MySQL Connection In Rust
The Rust MySQL Connector is required for Rust to connect to the database.
A separate MariaDB Rust Connector is available and can be installed.
The MySQL dependency must be included first. This tutorial uses MariaDB 10.11.8 and GCC 14.2.1 on Fedora Linux 40.
MySQL Interface
Name | Description | Example |
---|---|---|
get_conn | Create database connection | get_conn() |
query | Perform database query | query(query) |
Name | Description | Example |
Install MySQL Driver Via Cargo.toml
[dependencies] mysql = "*"
Rust MySQL Select Data
use mysql::*; use mysql::prelude::*; fn main() -> std::result::Result<(), Box<dyn std::error::Error>> { let url = "mysql://dbuser:dbpass@localhost:3306/dbname"; let pool = Pool::new(url)?; let mut conn = pool.get_conn()?; let result:Vec<(i32, String, i32)> = conn.query("select sid, name, age from Students")?; for r in result { println!("{}, {}, {:?}", r.0, r.1, r.2); } Ok(()) }
SQL Injection Security Warning
Variable input must be sanitized, validated, properly formatted and escaped. For this tutorial, the variable input is known and trusted and security is not concerning.
Rust MySQL Select Data Escape String
use mysql::*; use mysql::prelude::*; fn main() -> std::result::Result<(), Box<dyn std::error::Error>> { let url = "mysql://tutorial:tutpass@localhost:3306/tutorial"; let pool = Pool::new(url)?; let mut conn = pool.get_conn()?; let query_string = format!("SELECT sid, name, age from Students WHERE name = \"{}\"", format!("D'Arcy")); let result:Vec<(i32, String, i32)> = conn.query(query_string)?; for r in result { println!("{}, {}, {:?}", r.0, r.1, r.2); } Ok(()) }
Compile And Execute
cargo run
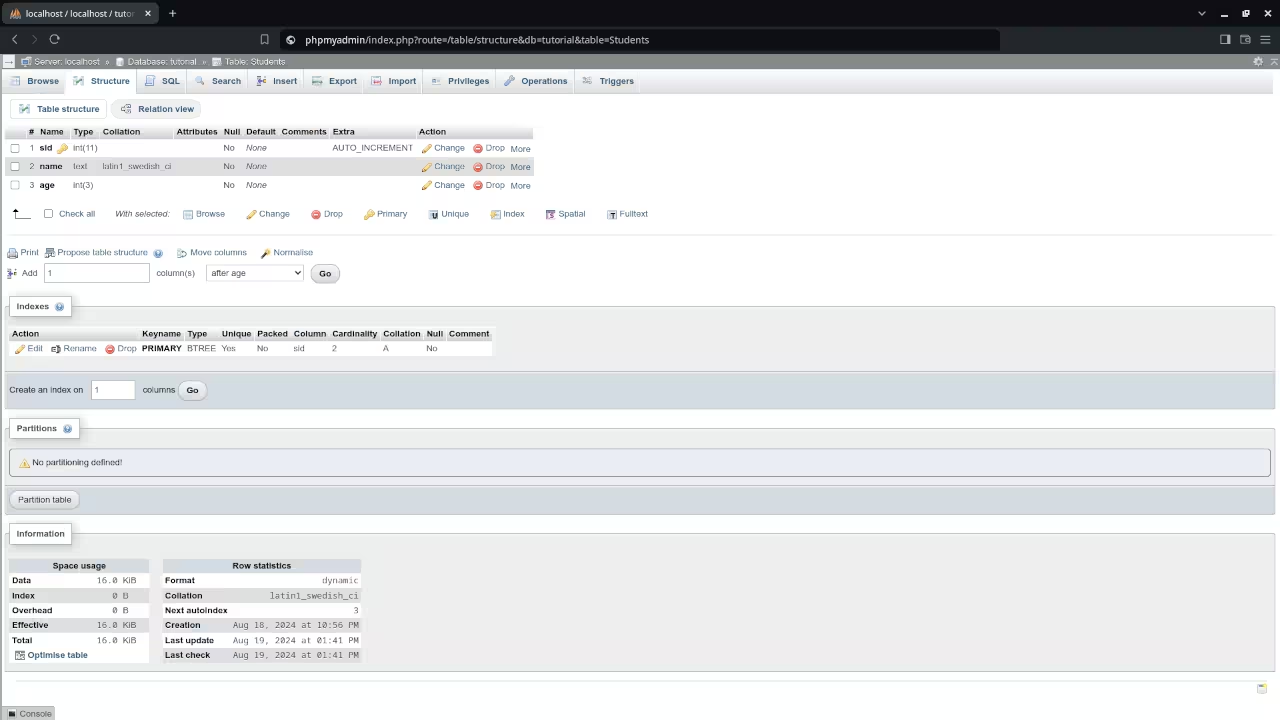
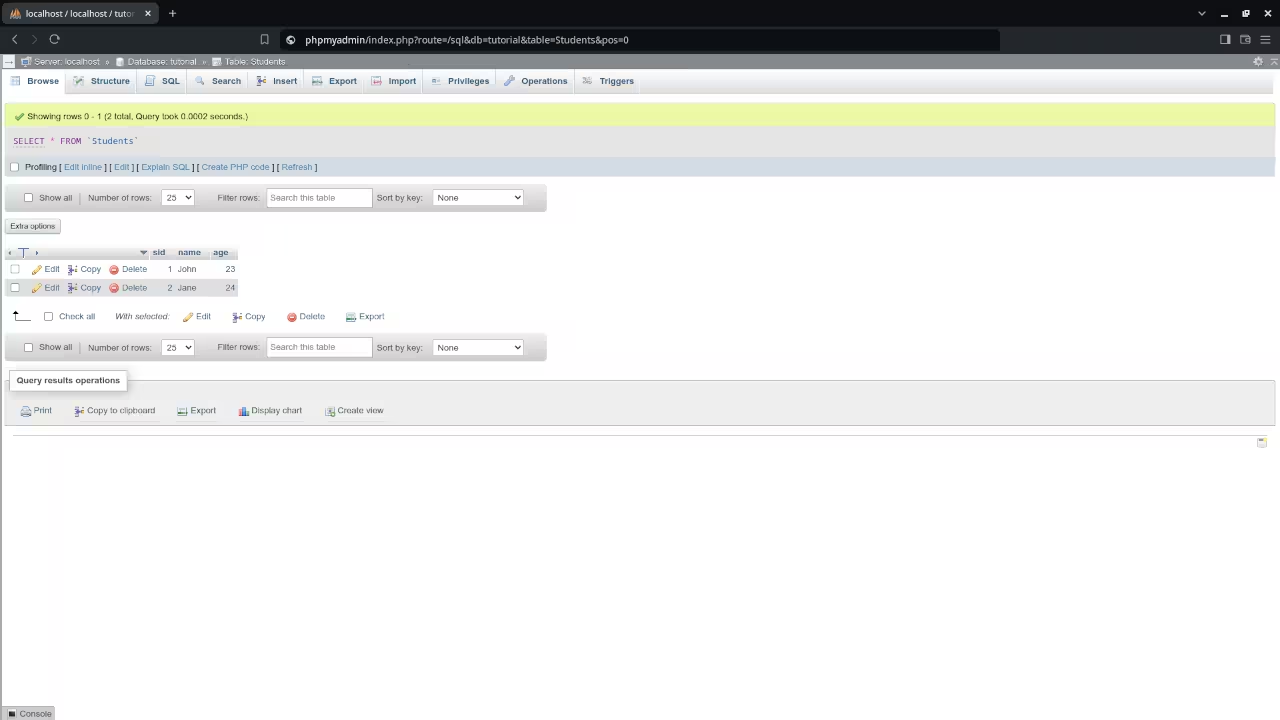
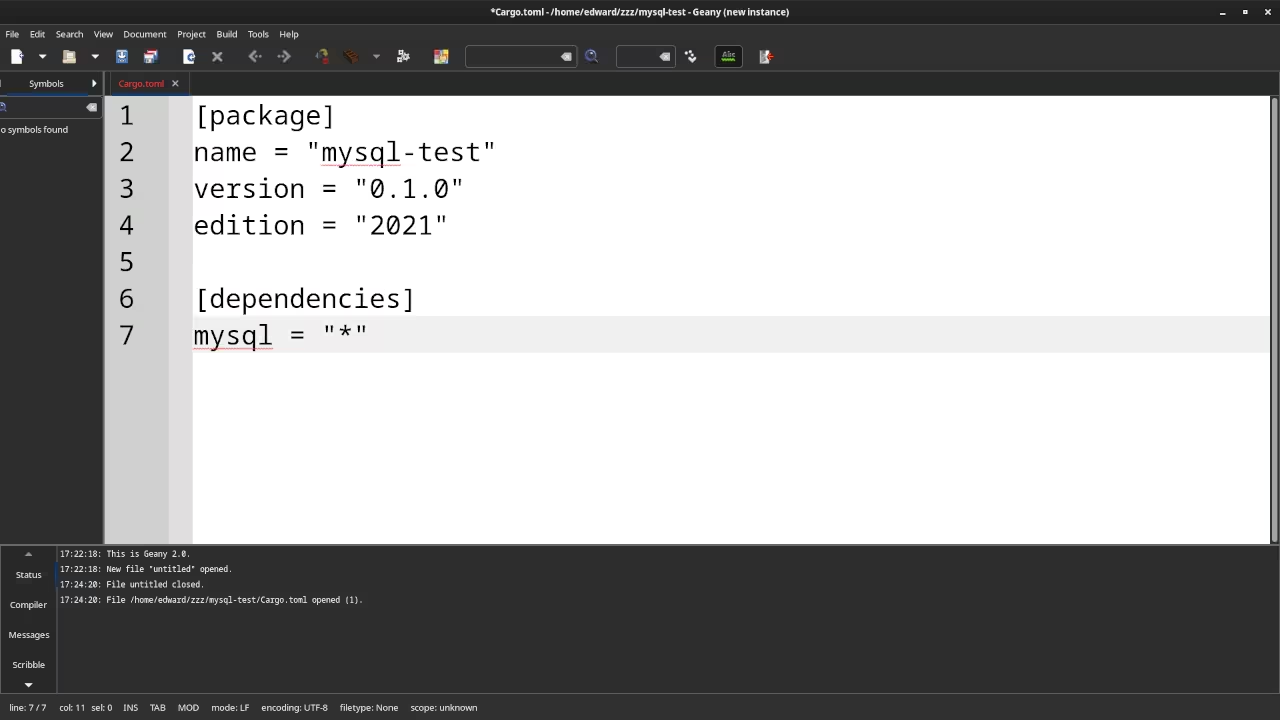
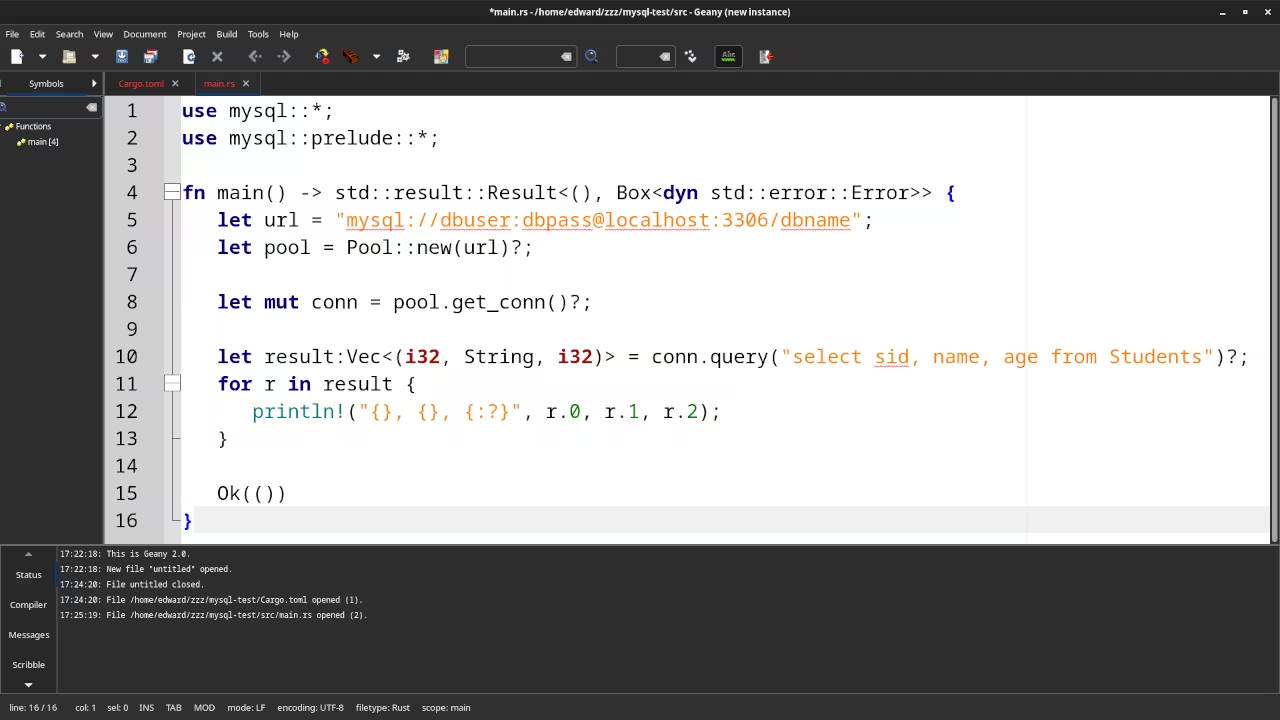
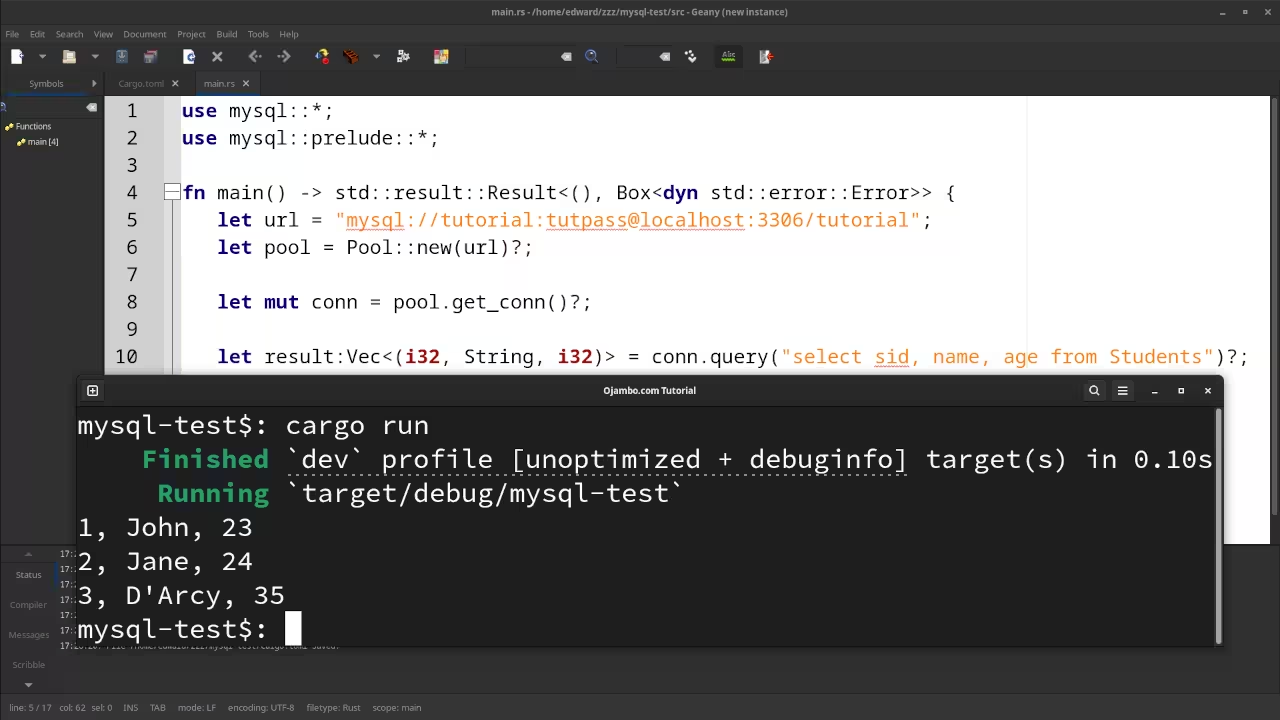
Usage
You can use any IDE or text editor and the command line to compile and execute Rust code. In this tutorial, the OjamboShop.com Learning Python Course and Learning PHP completion inspired the database connection.
Open Source
Rust is dual licensed under the Apache License, Version 2.0 and MIT license. This allows commercial use, modification, distribution, and allows making derivatives proprietary.
Learn Programming Courses:
Courses are optimized for your web browser on any device.
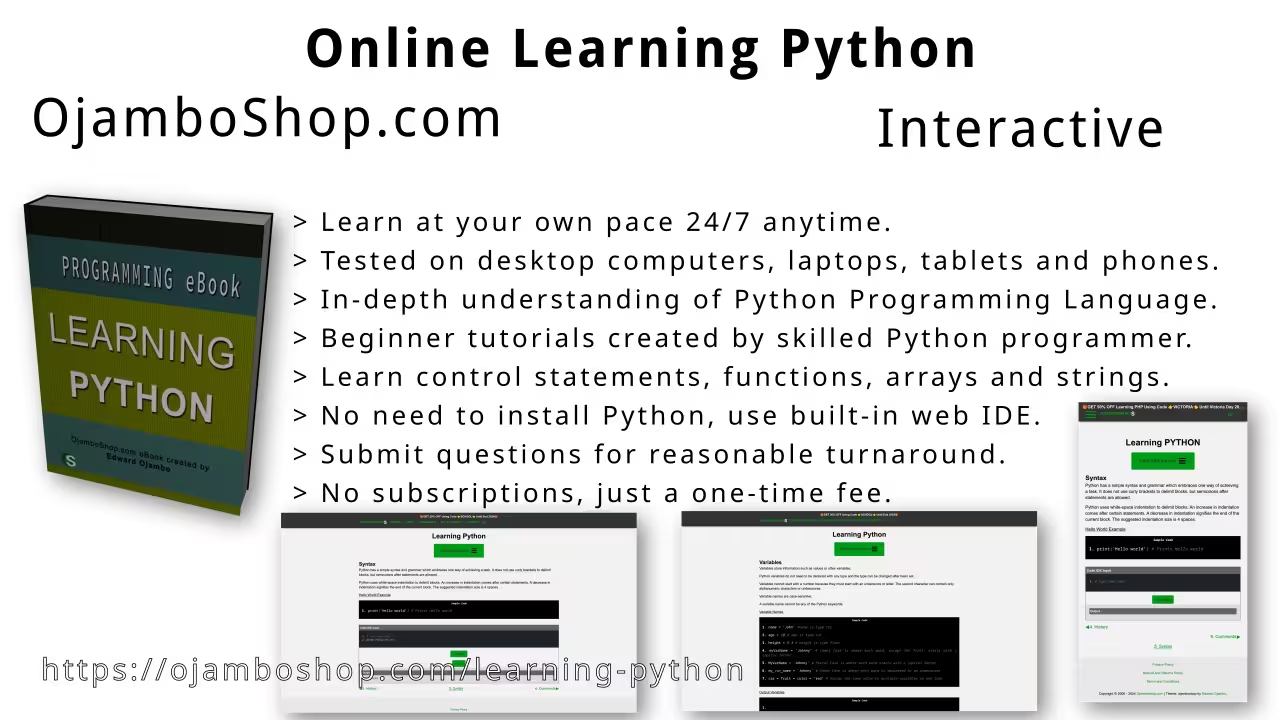
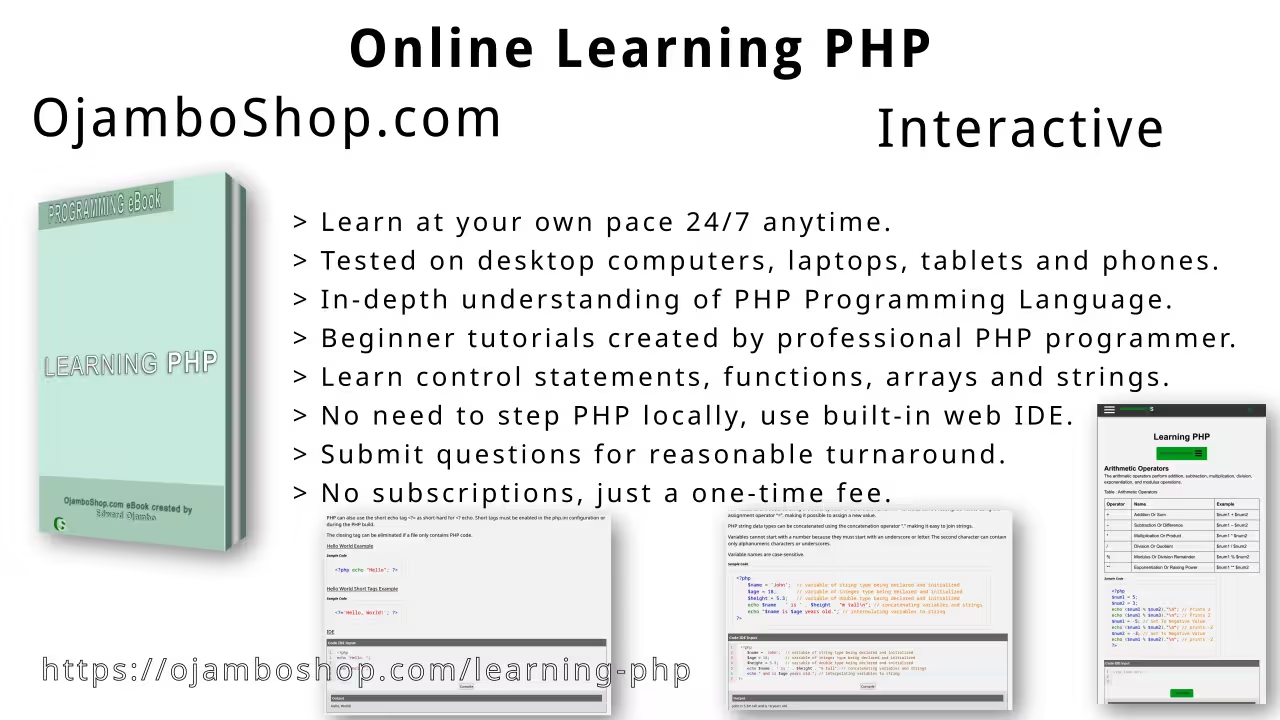
Limited Time Offer:
OjamboShop.com is offering 20% off coupon code SCHOOL for Learning Python Course or for Learning PHP Course until End Day 2024.
Learn Programming Ebooks:
Ebooks can be downloaded to your reader of choice.
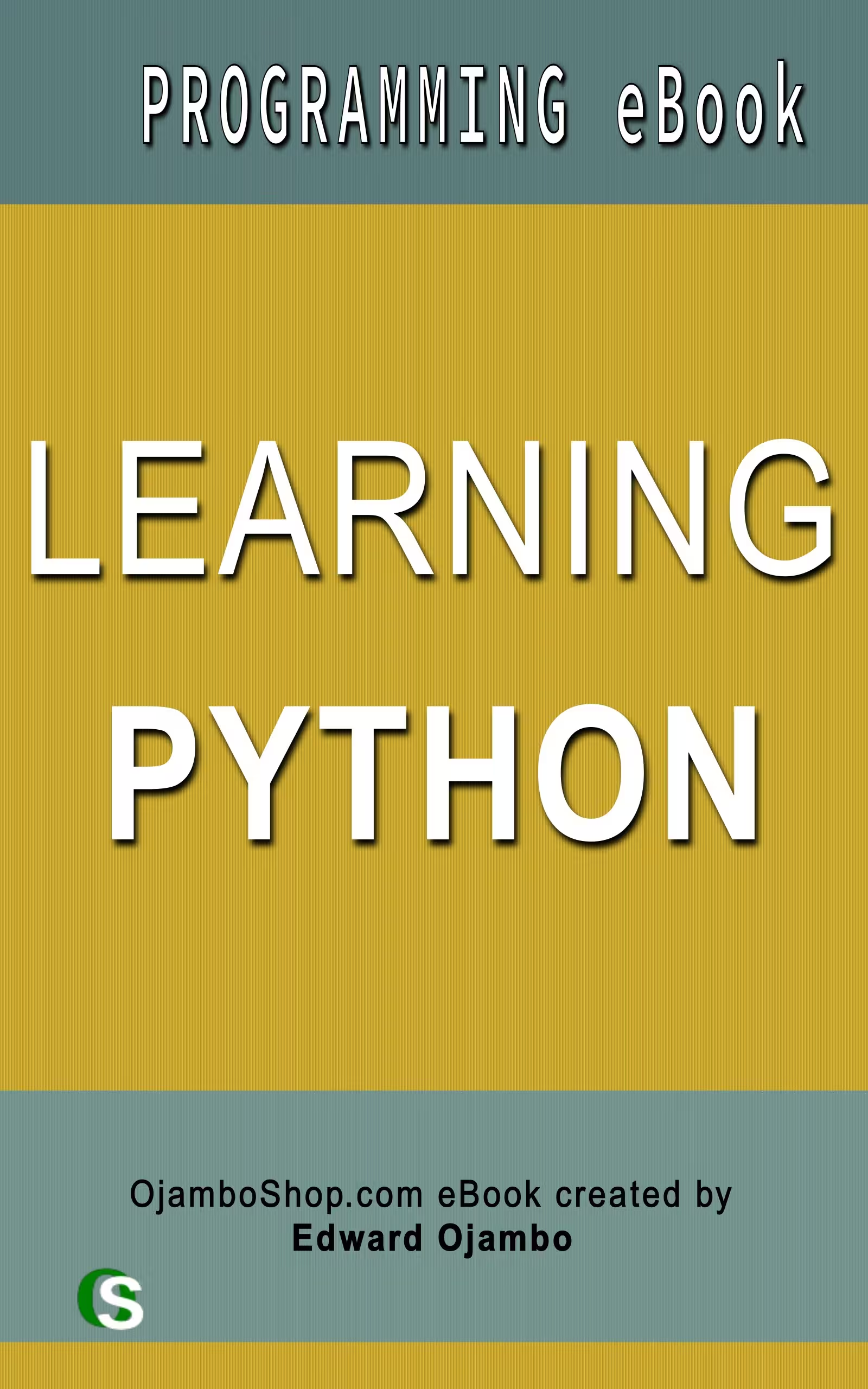
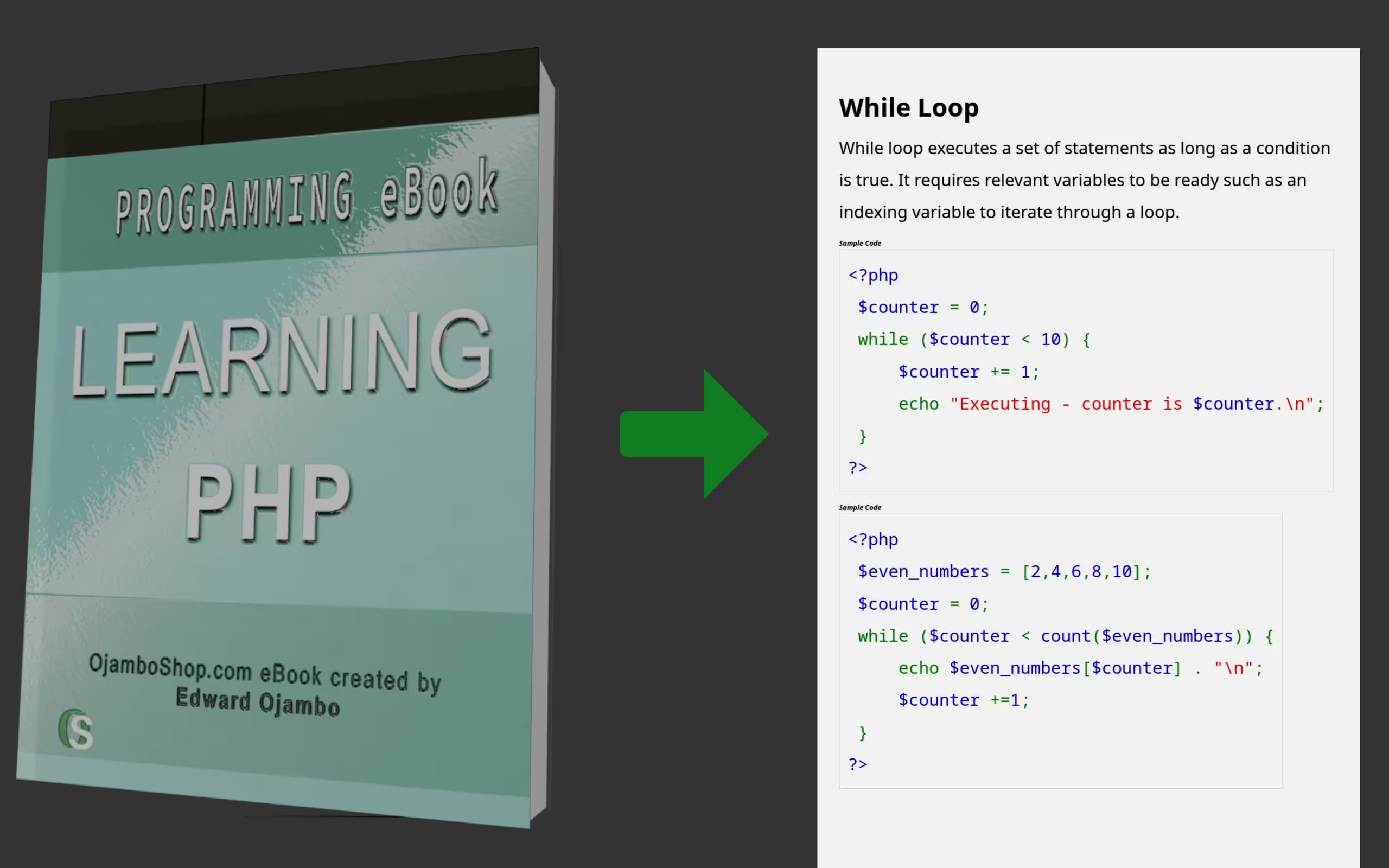
Conclusion:
Cargo makes it easy for Rust to perform database connections using the MySQL Connector. The MySQL dependency must be included in the Cargo.toml file to perform MySQL functions including the procedure to select database data.
Take this opportunity to learn the Python or PHP programming language by making a one-time purchase at Learning Python Course or Learning PHP Course. A web browser is the only thing needed to learn Python or PHP in 2024 at your leisure. All the developer tools are provided right in your web browser.
If you prefer to download ebook versions for your reader then you may purchase at Learning Python Ebook or Learning PHP Ebook
References:
- Learning Python Course on OjamboShop.com
- Learning PHP Course on OjamboShop.com
- Learning Python Ebook on Amazon
- Learning PHP Ebook on Amazon