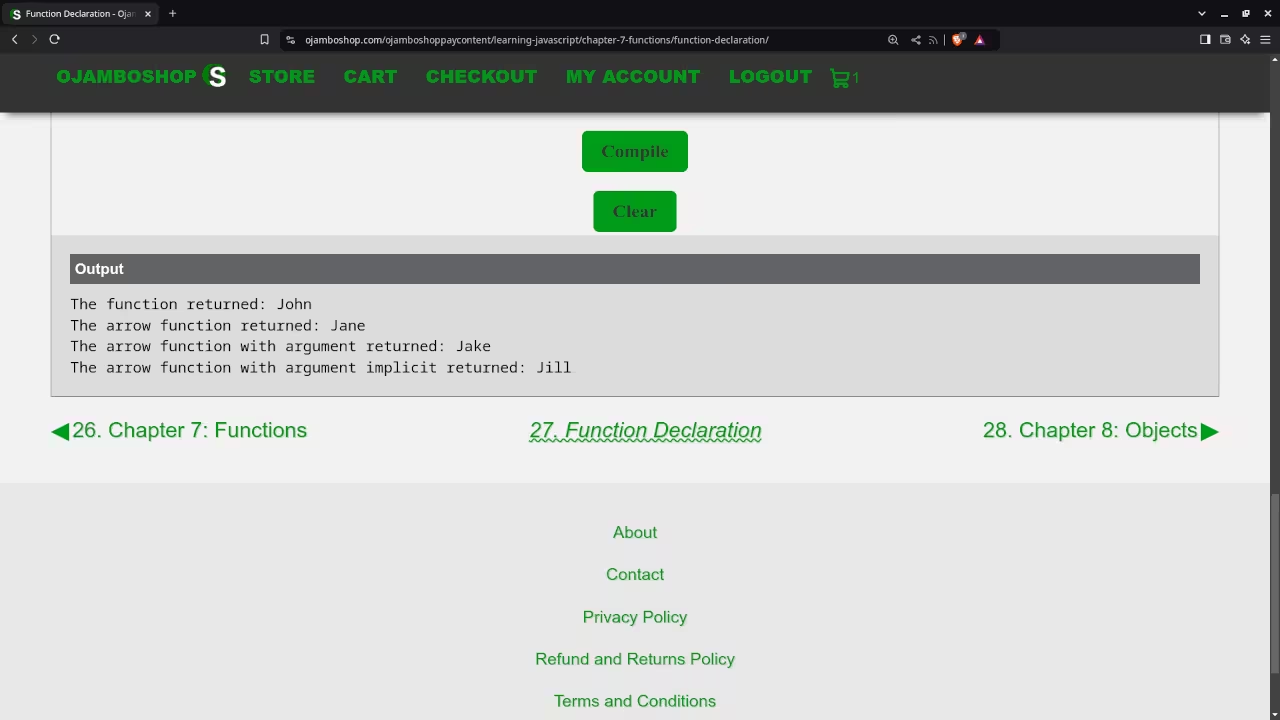
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the arrow functions.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Live Stream
Every Friday, you can join a live stream and ask questions. Check Ojambo.com for details and instructions.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
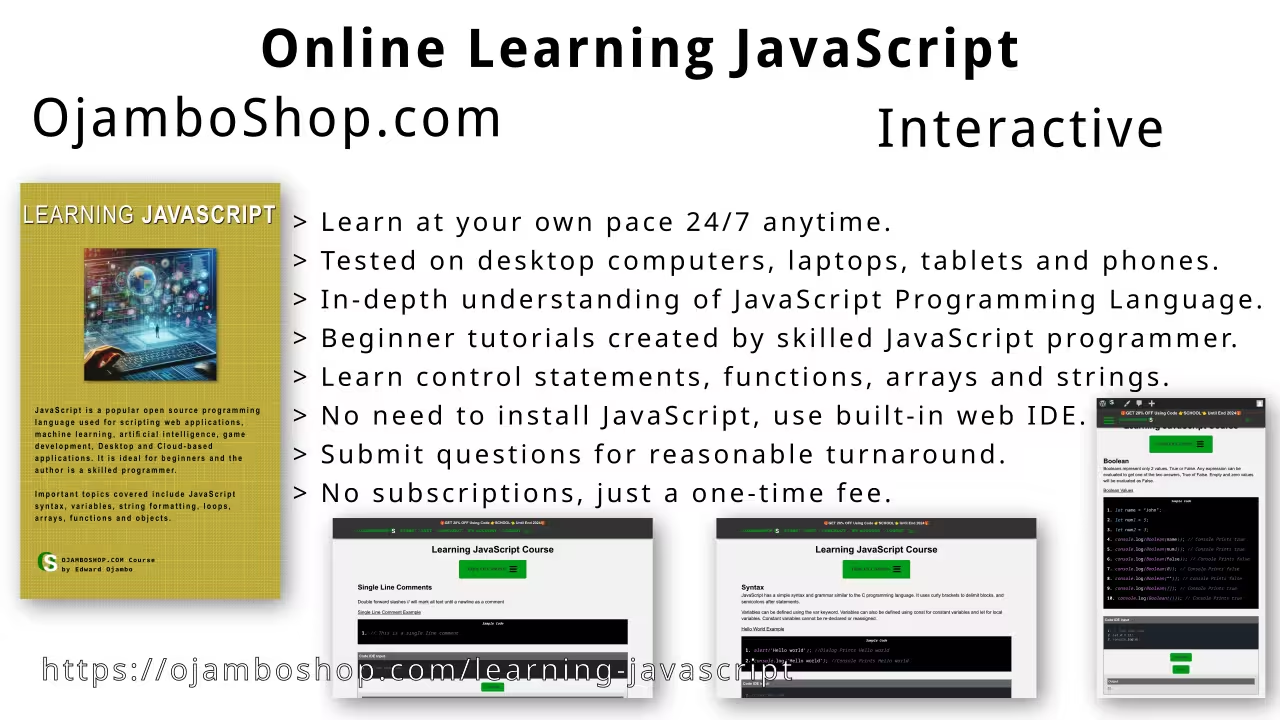
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
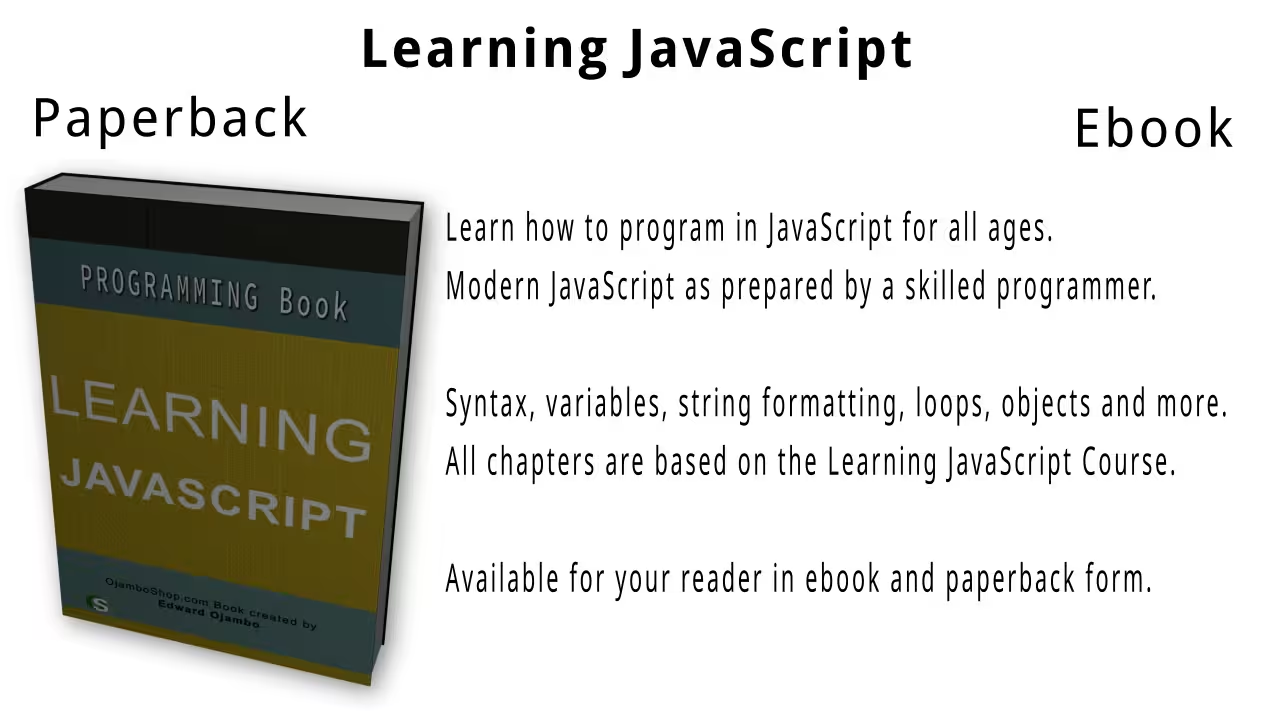
Conclusion:
JavaScript makes it easy to use create arrow functions. Arrow functions use the arrow symbol as syntax for concise function expressions.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials