Screen Capture API Download In JavaScript
Screen Capture API can be used to capture part or all of a screen for streaming, recording or sharing.
The getDisplayMedia method is used to capture screen contents as a live MediaStream. The stream can be recorded via MediaDownloader as a blob in chunks. The recorded stream can be downloaded using an anchor tag.
Calling getDisplayMedia returns a promise resolving to a stream containing the live screen contents.
Screen Capture
Glossary:
MediaStream
Represents a stream of media content.
Stream
Consists of several tracks such as video or audio.
Blob
Represents a file-like object of immutable, raw data.
Screen Capture API Methods
Name | Description | Example |
---|---|---|
getDisplayMedia() | Displays screen sharing stream | navigator.mediaDevices.getDisplayMedia(); |
MediaDownloader() | Download media | MediaDownloader(stream); |
Blob([arrayBuffer, typedArray, DataView, string, …], { type: ‘mime-type’ }) | Binary Large Object | Blob(recordedChunks, { type: “video/webm” }); |
download=”filename” | Anchor tag download option | download=”captured-video.webm” |
Name | Description | Example |
CSS Screen Capture API Download Snippet
.hide { display: none; } button, .download { padding: 0.5rem; font-weight: bold; border: 1px solid rgb(0,0,0); color: rgba(0,0,0,1); background-color: buttonface; border-radius: 5px; text-decoration: none; } button:hover, .download:hover { box-shadow: 0 0 10px rgba(0, 0, 0, 0.2); }
HTML Screen Capture API Download Snippet
<button class="start">Record</button> <video class="preview" width="640" height="360" autoplay muted></video> <video class="record hide" width="640" height="360" controls></video> <a class="download hide">Download</a>
JavaScript Screen Capture API Download Snippet
// OjamboShop.com Learning JavaScript Screen Capture API Download Tutorial let start = document.querySelector('.start'); let preview = document.querySelector('.preview'); let record = document.querySelector('.record'); let download = document.querySelector('.download'); start.addEventListener("click", function(evt) { let text = evt.target.textContent; if (text == "Record") { evt.target.textContent = "Stop"; navigator.mediaDevices.getDisplayMedia({ video: true, audio: false }). then(stream => { preview.srcObject = stream; let recorder = new MediaRecorder(stream); let chunks = []; recorder.ondataavailable = event => chunks.push(event.data); recorder.onstop = event => { let blob = new Blob(chunks, { type: chunks[0].type }); let [type, subtype] = chunks[0].type.split('/'); record.src = URL.createObjectURL(blob); record.style.display = "inline-block"; download.href = record.src; download.download = `captured-video.${subtype}`; download.style.display = "inline-block"; }; recorder.start(); }); } else { evt.target.textContent = "Record"; preview.srcObject.getTracks().forEach(track => track.stop()); } });
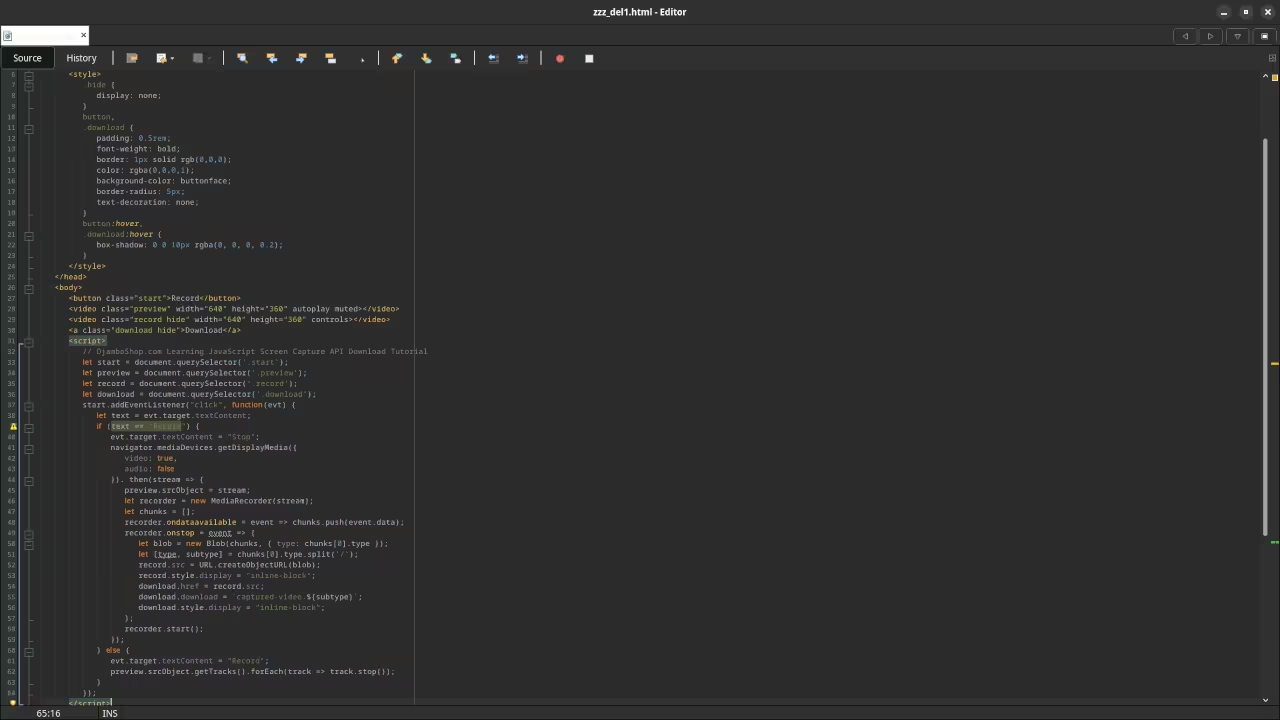
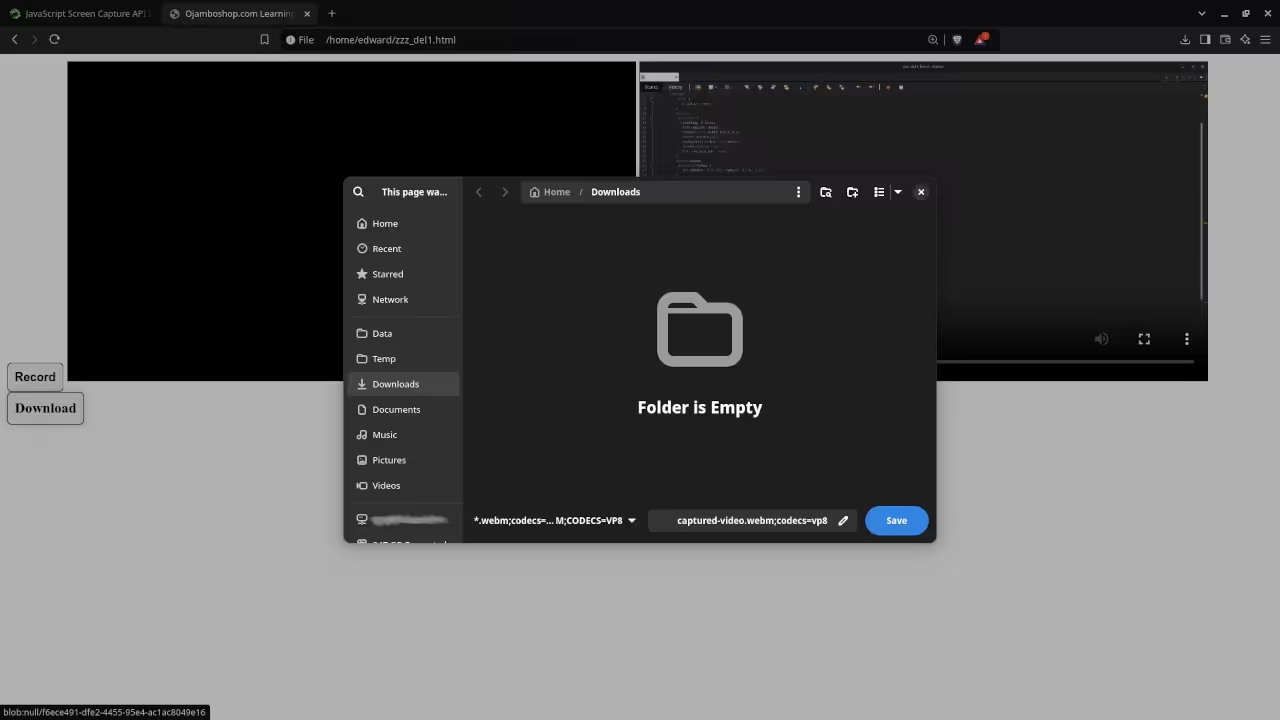
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE was used to input and compile JavaScript code for Screen Capture API record.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
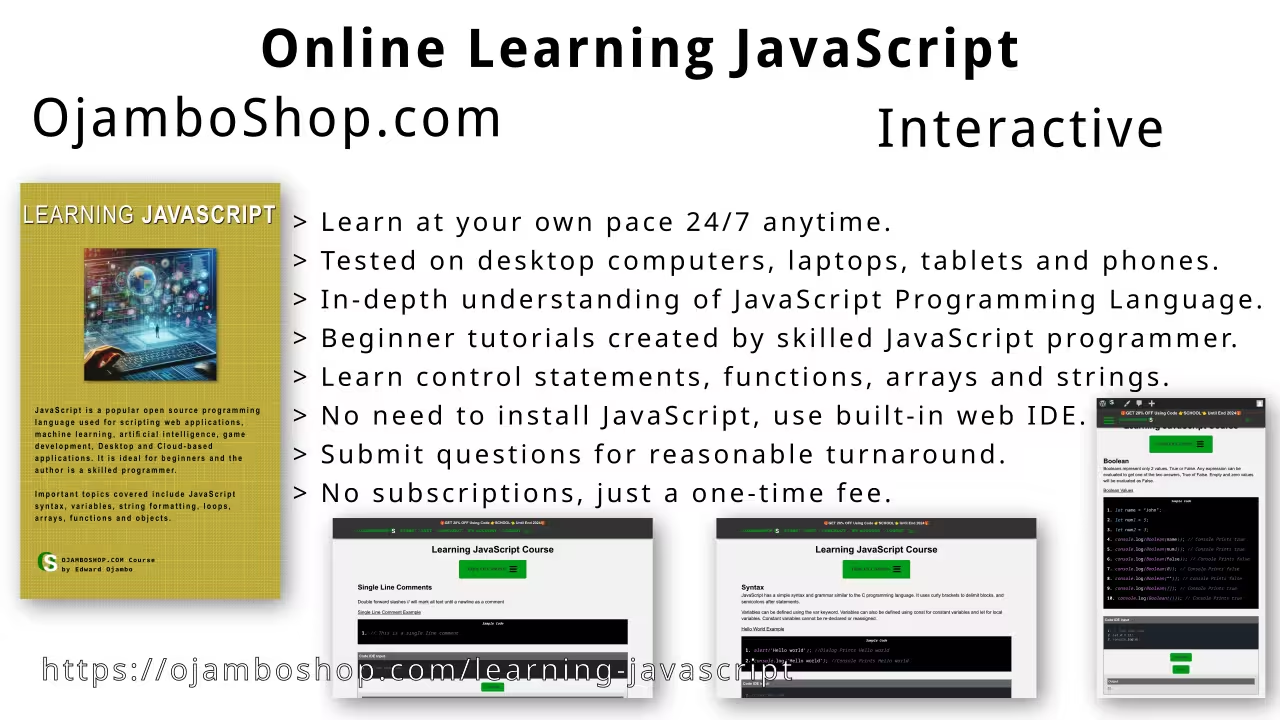
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning JavaScript Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
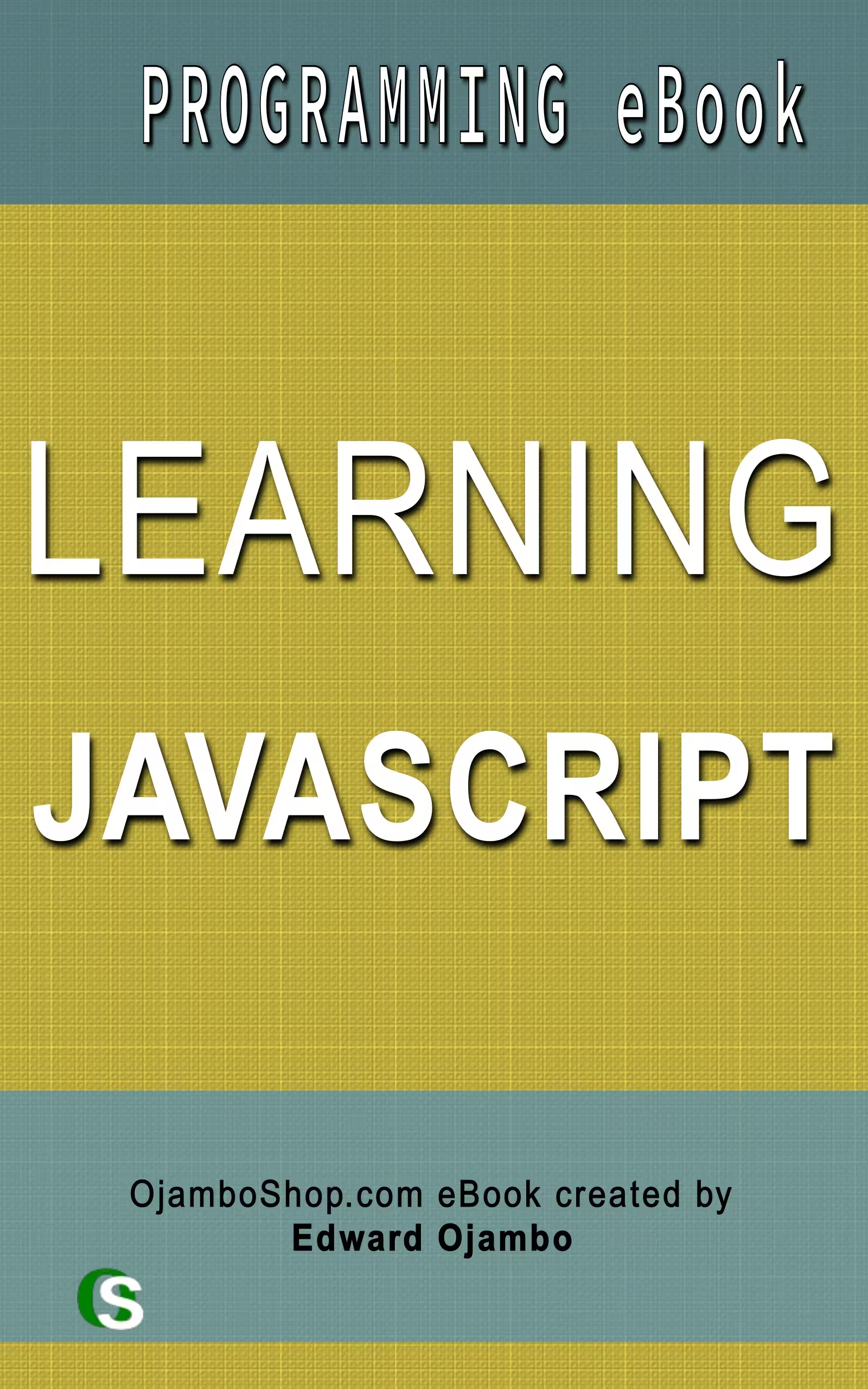
Conclusion:
JavaScript makes it easy to use Screen Capture API. Use the built-in getDisplayMedia method to display a screen capture, MediaDownloader to record the stream and the anchor tag to download.
Take this opportunity to learn the JavaScript programming language by making a one-time purchase at Learning JavaScript Course. A web browser is the only thing needed to learn JavaScript in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning JavaScript Ebook
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials