Create Set Object Methods In JavaScript
In JavaScript, Set objects are collections of unique values.
Set objects efficiently store, retrieve, and manipulate distinct values of any data type including other sets. JavaScript has built-in methods for manipulating Set objects.
A new Set object is created via the Set constructor.
Common Syntax Of Set Object Methods In JavaScript
Glossary:
Unique
The single one of its kind
Value
The representation of some entity that can be manipulated by a program
Object
Data structure storing related data as key-value pair
Method
Function defined within a object
Common Set Object Methods
Name | Description | Example |
---|---|---|
Set() | Create an empty Set. | ages = new Set(); |
add() | Add a new element to the Set. | ages.add(55); |
clear() | Remove all elements from the Set. | ages.clear(); |
delete() | Remove an elements from the Set. | ages.delete(55); |
entries() | Return an Iterator with [value,value] pairs from the Set. | ent = ages.entries(); |
has() | Return true if value exists in the Set. | ans = ages.has(55); |
keys() | Returns an Iterator object with the values from the Set. | keys = ages.keys(); |
values() | Returns an Iterator object with the values from the Set. | keys = ages.values(); |
size | Returns number of elements from the Set. | size = ages.size; |
Name | Description | Example |
JavaScript Set Object Methods Snippet
// OjamboShop.com Learning JavaScript Set Object Methods Tutorial let names = new Set(); // Create Set let ages = new Set([23, 33, 44]); // Create & Initialize Set console.log(names.size); console.log(ages.size); names.add("John"); ages.add(55); console.log(names.size); console.log(ages.size); console.log(names.has("John")); console.log(ages.has(55)); names.forEach (function(valn) { console.log(valn); }); ages.forEach (function(vala) { console.log(vala); }); let keysn = names.keys(); let keysa = ages.values(); console.log(typeof keysn); console.log(typeof keysa); console.log(keysn); console.log(keysa); names.delete("John"); ages.delete(55); console.log(names.size); console.log(ages.size); names.clear(); ages.clear(); console.log(names.size); console.log(ages.size);
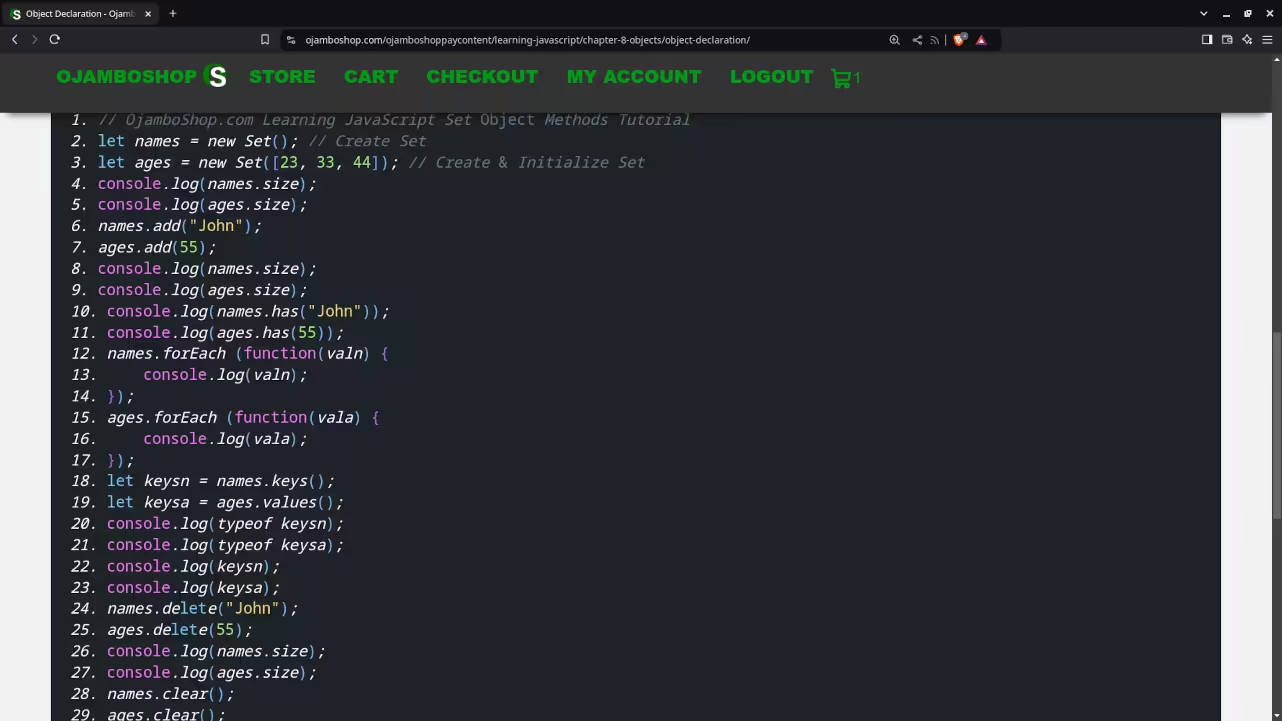
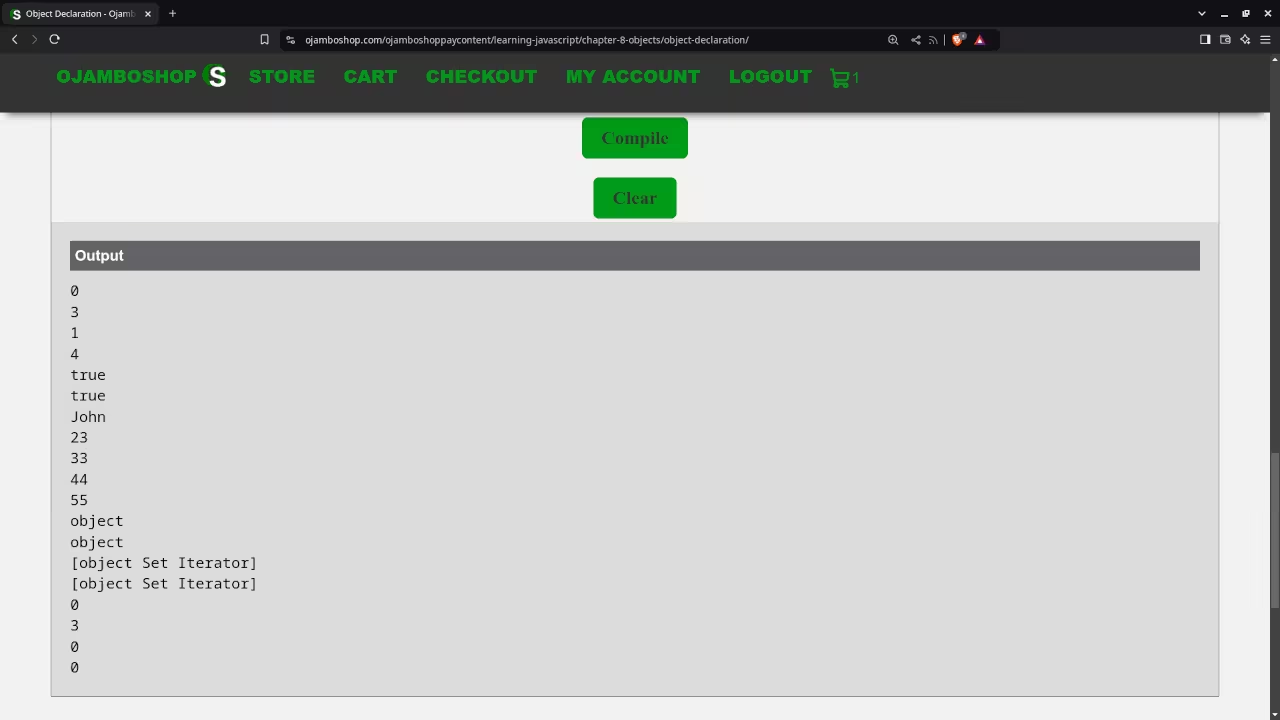
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the Set object methods.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
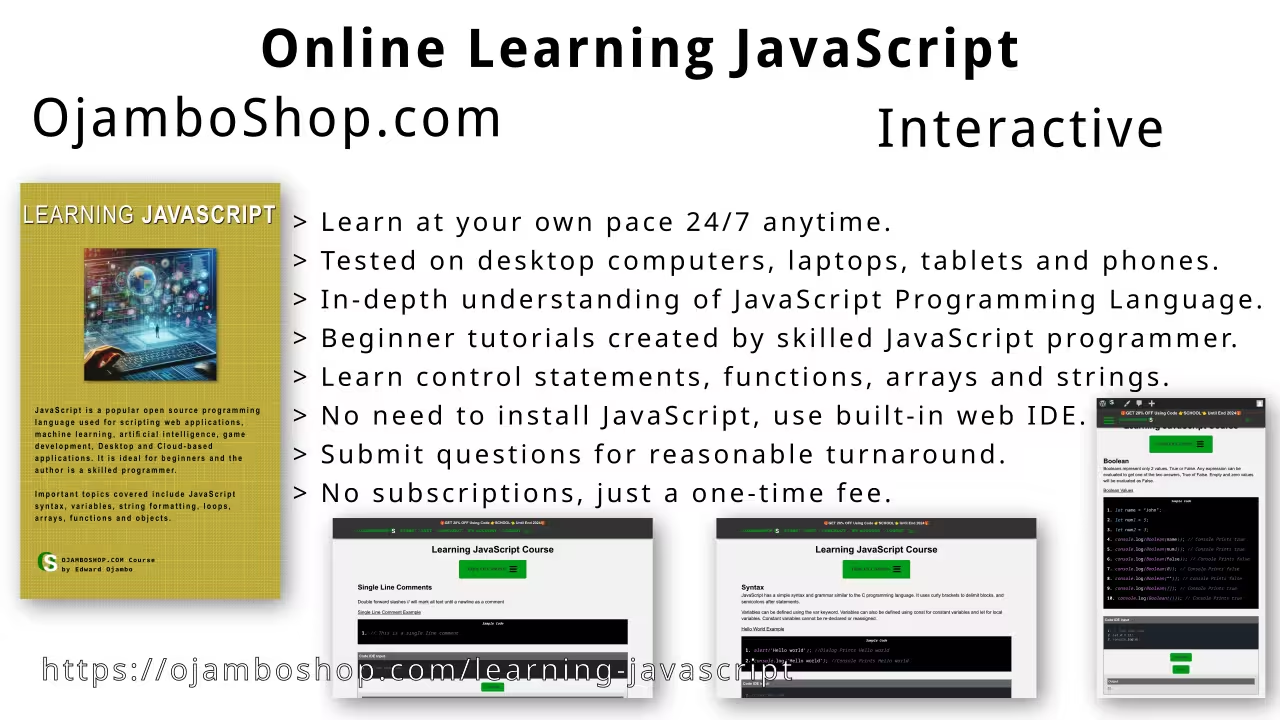
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
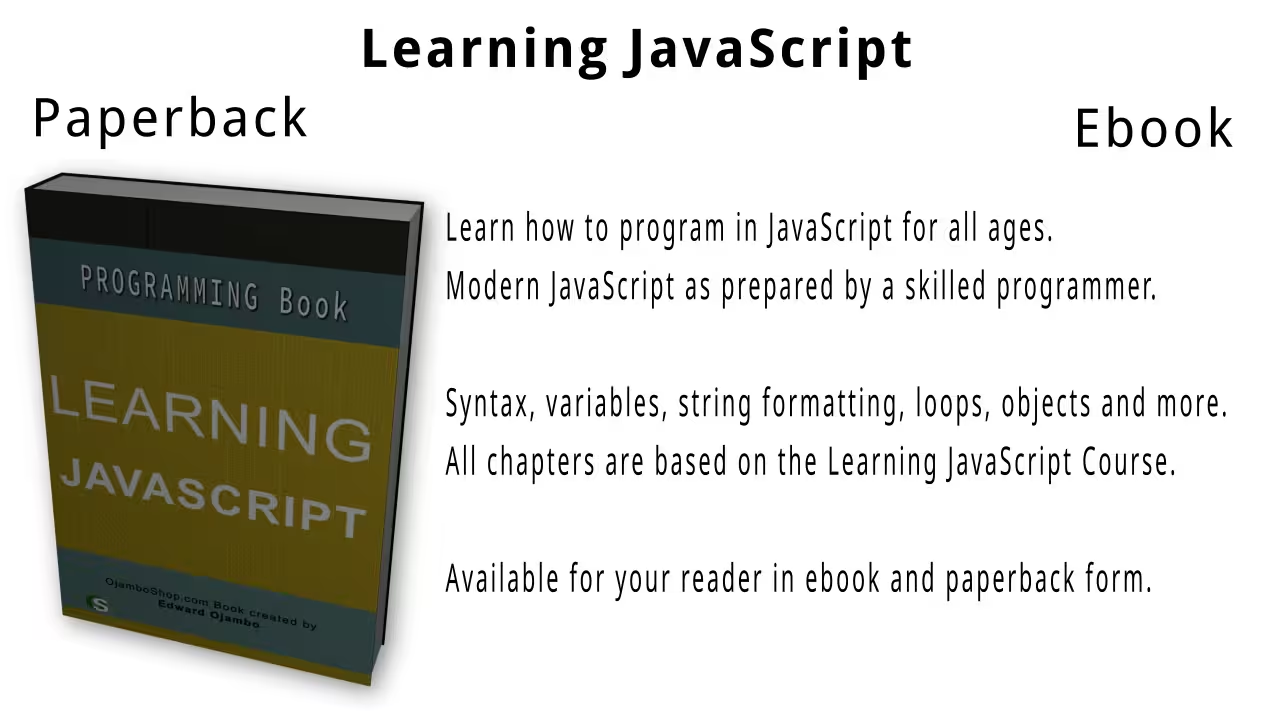
Conclusion:
JavaScript makes it easy to use the Set object methods. Use the Set object methods to handle the Set object values.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials