Create Ternary Operator In JavaScript
In JavaScript, the ternary operator is shorthand for conditional if else statements.
The ternary operator works based on a condition. It is possible to nest ternary operators.
Ternary operator can be used to quickly decided between 2 or more values depending on the condition.
Common Syntax Of Ternary Operator In JavaScript
Glossary:
Conditional
Return different values depending on Boolean expression.
Expression
Structured statement that may be evaluated to determine its value.
Boolean
Data type with only 2 possible values, true or false.
Common Ternary Operator
Name | Description | Example |
---|---|---|
condition ? IfTrue : IfFalse | Ternary operation returns expression if true or false | (age > 18) ? “Adult” : “Minor”; |
condition ? IfTrue : ElseIfTrue : IfFalse | Ternary operation nested if else if and else | (age > 18) ? “Adult” : (age > 13) ? “Teen” : “Minor”; |
Name | Description | Example |
JavaScript Ternary Operator Snippet
// OjamboShop.com Learning JavaScript Ternary Operator Tutorial let age = 15; let group = (age > 18) ? "Adult" : "Minor"; console.log(group); // Nested Ternary Operator group = (age > 18) ? "Adult" : (age > 13) ? "Teen" : "Minor"; console.log(group); age = 3; group = (age > 18) ? "Adult" : (age > 13) ? "Teen" : "Minor"; console.log(group); age = 30; group = (age > 18) ? "Adult" : (age > 13) ? "Teen" : "Minor"; console.log(group);
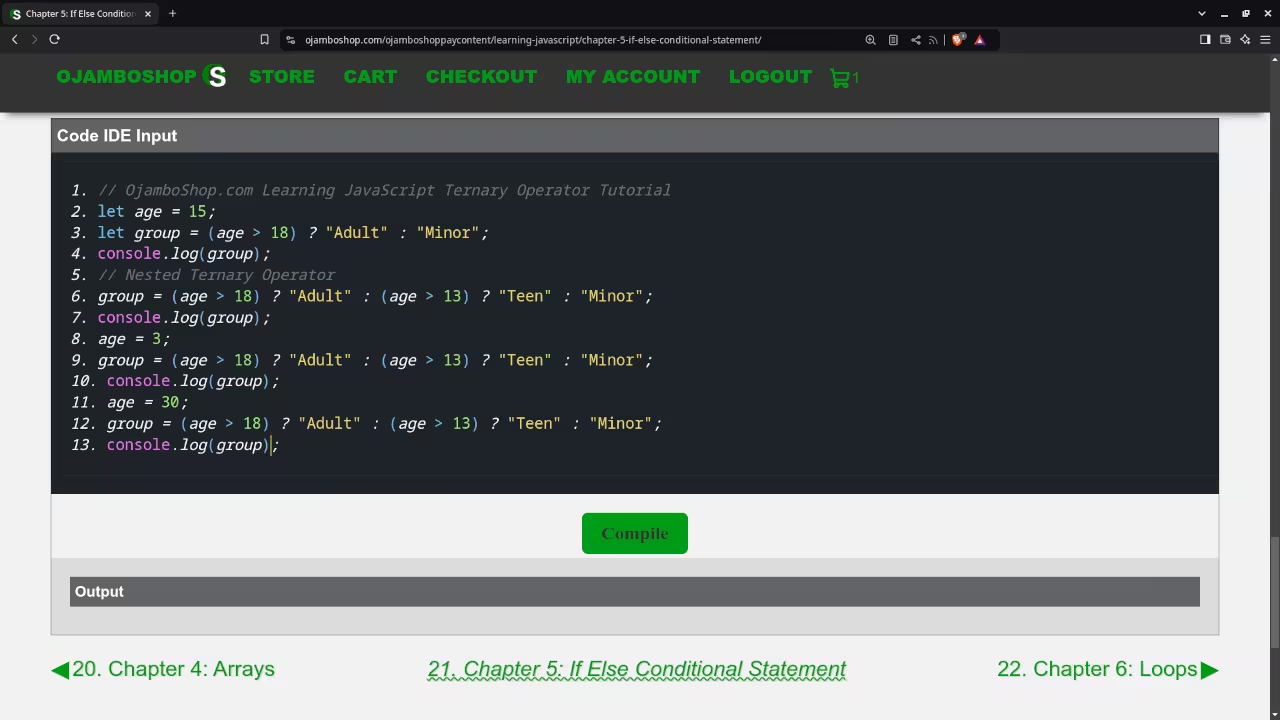
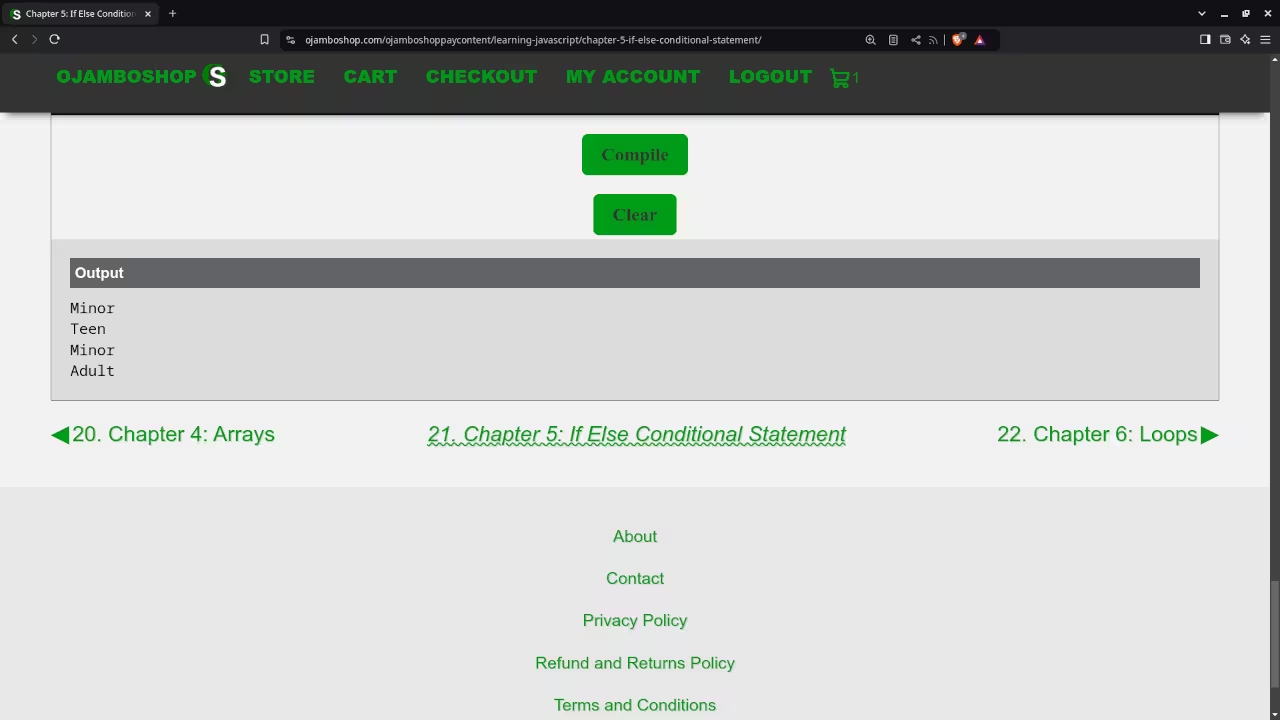
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE can used to input and compile JavaScript code for the ternary operator.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Get the Learning JavaScript Course for your web browser on any device.
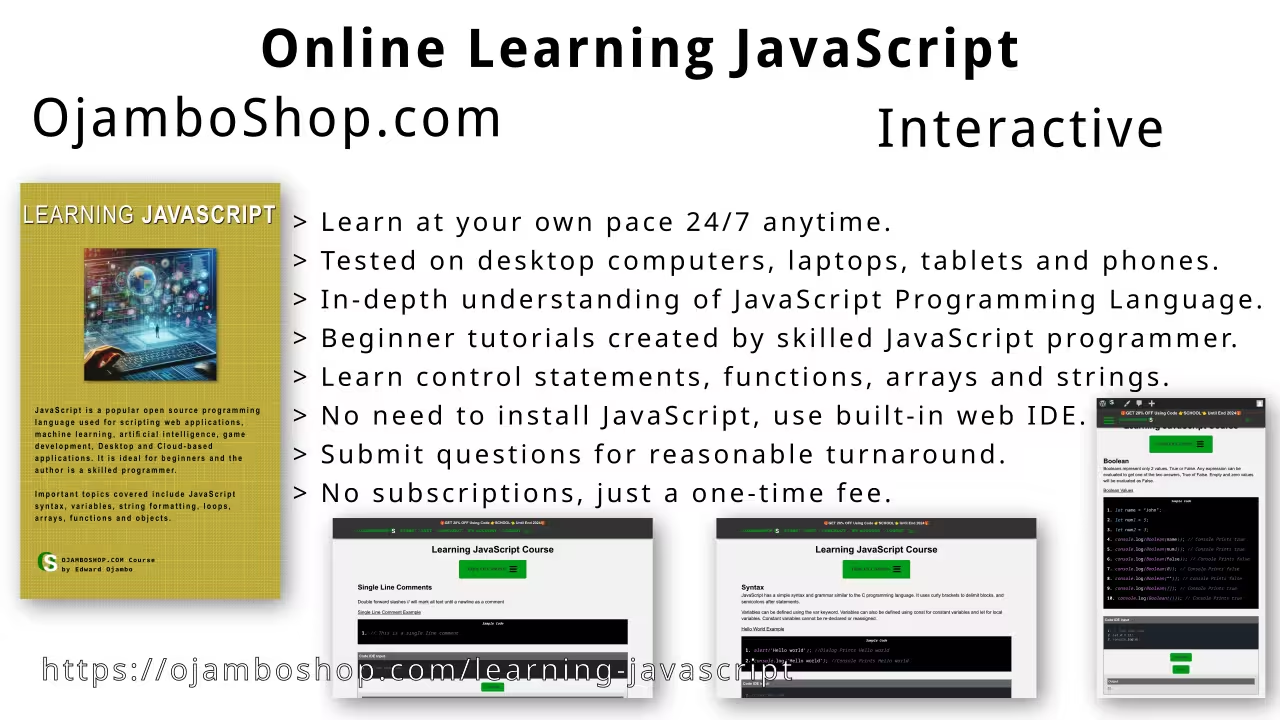
Learn Programming Books:
Learning Javascript Book is available as Learning JavaScript Paperback or Learning JavaScript Ebook.
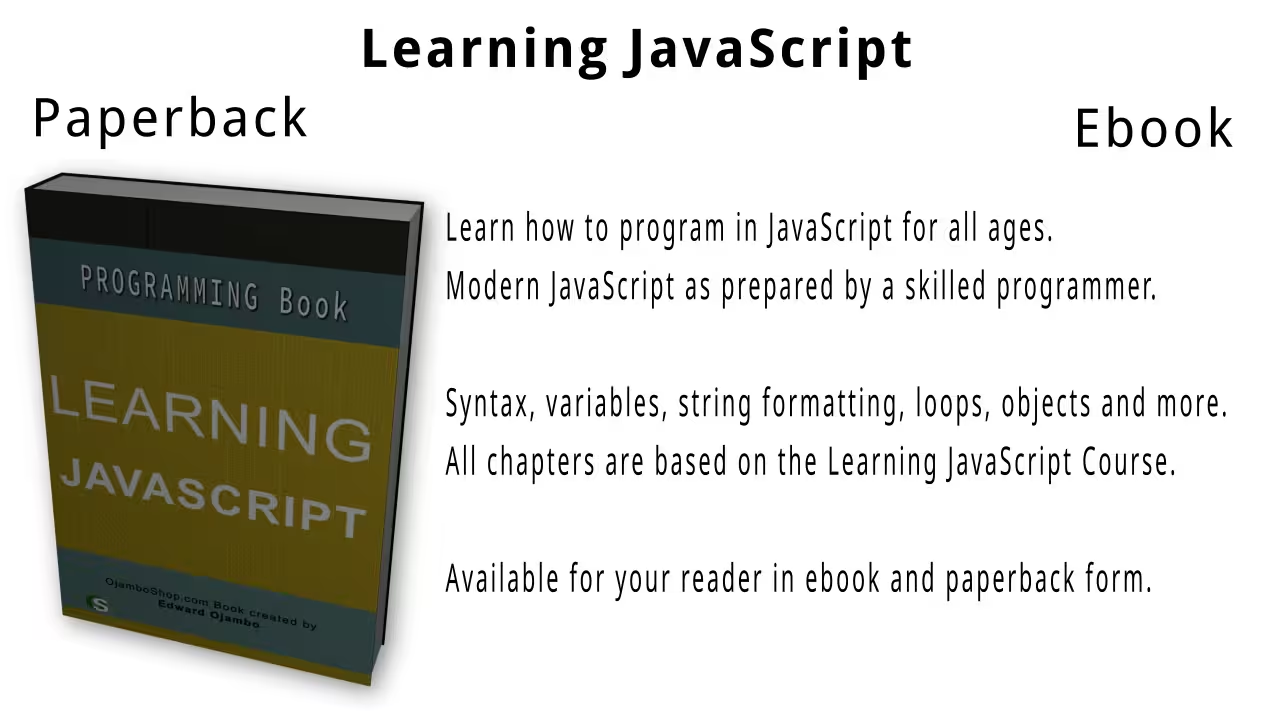
Conclusion:
JavaScript makes it easy to use the ternary operator. Use the ternary operator to write conditional logic in a more compact and readable way.
If you enjoy this article, consider supporting me by purchasing one of my OjamboShop.com Online Programming Courses or publications at Edward Ojambo Programming Books
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Paperback on Amazon
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials