Create Function Declarations In JavaScript
In JavaScript, a function declaration defines a function with the specified parameters and code block.
Functions can be called before their creation due to hoisting. A JavaScript function is a subprogram that can be called by code externally or internally to the function. Functions are reusable blocks of code designed to perform specific tasks.
JavaScript comes with built-in functions that can be called directly to perform a specific task. It is also possible to create custom functions also called user defined functions.
Common Syntax Of Function Declarations In JavaScript
Glossary:
Hoisting
The interpreter moves function declaration to the top of their scope.
Scope
The accessibility of variables and functions within certain parts of your code.
Declaration
Used to define variables, functions, and constants.
Method
Function defined within a object
Common Function Declarations
Name | Description | Example |
---|---|---|
function myFunc() | Function declaration for code block. | function myFunc() {} |
function myFunc(n) | Function declaration with argument(s) for code block. | function myFunc(num) {} |
Name | Description | Example |
JavaScript Function Declarations Snippet
// OjamboShop.com Learning JavaScript Function Declarations Tutorial function myFunc() { console.log('called myFunc'); } myFunc(); function displayName(name) { console.log('The name is ' +name); } displayName('John'); function calculateSum(num1, num2) { let sum = (num1 + num2); return sum; } console.log('The sum of 2 numbers is ' + calculateSum(5,3));
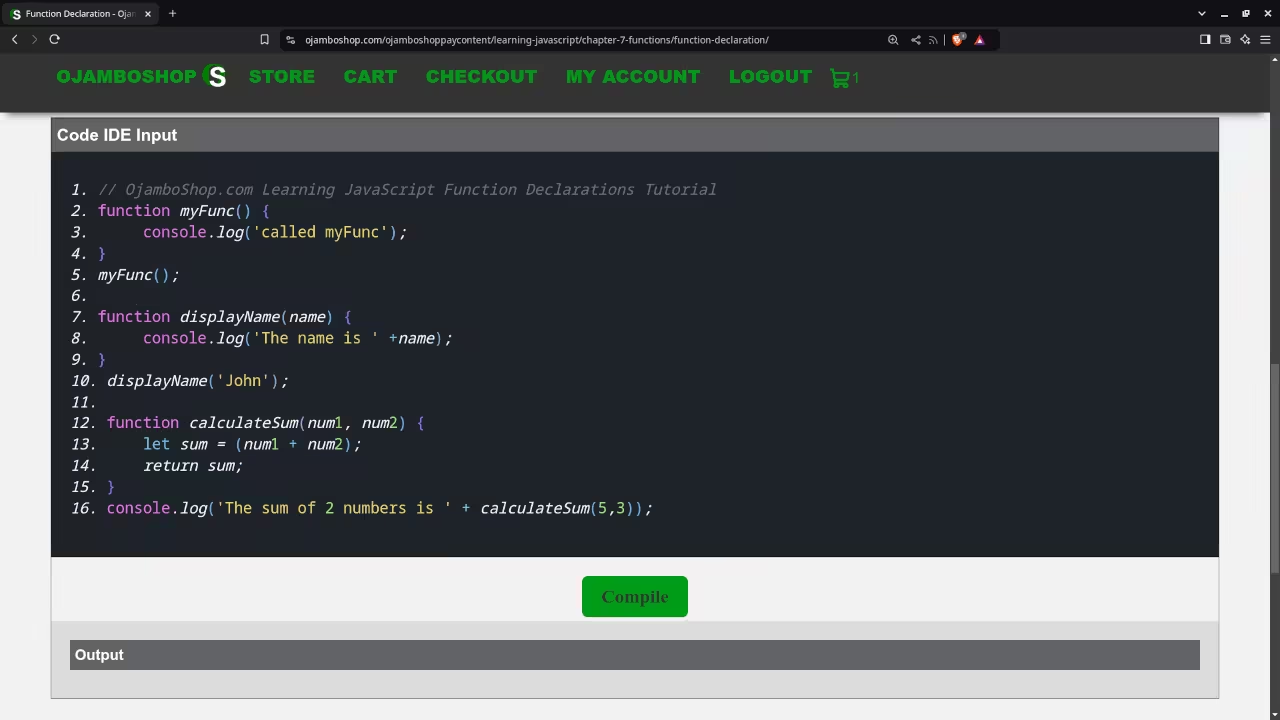
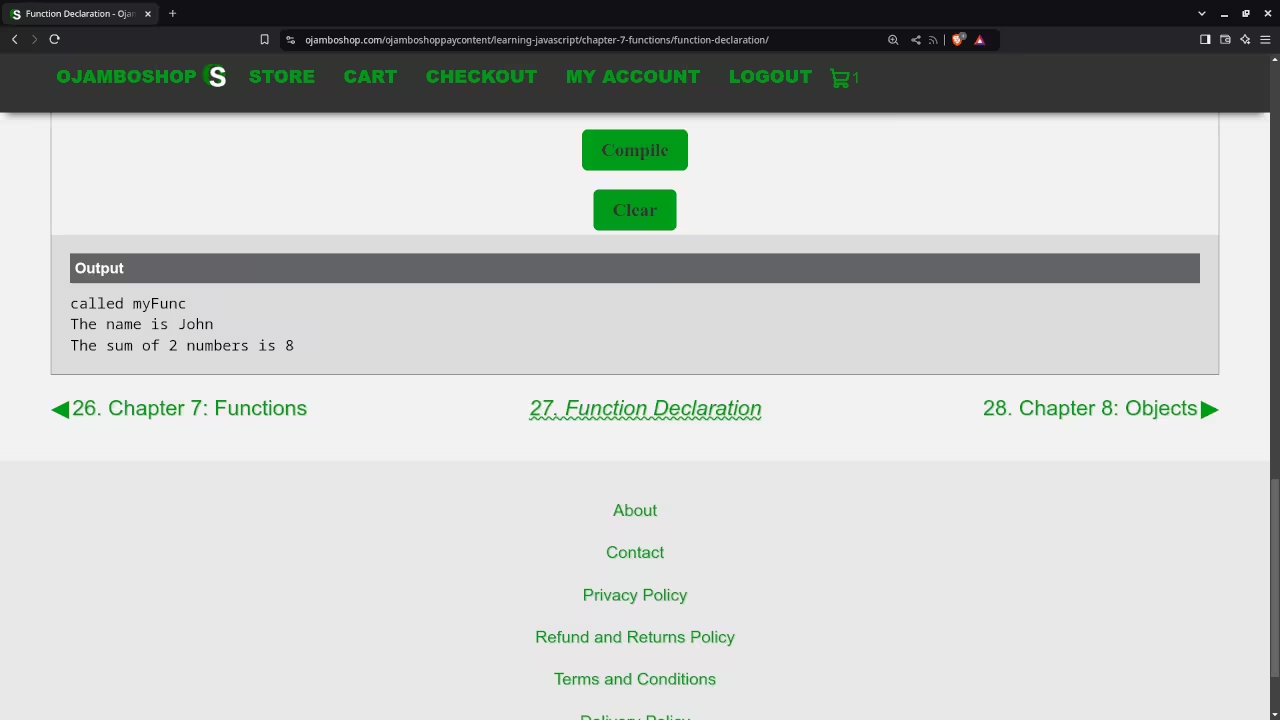
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the function declarations.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
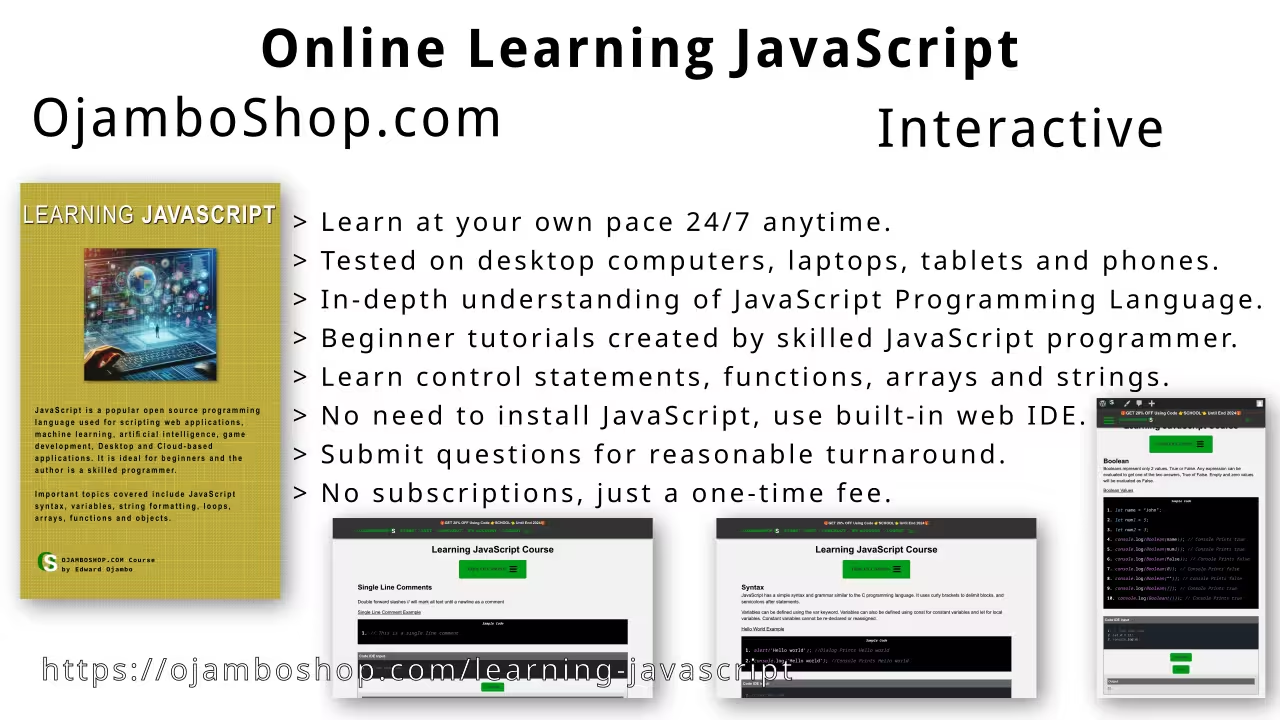
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
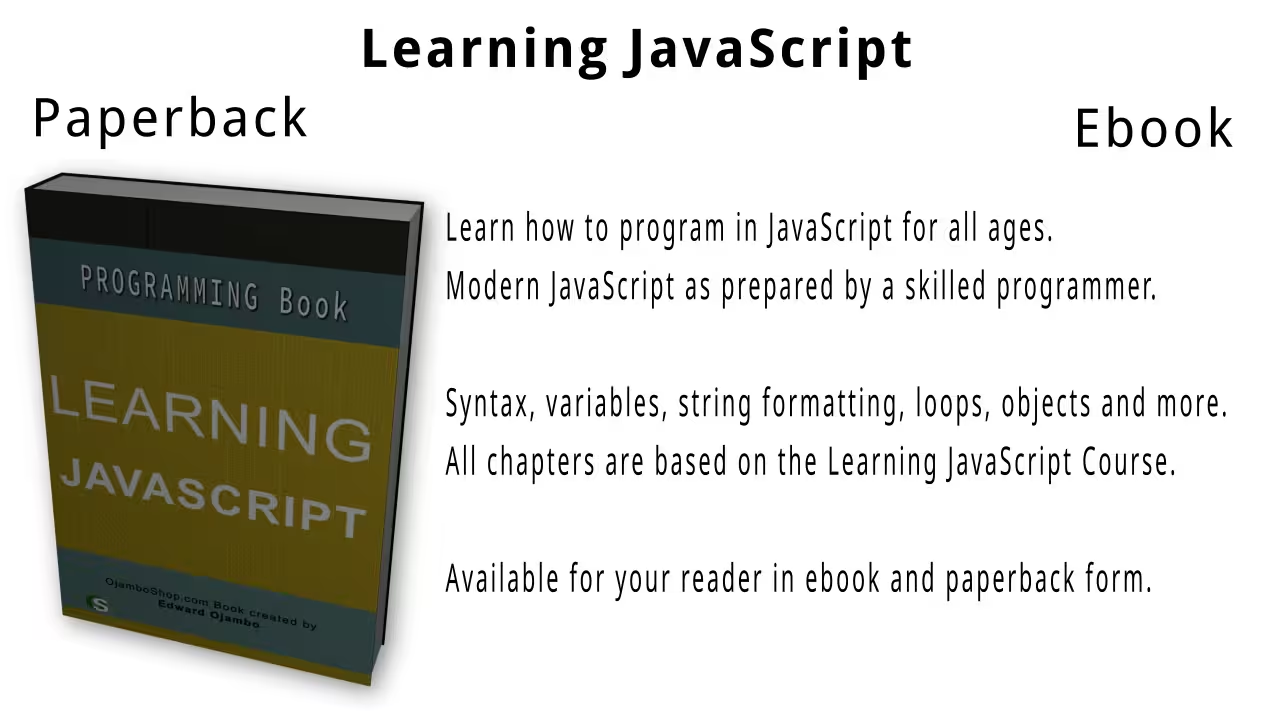
Conclusion:
JavaScript makes it easy to use create functions. Use custom functions to pass values as parameters or arguments and return a value if applicable.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials