Read JSON Files In JavaScript
JavaScript Object Notation or JSON is an open data interchange language standard.
JSON is a text format that is programming language-independent. JSON is a collection of key/value pairs.
The JSON object begins with a left curly brace and ends with a right curly brace. Each key/value pair is separated by a colon.
JSON Object
Glossary:
Open Standard
Accessible and available to anyone.
Electronic Data Interchange
Independent of communication and software technology.
Human-readable
Encoding of data that is easily read.
Name-value Pair
Data model in key/value pairs.
Array
Data type representing a collection of values.
JSON Object Collection
Name | Description | Example |
---|---|---|
Array | Ordered arrangement of values | “names”: [“Jane”, “Jake”, “John”] |
String | Sequence of alphanumeric text or symbols | “name”: “John” |
Number | Integers and floating point numeric types | “age”: 24 |
Whitespace | Represents horizontal or vertical space | “linefeed”: “\n” |
Boolean | True or false | “completed”: true |
Null | Represents non-existent of invalid object | “extra”: null |
Name | Description | Example |
JSON File
{ 111: {"username":"xyz33434434", "joined":"2024-11-15", "messages":0, "likes":0, "following":0, "followers":100000}, 11: {"username":"johnd", "joined":"2024-11-10", "messages":2, "likes":1, "following":10, "followers":0}, 1: {"username":"janeb", "joined":"2023-10-10", "messages":200, "likes":10, "following":20, "followers":10} }
JavaScript JSON File Reader Snippet
// OjamboShop.com Learning JavaScript JSON File Reader Tutorial fetch('accounts.json') .then(response => response.json()) .then(data => { let target = document.querySelector('.target'); for (let key in data.accounts) { let paragraph = document.createElement('p'); paragraph.innerHTML += `username: ${data.accounts[key].username}`; paragraph.innerHTML += `, joined: ${data.accounts[key].joined}`; paragraph.innerHTML += `, messages: ${data.accounts[key].messages}`; paragraph.innerHTML += `, likes: ${data.accounts[key].likes}`; paragraph.innerHTML += `, following: ${data.accounts[key].following}`; paragraph.innerHTML += `, followers: ${data.accounts[key].followers}`; target.appendChild(paragraph); } });
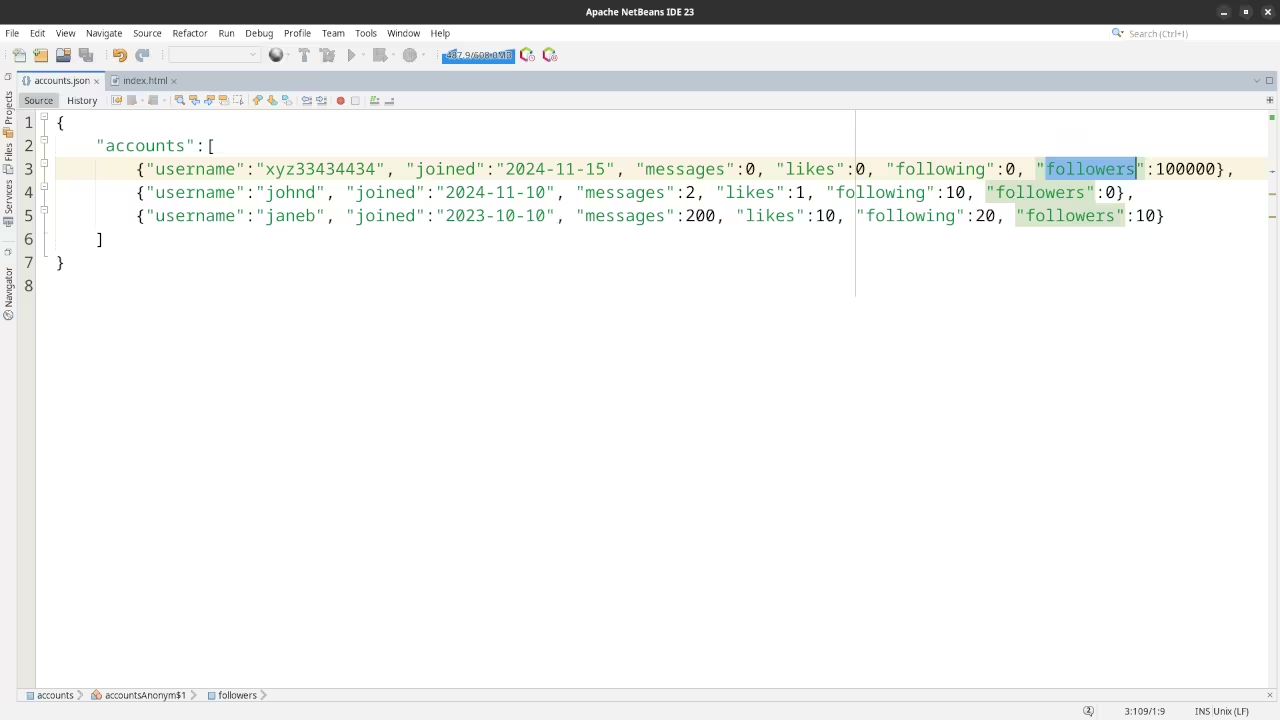
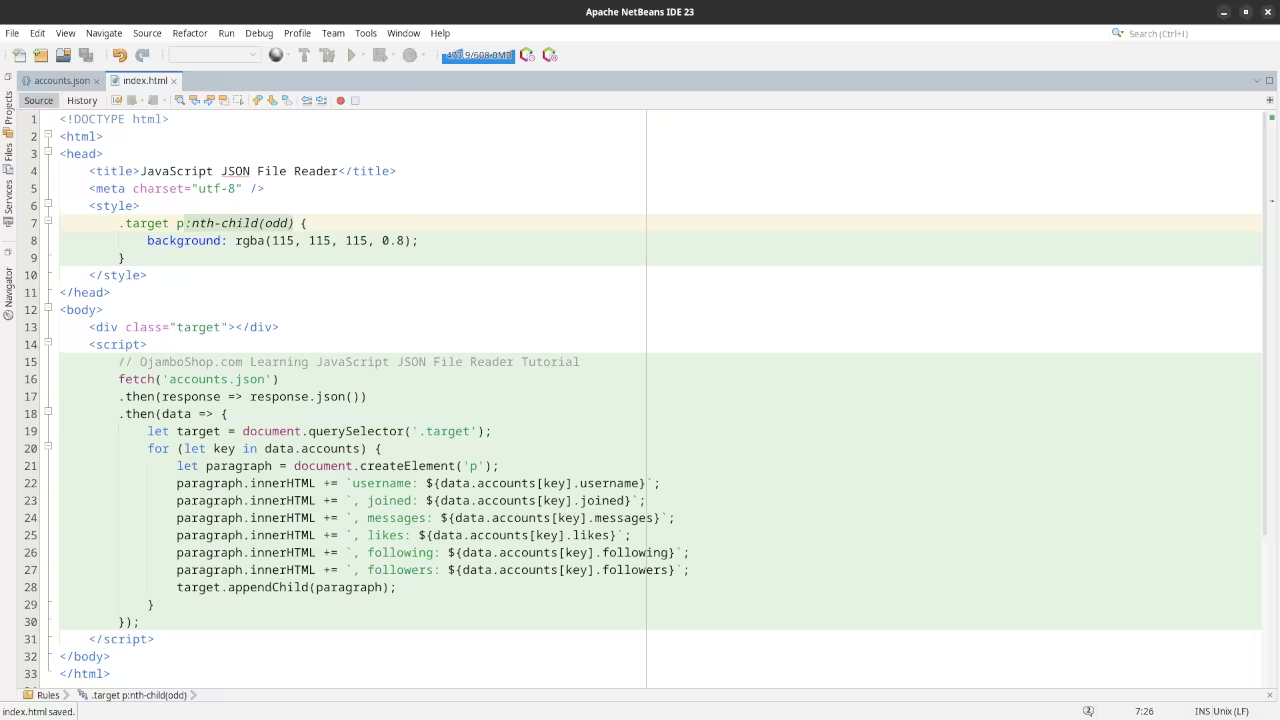
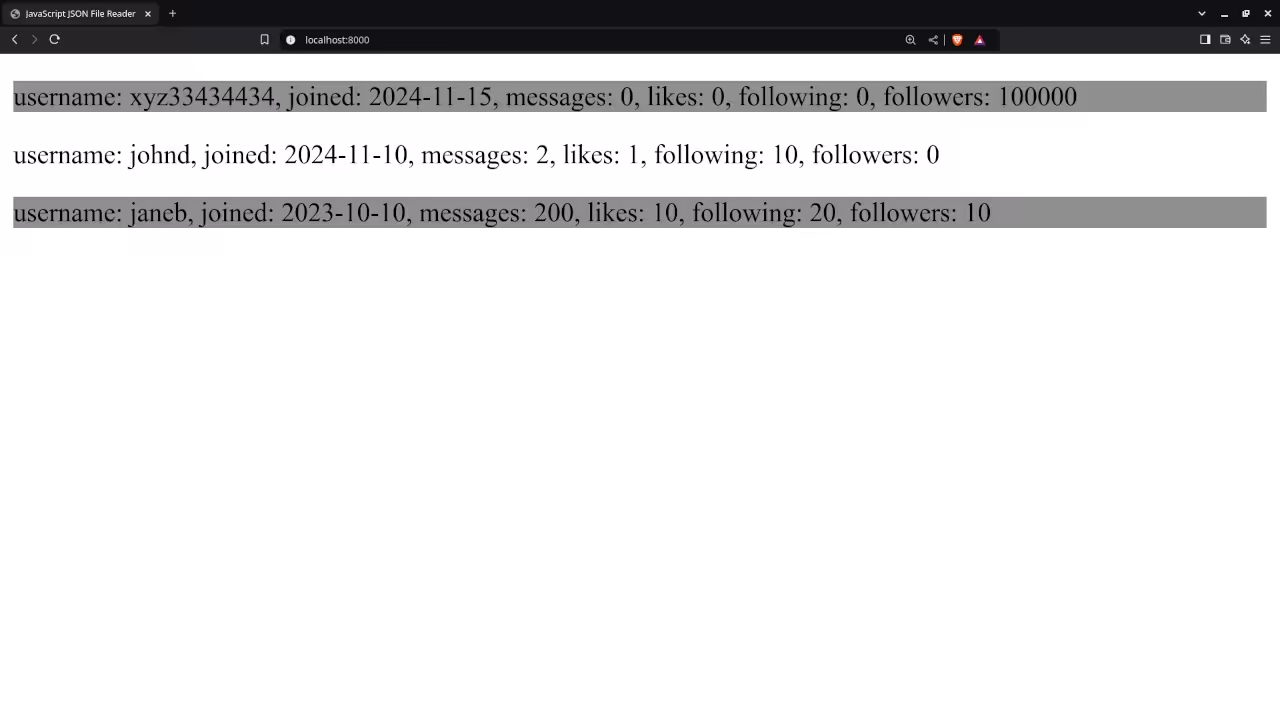
Usage
You can use any IDE or text editor and the web browser to compile and execute JavaScript code. For this tutorial, the OjamboShop.com Learning JavaScript Course Web IDE was used to input and compile JavaScript code for the JSON file reader.
Open Source
JavaScript follows the ECMAScript standard and is licensed under the W3C Software License by web browser vendors and runtime environment vendors. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
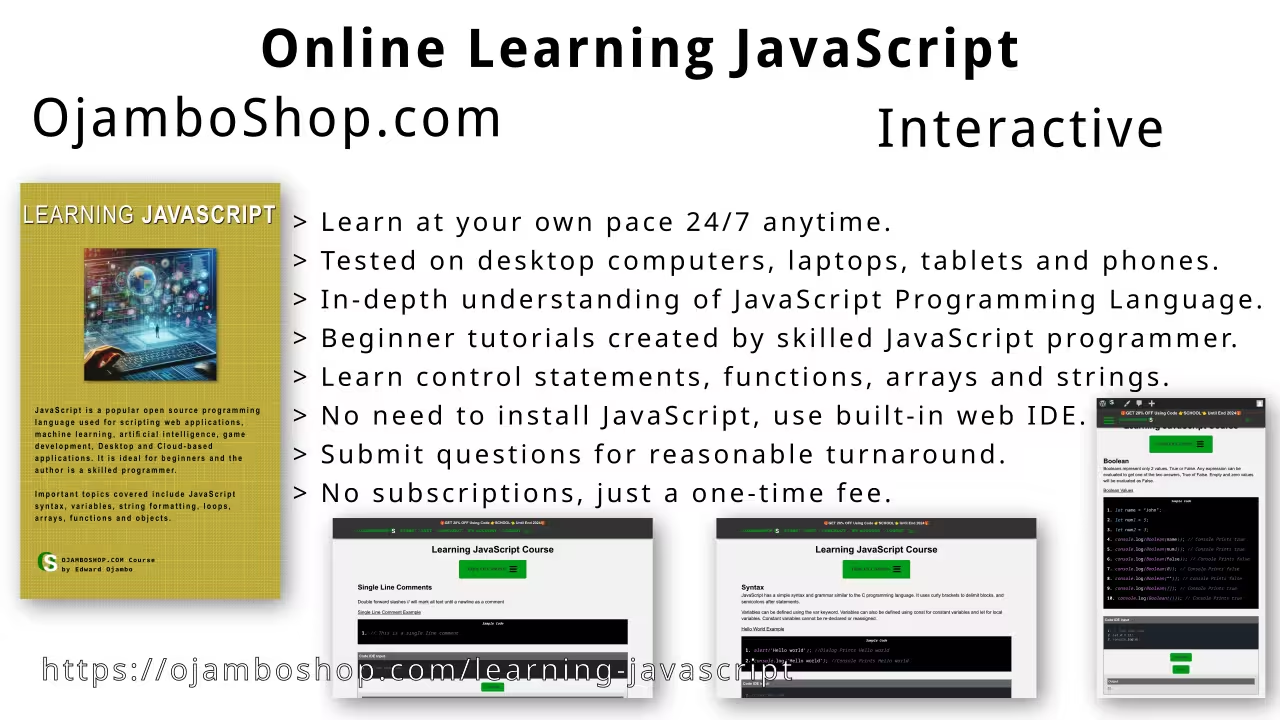
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning JavaScript Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
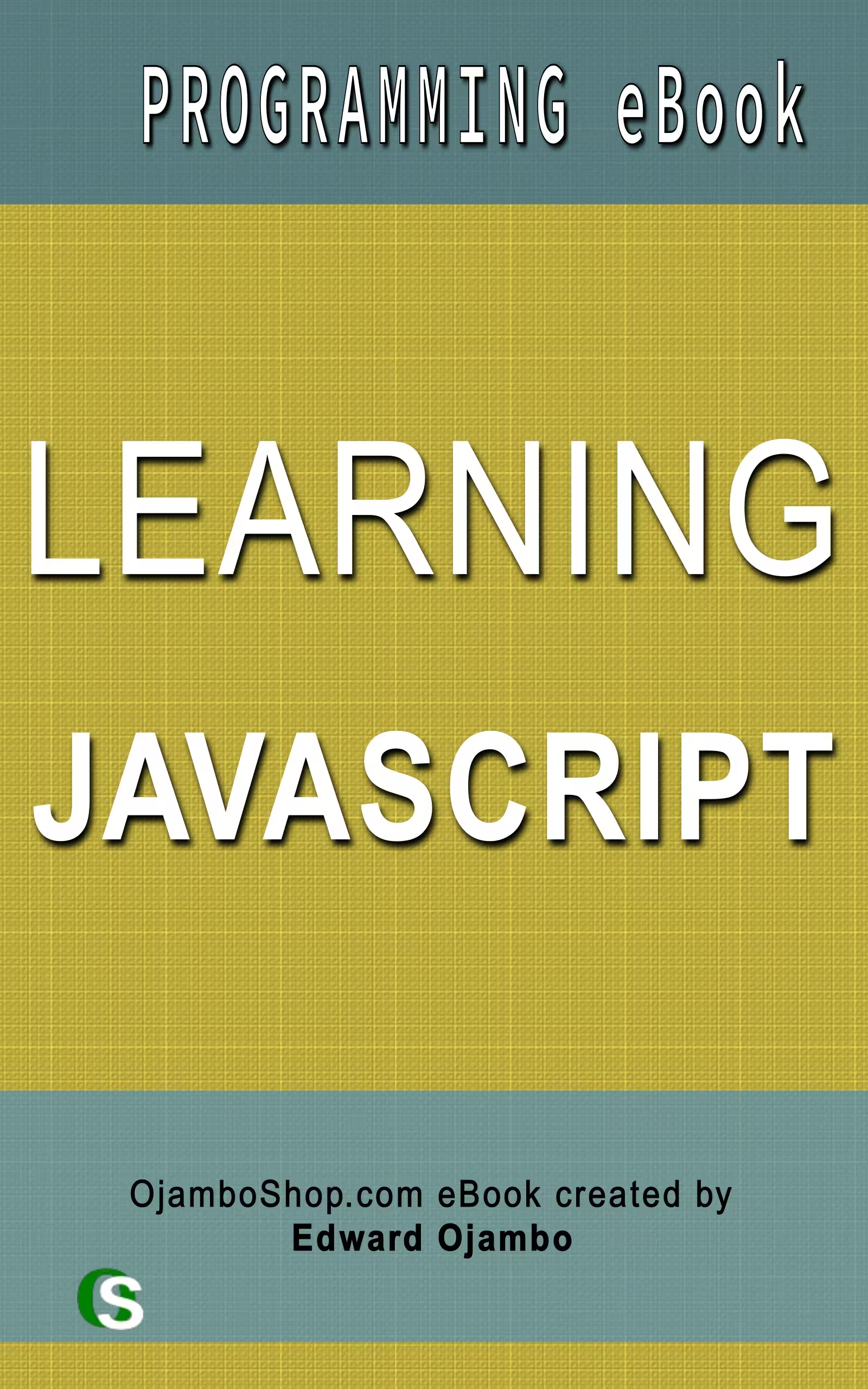
Conclusion:
JavaScript makes it easy to generate a JSON file reader. Use a loop to iterate data for the JSON file reader.
Take this opportunity to learn the JavaScript programming language by making a one-time purchase at Learning JavaScript Course. A web browser is the only thing needed to learn JavaScript in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning JavaScript Ebook
References:
- Learning JavaScript Course on OjamboShop.com
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning JavaScript Ebook on Amazon
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon
- OjamboServices.com For Custom Websites, Applications & Tutorials